Passing Props and Toggling an Element in React.js
**Aurooba Ahmed:** You are listening to viewSource a conversation around tech, web development, and WordPress with hosts Aurooba Ahmed That's me and Brian Coords
**Brian Coords:** Aurooba, we are back to our React series where we are building an accordion in React from the very beginning. In piece by piece. Line by line. Brick by brick, and we've made it Only I, this is I think our sixth episode in the series, but we've been consistently getting one piece of feedback. Can you share what is this feedback about our React accordion element we've been building that we keep hearing?
**Aurooba Ahmed:** Yeah, and rightly so, by the way, what happens is the way it's currently set up right now, It is only when you click on the accordion header that the content for the accordion panel gets injected into the page, into the dom. So if you have the accordion in the not open state, the content of that panel is actually not on the page.
**Brian Coords:** Yeah, and I think we've talked about this. As we've been building it, that we're using React and we're injecting stuff and removing it and injecting it and removing it. So it's something we've been aware of and we've talked about when we get to the phase of turning this into a Gutenberg block, which is like another part of this conversation, we will deal with that.
And it's been asked for, we've talked about it and the day has come. We have dealt with it. We've. Today is all about taking our accordion and instead of injecting and hiding and removing content from the dom, we're gonna load it all up in the dom and we're gonna show and hide it with good old CSS, , mixed into our, into our React application.
I'm excited.
**Aurooba Ahmed:** Yeah, me too. I think. The really great thing about something like react is, ultimately, once it all comes into the browser, it's just html, CSS, and JavaScript. And in the world of the web, those things are always very closely tied to each other. So I'm excited for us to sort of show that on the React side too.
Where yes, react can do is lots of funky things like inject and remove content, but sometimes. Especially like in this case, that's not necessarily the best use, and instead we should just use CSS for it, right?
**Brian Coords:** Yeah, and I think we talked about SEO, like people are very concerned about. Especially with the, the world of single page apps, like I need that content to be there so that it can be crawled, so that it can be scanned if you're constantly hiding and removing things, that might be great in like more of an application sense, but it's not very good in like a like content sense, which is where we're heading is towards like a WordPress page or something like that.
So there's that piece of it. And then there's the other piece, which is like, once we do move this into WordPress, You know, we kind of need everything. Like once we make an editing experience, , we don't, can't just like remove stuff from people. It has to be editable and visible and yeah. So
**Aurooba Ahmed:** exactly. And like how we do that is a, yeah. It's a different conversation. But before we get there, let's, uh, let's look at some code. Yeah.
**Brian Coords:** Yeah, let's do it.
## Previous code recap
**Aurooba Ahmed:** so right now I'm sharing the front end of the website, and it's that same page. Where we're just building an accordion, and you can see where we were last time, where we have three "hello world" components, sorry, accordion items. When you open it, it shows you the information. If you open another one, the one that was already open, it closes up.
So behaviorally if you're not looking at the code, it all actually still looks exactly the same as where we left it off.
So the difference is actually when you come into the dev tools, which I have here, open on the right, and here we have our heading the very first one, which is Hello World. But underneath it, before you would've just seen the next heading and the next heading. But now in between the headings, you're actually seeing the panel, uh, that has all of the content in it.
And all we're doing is we're making it visible instead of actually just injecting it whenever we click on it.
**Brian Coords:** Can I make one comment about the markup here, which is that. Sometimes in accordions, if you've used like a bootstrap or something, you get like a div and a div and a div and a div. it gets so divvy and you like what we have here is like heading and then one div just for like everything. That's the content.
And if we were only allowing one paragraph, we could even just have that. But in this case, you know, potentially we might want more, you know, rich content inside there, but it's, there's no wrapper around each item. It's, it's like so semantically clean, , that I really love that about it. I, I just love how, like, how limited, you know, it's not another div around it and we're toggling the class here and we're, you know, it's, it's just, it's, it's a very nice clean, uh, chunk of html.
**Aurooba Ahmed:** Definitely, and you know, those divs and everything. I think that once we go into the block perspective, it may not be quite that clean, but we can still make it very clean, you know, comparatively.
**Brian Coords:** , I think that's one of the big. goals of Gutenberg in like when I was saying it's really like divvy, I didn't mean like, like a Divi builder. I meant like it had a lot of divs, but then like I thought in my head like it is like a Divi, like those page builders, like sometimes man, they get like row, column, column, inner row in like, it's like so many elements and you know, a really, really good Gutenberg block will just be
the thing that you need. And it will be nothing more, nothing less, not a bunch of divs. Um, it's generally pretty good. So I think if we can achieve that, that would be, I would love to see us get to that place with this.
**Aurooba Ahmed:** for sure. But I think like that will be something we do a little bit later in the second large part of this series. Right? Yeah. Where we actually take all of this and turn it into a block.
**Brian Coords:** Yeah. Which we have thoughts on.
**Aurooba Ahmed:** Yes. We'll get to that in a little bit, but let's take a look at how we took our existing accordion and made it so that the content is always there, and then used a little bit of CSS using the same logic as before to, you know, show and hide the content.
**Brian Coords:** at the end of the day there's state and state is telling us what's expanded, what's not expanded. We're still using that same state that we learned about in the previous episode. We're just going to take that state and instead, instead of removing a component, we're just gonna like toggle some attributes on it.
## Adding a key for looping elements
**Aurooba Ahmed:** Yeah, so here we are looking at the app js file, and I'm just in here to show that literally nothing has changed. Here we have, we added a little key because React was yelling at us,
**Brian Coords:** yeah.
**Aurooba Ahmed:** but aside from that, the actual main component is exactly the same. We're still passing the same Props to the accordion item.
So then when we go into the accordion item file, this is also almost the same. Instead, what we're doing here is we're taking that is expanded and instead of using that to inject the accordion panel, what if the is expanded is true. We're actually passing that as a prop to the accordion panel item because we wanna use it inside it instead Now,
**Brian Coords:** Yeah, so before we were literally checking as expanded and if it was false, just not even loading the panel Now, Of the com, the panel's always there and we're letting the panel know if it's as
## Components are not HTML elements
**Brian Coords:** expanded. But when we were speed running, writing this little change for this episode, the first thing you did was put a class.
Do you remember? Like we were just like not thinking, like doing it really fast and you used. The className attribute and like just tried to set a class on the accordion panel and then like we were like staring at it and we're like, why won't the class show up? And then we're like, oh yeah, cuz it's like this is a component and it's, it's not a, like you think, you start thinking this is just another HTML element and I can just stick things on it.
But it's not, it's a component and it's, if you put something on there, you better in your component deal with that attribute and do something about it. So instead we're just passing isExpanded to it. And then, and then we'll see the logic from that.
**Aurooba Ahmed:** Exactly. I wanna talk about that just a little bit more. Technically, if we had set up our accordion panel component to be able to have a className, we could put the className here, and then that would get passed down as a prop to the final HTML element. And lots of blocks and lots of elements
that you might see in the wild world of React, including inside Gutenberg do that they have support className and ID so that you can pass it down to the final HTML element. But in this particular case, you know, we, that's not how we set it up. So obviously that wasn't gonna work cuz you hacked to explicitly give it that support, that logic.
So instead, yeah, we've passed that prop. So then let's go and take a look at the accordion panel. So now I'm in the accordion panel file and in the top we are expecting the isExpanded prop now and here what we did was we add a className that has a little bit of logic in it.
**Brian Coords:** We're checking is expanded. Our nice turn operator, if it's true, the className will be, is expanded. If it's not true, the className will just be an empty string. Um, and apart from the.
## className
**Brian Coords:** Well, I think the first thing that some people do get tripped up on, which I don't think we've dealt with yet, is className versus like class because we're on a div.
Why can't I just put a class, you know, instead I'm putting a className. But, um, you know, that's just one of those react things that you like, you don't even realize it's a thing after like, you know, five minutes. Like you get so used to using className instead of class, um, that you get pretty used to it.
But I know for new people it's a little weird at first.
**Aurooba Ahmed:** Yeah, like it is classes a reserved word in React because you can create classes like just how you can make them in php. So you can't use that for the Div class or like an HTML element class. And you know, something that I find really helpful is to have a. Like an intelligence plugin or something like that.
In, for example, it's already built into Visual Studio Code, where a lot of the times it will detect it for you and it will correct it for you. So that's always really helpful to also get over that initial like, oh, the className, why is it className and not class, but, and there are a few different elements like that in a lot of situations.
For example, like. Normally Aria labeled by would be set up in Camel case, but because it's, it is what it is, we can just pass it the regular way that it's set up in HTML and it'll just work. But you'll notice in a lot of React components, sometimes people will expect you to pass them in Camel case form, which is more react friendly instead of in the, what's, what's this called?
Snake case. Snake case instead
**Brian Coords:** No kebab.
**Aurooba Ahmed:** Or kebab. Yeah. Oh yeah. Snake case is when it's an underscore. Mm-hmm.
**Brian Coords:** underscore. Mm-hmm.
**Aurooba Ahmed:** Yeah. Yeah. So you'll like, they'll expect that will be in Camel case instead of in the kebab case. So there are some gotchas like that, that you just wanna be careful of when you're dealing with attributes inside React.
But as long as it's not a reserved class, a sort, sort of a reserved name, like className, just putting it indirectly will work.
**Brian Coords:** And. You mentioned that your, your code editor can kind of take care of some of the stuff for you, or at least give you warnings about it. And so like that's one of the useful things you can also do. Um, well, I know that. We started a bit of a conversation about ligatures and your coding fonts, and some of us have opinions on that.
Me. Some of us have opinions on dark versus light mode. Okay. Me. Um, and some of us have opinions on like code formatters that clean things up for you. Again, also me. , these tools are super. Super useful. Um, but when you're collaborating you also, you know, learn that you have to work with other people and stuff.
So it actually is really helpful to have those things turned on that can just kinda give you that extra stuff. Oh, you cleaned up that little space right there.
**Aurooba Ahmed:** Yeah. Yeah. Well, if I had my formatted, no, I have it turned off for you.
**Brian Coords:** What? No, I turned it on to match you. Now I have it on.
**Aurooba Ahmed:** Okay, I'll turn mine back on after this episode. Uh, yeah, that was bothering me. The space, which my prettier would've normally just fixed it for me on save.
**Brian Coords:** Yeah,
## Doing expressions inside attributes
**Aurooba Ahmed:** so one of the things that we're doing here is we're actually using an expression straight in our className. Now, you don't necessarily have to do that. We could actually do the expression and logic before the return statement, but sometimes when the expression is so small and so tiny and so specific, I find it just easier and cleaner to do it right there in the element, even if it makes the ultimate code look a little bit, maybe a little messy, you know?
**Brian Coords:** Yeah, so like. If we wanted to have additional classes, that's when it would get a little tricky. And that's when maybe className would be a string with like back ticks around it where we could put in some content and then we could put in a variable that we defined somewhere else. And that's kind of a common thing you'll do.
So there are different ways to pass things to className, but if you are in this very simple situation where you can just do the logic in that spot, I mean, why not?
**Aurooba Ahmed:** Yeah. Yeah, and I mean, we're using the back ticks of above of here, right? Where we have the ID, for example. Mm-hmm. Right. We have VS dash accordion dash panel, and then we're passing it this dynamic variable ID to finally create that right here. We don't have to do it here, but it's such a simple little thing that it just makes sense to do it and.
Do it in one line of code rather than have than two lines of code. One where you're creating a variable or a string and then passing it here, right?
**Brian Coords:** Do you have that WordPress, like PTSD, that you have an ID equals a string and there's no like escape attribute around it? Like
**Aurooba Ahmed:** Yes.
**Brian Coords:** the escape attribute needs to be everywhere.
**Aurooba Ahmed:** yeah. And like, why aren't there squiggly lines? It's not, it's it's not warning me. Oh no. Is my PHPCS not working? Yes, that
**Brian Coords:** this string that I literally just wrote gonna be a security vulnerability? Yeah.
**Aurooba Ahmed:** And security and react is also, it's a pretty interesting topic, actually. Something that maybe we can cover another time.
**Brian Coords:** Yeah, definitely.
## Adding CSS to our component
**Aurooba Ahmed:** So this adds all of the logic in the right places, but it doesn't actually work because of the fact that all we're doing now is adding classes. Right? So then that means that's where the CSS comes in.
And this was. Mm, like 1, 2, 3, 4, 5, 6, 6 lines of CSS. In order to make it work, essentially the first thing we have to do is we have to make sure that the panel is hidden because we don't want it to show. So I'm targeting any, any panel, any Div that has the VS dash accordion dash panel as the beginning of its id.
That way we can target, you know, the zero one, whatever we added there as the pattern, and then we're saying, Hey, Don't show it, display none. However, as soon as that Div gets the is expanded class, then we wanna show it.
**Brian Coords:** And that. Is expanded class, like just the naming convention of that, that's also very WordPress. Um, in WordPress, blocks do get things like is selected, has background color, you know, so is, and has classNames start to become like a default if you're dealing with a lot of stuff, uh, as you move into WordPress.
**Aurooba Ahmed:** exactly. And. I think when you look at this code that we've written, a lot of it has those styles of WordPress in it. Because you and I write so much WordPress code, and so we start thinking of our classNames and our variables in that same sort of style. So if we go back here, you know, and we notice when I open this, that's when the class is expanded, gets added, and then when I close it, The class attribute right now is still there, but it's empty.
So if I wanted to keep this even cleaner, I would actually make it so the class attribute doesn't even show up when it's not needed. But in this case, we just left it there.
## Prop drilling
**Brian Coords:** I think there's one thing that sticks out to me about the code that we wrote very quickly before this episode. Let's go back to the code editor and I wanna get your opinion on something.
**Aurooba Ahmed:** Mm-hmm.
**Brian Coords:** One of the things that seems to happen here is we have, is expanded and it gets passed from the app. To the accordion item. Then it gets passed from the accordion item to the accordion header and the accordion panel. And then we do all this logic there. And that's because our app is really in charge of determining which one is expanded.
So like that's important for that to be passed down, but then you get to this place where then you gotta pass it here, and then he has to pass it here and she has pass it there and he keeps going down. Um, and there's a term for that. Can you give us like, like what is that? Why is that? How do we feel about it?
**Aurooba Ahmed:** Yeah, so the term for that is called prop drilling. So you're starting with a prop that's, you know, in this case it's the, is expanded. It starts out in the app js file, and then it gets passed. Drill down, right? It gets, you're going into the accordion item and then you're going into the accordion header and also the accordion panel, and you're drilling down into your components inside your app and passing it down.
And sometimes you're passing it down because you started it in like the top level, but you actually need it in like child four or something, like a few, a few children down. But because of the way it all works, you can't do the logic of that inside. You have to do it at this really high level. So It's a very common problem. And so there's a bunch of different ways to solve it. Which is the first that comes to your mind now that you, you know, you've been doing so much React stuff recently.
**Brian Coords:** Well, there's solution zero, which is to do nothing about it and to just pass some props down. And I think when you're at this. Part of a project, and this is maybe about as big as this project is gonna get. That's what I would do. You know, I would just let, I would just pa like, I wouldn't really change this particular example.
Um, I think the next
**Aurooba Ahmed:** list. I never pass anything else. I just drill it down, you know, in my super list block. Yeah. Because it's, it's three, three elements and I'm just like, am I really gonna create something special just to be able to pass these? No, just drill it down. No big deal.
## useContext vs Prop Drilling
**Brian Coords:** Yeah. Or you import. A hook, which would be context.
**Aurooba Ahmed:** Yes.
**Brian Coords:** as like the next, that would be like what would we consider maybe the next step upward, which is use context.
**Aurooba Ahmed:** Yeah. Yeah, and I think it's awesome. There was a time when context wasn't as powerful or even as integrated into React, and so people used things like Redux, which helps you manage like global state. And when you're playing around with Gutenberg, you're gonna come across that term quite a bit because there's something similar like that inside Gutenberg in the block editor.
But the context is really nice when you have something that you need to just keep globally available because it needs. To be accessed in a few different places and it's just built into React, and then you're able to use it to. You know, get the information where you need and not have it where you don't need it.
So for example, accordion item is really just a bridge, but it doesn't need to use is expanded itself anymore. So if you were using context, we would just not have it's expanded here at all. And instead we would just import the, it's expanded argument or prop in directly in header and in panel from our app js.
But doing that, It takes a little while before you get there and in, in a lot of blocks and a lot of like small react programs or you know, applications. You really just don't need to get that fancy and adding that extra dependency of managing a context and creating hook for it, I mean, it's only worth it if you need to do it a lot or if you're dealing with a really gnarly application.
**Brian Coords:** I think a good analogy is, If you are coming from the WordPress, PHP background, there's global variables. So in WordPress, like Post is this global variable that you can access from anywhere. You just have to say global post, and then you can use that post object variable anywhere in your code and and it's great and it's super helpful.
And you can define a global variable, but it's really frowned upon. It's really seen as like a, don't use it unless you absolutely need to. And I think, I'm not saying that that's what use context that's frowned upon or don't use it. It's just like, it's a very powerful thing. So if, and it, if you're going to use it, you just wanna have a good reason for it.
And if you're dealing with something small where you can just pass an argument from one thing to the next, you're probably okay just doing that. And you know, it's not something to stress out about.
**Aurooba Ahmed:** Yeah, I think there's something to be said about trying to stay as simple as possible and only introducing complexity or a more complex like feature when doing that is simpler than what you were doing it before. So in this case, adding context, adding a hook, all of those things, it doesn't make sense to do it because it's a lot more complex.
Like it's a lot more complicated to do that in order to do something very simple. Whereas here we just have to go and drill a prop, one extra file down. That's not a big deal. So, It's really important to just not get super complex super quickly, which is something I see a lot happen.
## Attempting to remove a component
**Brian Coords:** Because I think we even talked about how the accordion item component here, like probably doesn't need to exist. Like I think we had all these ideas, like this is just a component that's just wrapping two other components and it really has no logic at all to it. And it maybe you would want this, but like at this stage, like we could have even skipped that.
And then the prop drilling wouldn't even be an issue because like this would just sit in our app, you know?
**Aurooba Ahmed:** Yeah, and it, it really could, I mean, instead of having all of this passed down here, I could technically, okay, so I pushed, I pulled it in,
**Brian Coords:** There's a, a few things while you're typing. One is that we had to wrap it in like the little fragment tags because in J S X, you just need one parent element. You can't have like a bunch of people next to each other. You need a parent, um, around it. So we had to do that. The other thing is, um, I think there was a little bit of logic that we were doing or is expanded, was um, actually we were doing the logic in
the selector right there. So we were actually saying active ID equals accordion id. So we're, we'll have to use that. And I think the third thing we know we'll get yelled at for sure is that we don't have a key for our map. And so even though we're in a fragment, can you add a key to a fragment or no? Do we have to add a Div??
**Aurooba Ahmed:** No, I think we would have to add a Div
Okay, so we've added the accordion header and the accordion panel into our app js now, so we're not using accordion item, which means we actually have to pull in these two components into app js now instead of accordion item.
**Brian Coords:** there is a feature that will default do imports, and I'm not talking about copilot, but like, Sometimes your imports just show up at the top and sometimes they don't. And it's somewhere in VS code. I don't know what does it and how to get it to work all the time.
**Aurooba Ahmed:** I don't
**Brian Coords:** it matters
**Aurooba Ahmed:** Sometimes it does work and it's magic. Yeah, exactly. Uh, I, I love it when it does happen, but then sometimes because it doesn't happen, then I have to check anyway. And if I have to check anyway, I might as well just do it myself. It's like a whole thing.
**Brian Coords:** And honestly, Copilot just does it for me now.
**Aurooba Ahmed:** Yeah. Agreed.
**Brian Coords:** Spells something wrong or like names the file, something weird, but it like gets it, gets it pretty close.
**Aurooba Ahmed:** Yeah. Okay, so I think that we got everything and
**Brian Coords:** No,
**Aurooba Ahmed:** no.
**Brian Coords:** I see. One more error I think that you're gonna deal
**Aurooba Ahmed:** Expanded heading Content. Oh, ID right here.
**Brian Coords:** Yeah, that's the one
**Aurooba Ahmed:** There we go. Which maybe if I spelled it right, that would be good.
**Brian Coords:** I don't know if that matters.
**Aurooba Ahmed:** No, it doesn't matter. You don't have to get the variable spelling name right. The computer just knows.
Okay. So the one thing that we did miss was the expand had to also get passed down with the current accordion element. Function so you can just pass it down directly. The reason it was that that in the header was because initially the accordion item was getting passed this function, but of course now we have to directly pass it.
So now if I save this and I refresh, it will work. And now we've eliminated an entire component essentially, cuz we didn't need it anymore. There was no logic in it at all.
**Brian Coords:** Well, two things about that. Number one, there was a little logic cuz it saved us from having that. Div around it because now we don't have a key for our map and reacts not gonna like that. And I did say at the beginning of the episode how much I loved how we didn't have Div. So something to consider.
other thing I, if we were really gonna be nitpicky about this code is I don't like, That I named expand in the accordion header component. Expand like it, that's too confusing to me. I actually, like, if I were to rename that prop ex, I would rename it like onClick. Cuz that's what it really is. It's like a click handler or something.
that did confuse us that I couldn't pass, expand to expand. Cuz in one case it's a like, in both cases, like a function. So then it needs the function to function and
**Aurooba Ahmed:** Mm-hmm.
**Brian Coords:** if it was on click, I think we would've both default thought, okay, I'll put a function in here cuz it's an on click.
**Aurooba Ahmed:** Yeah. Yeah. So naming things is really important and sometimes you, sometimes one name makes sense as you're in the thick of things and you're writing something, but then when you come back to it, You find that, oh, I should have actually named it this, because that's what makes sense if you're not in the middle of building something very intensely so, but that, that's like a general programming creation tip thing that you have to keep in mind.
You know, that's not specific to React per se.
## Next Steps for viewSource and Season 2
**Brian Coords:** Well, I think the idea that you can do this 50 million different ways and you can explore different ways and you can try putting components different places, is actually the perfect way to talk about the big picture next steps of this series and the podcast in general, which is, you know, our goal with this was to turn it into a block in Gutenberg, which, you know, we are definitely going to do, but.
We need a lot of time to do that. I think because there's just like this, so many ways to do things. So many decisions. You have to make so many options. You can paths you can go down that, um, it's gonna be a big conversation to talk about turning an accordion into like actually making an accordion block in Gutenberg.
**Aurooba Ahmed:** Yeah, even the conversation of us trying to decide how we would demonstrate doing that was it. We were like, we could have do it. We could do it this way, or we could do it this way, or we could do it this way. So, you know, we definitely need more time than we have in this current season to do that.
**Brian Coords:** So we have a couple more episodes left. Not enough time to do it. Summer break is happening, viewSource will be on like summer vacation and take like some time away for all the fun things that happen during summer. And then in the fall when we start the second season, I think just building an accordion block will be, again, a recurring like
thread through not every episode, but like a number of the episodes. And we can, we can take step away from front end, just like react stuff and say, okay, in the block editor with all the WordPress packages and all the like, stuff that goes into it. Now how do we build our accordion?
**Aurooba Ahmed:** Yeah, and we wanna take that same approach that we've taken throughout this series of going really step by step, really talking through a lot of these small elements that seem small, but there's so much thinking and logic that goes into it and stuff that you need to understand around them that it's really important that we talk about them.
So when we start, we'll start kind of at this. Day zero of creating a block. We'll look at setting up the environment and some of those other things that we haven't done here for React, cuz we didn't talk about the scripts part at all. But then when we do the block, we definitely want to, because it's really important to understand the build process that is helping you create stuff.
Even if you don't work on creating the build process yourself, it is important to understand what it is doing and how it's doing it, you know?
**Brian Coords:** This was just one little piece of react sitting on a page. When you build a block, there's a giant React monster running, and you're like, Putting things into it, and you have to play by those rules. So that's a big part of it. But so much of what we talked about is gonna be really important foundational stuff like props and like prop drilling and accessibility and, useState um, components and imports and destructuring things and, you know, all that stuff is, is all there.
So it's all important stuff that you use when you build a block.
**Aurooba Ahmed:** exactly. Yeah. So, you know, we've talked about not just react basics here, but just some basics of modern JavaScript. For example, destructuring, which is not specific to React, it's specific to modern JavaScript and. When you're in that block editor ecosystem, everything is now mish-mashed and on top of it you have the block editor API.
So it was just so important to talk about everything, some of these basics outside of that API, which is a monster of its own, a very lovely monster, but a monster of its own. Uh, so that when we get there, it's a little less overwhelming when you have all these different things that you need to be looking at and connecting the dots on and using to create
this one seemingly simple component slash block in the block editor to make it editable.
**Brian Coords:** Yeah, because like the soup of like, this is modern JavaScript, this is Word or this is React, and this is WordPress/element, which is sort of like, their version of React, but it's pretty much react, um, like those layers stack on top of each other and, and that seems confusing. The benefit though, is WordPress comes with so many things that you don't have to think about.
So like all the UI, all the components that you can pull in, all the text editors and rich field editors, and there's all this stuff that's ready that all it takes one line to import it and use it and you don't have to think about it. So, um, it's gonna be really fun to like, Dig into this was a blank slate that we built up on, but now next season will be, here's this huge palette of things.
How do you like deal with all of it coming at you and turn it into something fun and it's exciting.
**Aurooba Ahmed:** Yeah, so you know, finally take everything we learn and take it into that CMS context, which gives you a thousand hour headstart on building something like this that's editable in a user-friendly context. But you also need a little headstart. You also still need to come with a little bit of knowledge that we've talked about here to be able to take advantage of everything.
I'm excited for that. That's gonna be, it's gonna be a really fun series, part two that we do in season two.
**Brian Coords:** Yes, and we have a few more episodes left of season one that I'm also excited about. So we will wrap these up with some really cool final developer thoughts for this year and this season one. And we'll do it next week when we come back.
**Aurooba Ahmed:** Yeah. All right. See you then.
**Brian Coords:** See it.
Visit viewsource.fm for the latest updates and links to the show notes. Review and subscribe to viewSource in iTunes, YouTube, or wherever you get your podcasts.
Creators and Guests
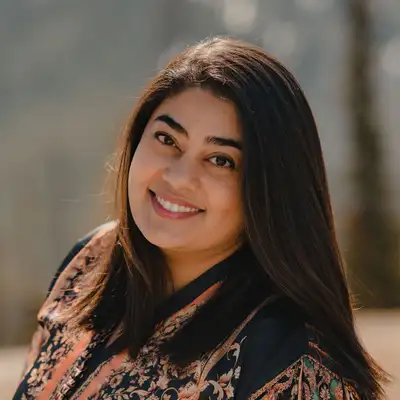
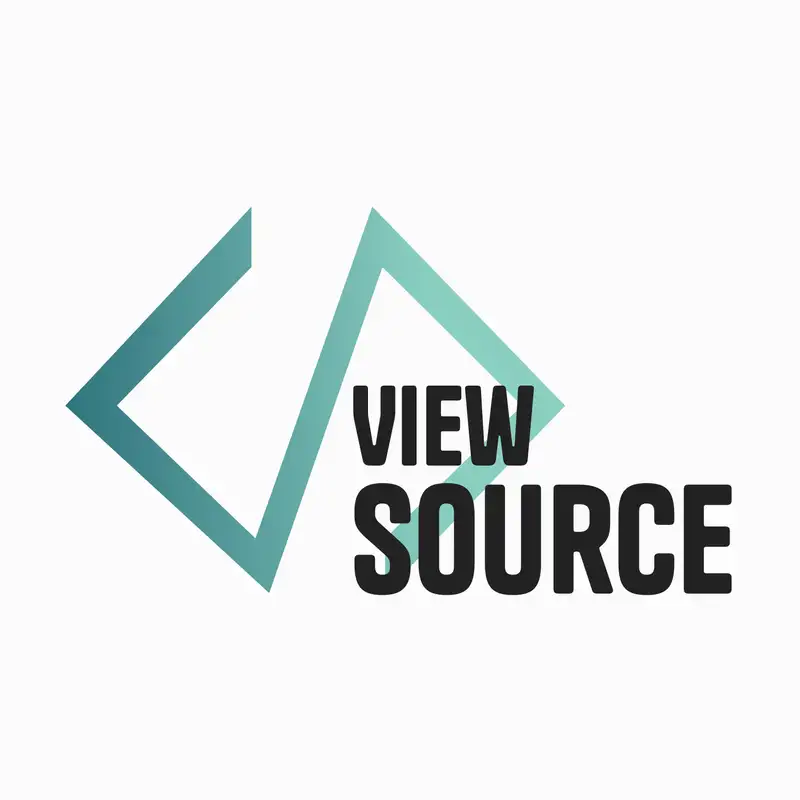