What is a React Component?
## Introduction
**Aurooba Ahmed:** You are listening to viewSource a conversation around tech, web development, and WordPress with hosts Aurooba Ahmed That's me and Brian Coords
**Brian Coords:** Are you ready for part two of, part one of our building a custom React component in WordPress, series. Are
**Aurooba Ahmed:** Yes, yes, I'm ready. So in part one, to recap, we looked at how to include React on your website on the front end, and we talked about how to set up React, you know, how it gets included and the function that is a component in React and spits out HTML. That's where we got two.
**Brian Coords:** Yeah. And we talked a little bit about build process and we talked a little bit about, um, JavaScript. So are you ready to take us back into the code so we can start looking at our React component and digging into the accordion block that we're about to build?
**Aurooba Ahmed:** yes. Let's get into the actual meat and potatoes of what was happening with the button When you open and close, when you click it to open and close and show the world text.
## The Return in React Components
**Brian Coords:** I also, I feel like I interrupted cuz you then explains like, this function has a return where we're returning HTML. Like, like that's a React thing, right? Like a React is like, we would call this a component and a component just gives us some HTML, like that's what it's returning. Like here's a chunk of HTML.
**Aurooba Ahmed:** That's right. In in React, anything, any component must return some sort of HTML. It can't just like have random stuff in it that it has to be HTML if you want it to show up on the front end. If you're using JSX, sometimes you'll write that like HTML in a funky way, but in this case, we're literally just writing some very plain looking HTML, all regular HTML works inside React.
**Brian Coords:** Yeah, plain. You, you said JSX and I felt like my face, like twitched a little bit. Like I felt, I felt it happen like, uh, yeah, I mean, You're returning your button and your paragraph, like I can see there's a button and I can see that there's a paragraph, but, you know, there's a little bit more going on. Like your ghost HTML elements that are like brackets with nothing inside of them. Um, you know, the use of the, um, this like, I don't even know what it's called, like the squiggly brackets. There's a name for squiggly brackets, but, uh, I'm not, you know, curly, curly, curly braces or something.
**Aurooba Ahmed:** That's it. That's
**Brian Coords:** like it is HTML. Like you could just return HTML. Like it doesn't have to be anything other than HTML, but in this case it is a little bit more than just HTML.
**Aurooba Ahmed:** Yeah, you're totally right. Just, just like when I was talking about PHP and PHP, you know, you often mix your PHP and your HTML together. Here you're mixing your JavaScript, your React JavaScript, and your HTML together. The logic and the view, the logic and the presentation are a little bit mixed, but you know, we can.
Add some element of separation here by doing most of our logic work above the return, like before the return, and then trying to do only a few conditional presentational things in our return function, um, or our return section. Really
**Brian Coords:** so like what are, you know, , big picture. We need to be able to click the button and I can see, you know, you have an on click and it is passed something called toggle button. And then we need our paragraph to like show and hide. So what, you know, what was the process like you said earlier, you have like kind of your return and your h at the bottom, but you do put some functions up at the top.
So
**Aurooba Ahmed:** Yeah.
**Brian Coords:** walk me through what that function is and, and kind of how, how that, how this works.
**Aurooba Ahmed:** Okay. So if you're thinking about this in like regular JavaScript, let's say you have a button and on click, you needed to do something, you wanted to show something else, you are probably gonna set up a variable where you're gonna track whether you know something is true or false. Like is this button clicked or not clicked?
For example, you, you could do it that way. Um, And that's kind of what we're doing here, where I've said, Hey, there, this is a button. And when this button is clicked, I want you to, you know, run a function called toggle button. And this is how you, you know, add a function inside HTML in React. You can just give it the function name.
You don't even have to put the, the two empty braces like brackets beside it. Uh, and as long as you put it in curly braces, it knows that this is something React. You know? And that function is essentially changing the variable that is tracking whether like it's a boolean variable that says set to true or false.
And it's saying, Hey, if I clicked it, if it was true, turn it to false. And if it was false, turn it to true. And then in my return function underneath the button on line 14, I'm saying, Hey, if the variable is open is true, then show me the world word in a paragraph.
**Brian Coords:** That's actually like WordPress. WordPress Coding Standards are very: Be verbose as much as you can. Don't like, do like fancy short like operators and things like that, but like your isopen && paragraph. What would be like, how would you explain to somebody like this is, this is making that happen?
Like
**Aurooba Ahmed:** Yeah, so
**Brian Coords:** work?
**Aurooba Ahmed:** In React you can do if statements, but if you try to do an if statement inside the return area, it kind of hates you . So it, it, it doesn't really like it. You can do it, but it's not generally, a best practice. So the && this, I think this is a no, this is not the ternary operator. It's just the && operator, right?
It doesn't have like a name, I don't think. Um,
**Brian Coords:** Noone's fact checking here. Don't worry.
**Aurooba Ahmed:** Um, you know, this is how you would write it. Like this is not, not verbose. This is just in React. This is that way. You would write it inside the return area. But if I was writing this above, not in the return function, yeah, I could write a little if function like, you know, if statement where I say, Hey, if you know, set, if is open is false, make it true.
If not, make it false. Um, but the, the, you don't, you don't have to like the rea, the JavaScript standards and WordPress are actually different from like the PHP coding standards I
**Brian Coords:** Yeah, so, but like essentially that line of code means like, it's gonna look at what's before the ampersands, and if it's a true statement, then it'll execute what's at whatever's after the ampersands.
**Aurooba Ahmed:** That's right. Yeah. So it says, Hey, if the isopen variable is true, um, then show me whatever I put after the double ampersand. And I put that all in curly brackets because it is a React statement and uh, we want it to be identified as React.
**Brian Coords:** Yeah, we don't want it to just literally print that on the page.
**Aurooba Ahmed:** Exactly, exactly. Oh, and it, well, if I, if I took away the re the curly braces and I were to save this, um, let's see what happens. Yeah. So it literally did, I'm not showing this on the screen, but it just literally shows is open &&, and then world.
**Brian Coords:** Because it thinks like it. It's thinking you're doing HTML until you throw some brackets and you say like, no, no, no. This is JavaScript, you know?
**Aurooba Ahmed:** That's right. Exactly. Yeah. Yeah. So that is a return function. But you know, there's a bunch of funky things happening before the return function.
## Setting up State with @wordpress/element
**Aurooba Ahmed:** So now we have, we come back to something called state. In React you can run, you can set up sort of a local temporary tracker of whatever you need. Track of. So for that, in React there is this thing called use state and it lets you whip up two different variables.
One variable where you're gonna track, uh, the information about something and then another variable that allows you to change what is being like, change the information, you know, update it. And that way you don't have to do it like directly yourself, it, you just pass it the value that you want it to change and it will change it.
**Brian Coords:** and, and there's like, you know, the idea of state is like you're gonna store a bunch of information and then you're gonna want to know that information and you might want to change that information. And that like turns into like a traffic jam when it gets really complicated and you want somebody that's in charge that's saying like, no, no, no, you don't change it cuz I just put it here like I'm directing this traffic.
I'm in charge of all of this. I'm gonna make sure you get the correct information and I'm not gonna make sure you're not gonna ruin my information. It's like, it, it kind of abstracts that and in this situation, it's not like say necessary, but like it would vary. You know, imagine we start doing like stacked accordions, like multiple accordions, and you close one and you open it up like it very quickly like becomes necessary.
So it's such a, like an easy, best practice to follow, but, but you're telling me that on line five isopen is gonna be our value, and then the second thing will always be a function that will
**Aurooba Ahmed:** Update the is
**Brian Coords:** update the value.
**Aurooba Ahmed:** That's right. Yeah. So here you can see it's a constant, but I'm give putting it inside square brackets because they're connected together. And that's how what useState, which is called a hook, requires you to provide. And then it's saying, hey, the first one is going to be the variable, like the, the
the place where we store the information and then the second word, whatever we're doing is needs to be transformed into a function that will, let me update that first one. And then we say equal signs, and then we use the variable useState to transform them. And the very first thing we can do in there is either:
leave it empty or pass it the initial default value that we want to keep track of, so in this case, because I want this to, isOpen to function as a boolean
And if I were to hover on it, you know, my little VSCode, helper tool tooltip will tell me isOpen as a boolean and I'm passing it false.
Because at first the button it like the isOpen needs to stay false. We don't wanna show the world text.
**Brian Coords:** Yeah.
## Destructuring and Getting Multiple Values from a Function
**Brian Coords:** And yeah, and I think even just the idea that like a function is returning more than one thing. Like that's, that can be a little like, you know, mind blowing on that because you're like, oh no, I just, it's a function. How is it returning multiple things, like, you know, and, and how am I setting those as, how is it not like an object?
It's, it's, it's two separate variables or, you know, that I can use separately. So like, those sorts of mental shifts are really required when you get interact. Cuz you'll have to start thinking like, oh, okay. The, the, the base layer of JavaScript of like what I thought I could do or what I'm used to if I'm, if I'm haven't really gotten deep into it.
Uh, like there's a lot of those things that until they're broken down, uh, for you, you first look at them and you're just like, you know, this is, this is so far from what I thought JavaScript was that I don't even wanna look at it, you know?
**Aurooba Ahmed:** Yeah, so something like this, this is very similar to the concept of Destructuring, which is when I started learning React, it just like blew my mind and it took me so long to wrap my head around because in React if you had an object that was called, you know, um, viewSource and let's say this object (and I'm just like making this up as I type here) and it has like, you know, hosts and then it has, you know, Aurooba and Brian and that's the first value.
And then it maybe has another thing that says, you know, um, Type, and that's called podcast. Now, if I wanted to pull out hosts and type from this view, source object into separate variables, I wanted to destructure it in PHP, you would be something like, you know, oh, let me create a variable called uh, hosts.
And then I would go equal sign, and then I would choose to say, oh, view source, you know, and then it's like an object. So you would like pull out hosts from it and assign it. And you can do that in React. Like that's just like regular JavaScript stuff. But in React, like you would actually, you could actually do something like set up a constant.
and, uh, put curly brackets here and say, hosts type and then equal sign the name of the object. And now it'll say, oh, I wanna take viewSource host inside, like the host inside viewSource, and assign it to this host variable. And the same thing for the type variable. And I used to look at that and I'm like, this makes no sense what is happening.
But it's actually a really, really nice way to pull out a multiple, you know, variables or information from a larger object, which you have to do a lot in WordPress Block development.
**Brian Coords:** Yeah, when you, when you get to like destructuring or spreading out arrays or like all these sort of like modern things, you start, like, at first it's so confusing, but then once you know it, it's like you're like, oh, I'm so glad I don't have to do this manually. Like , you know, I
**Aurooba Ahmed:** Yes,
**Brian Coords:** it's super time saver. But so, You know why?
I'll, I'll do one last question on it. Why would, um, why did you use brackets in your example, like curly brackets, but in the useState, you're, you're using square brackets.
**Aurooba Ahmed:** Yeah, so curly brackets in JavaScript and in React they mean that you're creating an object and. and that one was saying, Hey, I want to pull something out from an object. And that's why we had the curly brackets. But in the, the square brackets for useState this is actually just like a array, an empty array array with two variables in it.
And then we're passing that to the useState hook, which transforms them into the function and the variable. So we're actually literally passing an array of two values to it. That's why.
**Brian Coords:** Hmm. That difference between an array and an object.
**Aurooba Ahmed:** Yes, exactly.
## Calling Event Functions in JSX
**Aurooba Ahmed:** And then after that, you know, the only other piece we're here we have is an update function because onclick in the button, we pass it up function called toggleButton. And so we write out that function, which is also kind of trippy because here we have a function inside a function.
**Brian Coords:** Yeah, Why not just put, setIsOpen in your onclick on line 13? Like why wrap it in a function?
**Aurooba Ahmed:** Because if you don't put it in a function, it does, it tries to do it every single time it's on the page. It doesn't know that you should only do it on the click, even though you're passing it as part of the on click. So if you, if I was to do that and I saved it, React would tell me an error. Nothing would show up and it would be like, oh, there's too many re-renders.
Because it's constantly trying to keep track of what you're doing and what the HTML is, and if you try to pass it a function, that function is gonna to gonna try to happen every single time. So
**Brian Coords:** it's trying to execute
**Aurooba Ahmed:** instead,
**Brian Coords:** Instead of like getting it ready. Yeah.
**Aurooba Ahmed:** Exactly. Yeah. So instead we create a function call toggleButton. And then there I'm using the useState function called setIsOpen that we created.
And I'm saying, Hey, take the existing value of isOpen and pass it the opposite of it by using the exclamation mark. But I could also have done something like, if, you know is open, then set it to false and co-pilot is helping me write this really quickly. And if it's false then you know, set it to true.
So I could do that too, but it's just super fast to do it other, like the other way
**Brian Coords:** I think that's, it's useful just to see that though because,
**Aurooba Ahmed:** Yeah.
**Brian Coords:** It's like that whole verbose thing. Like sometimes it's just useful to somebody to see it and you're like, okay, now I know what it's doing, but like, I got to see it kind of like, it's really just setting it to the opposite.
But
**Aurooba Ahmed:** Yeah. It is nice to see it. You're right. And that's it. So now we have our little function that, uh, shows and hides world.
## Frontend Recap and Next Steps
**Brian Coords:** And so we exported it from our app.Js.
imported it back into our index js and we said, stick this, this function on the front end of our website on this div with an ID and it's empty. Stick this HTML, keep this little JavaScript logic running. Pay attention for my click, run my function, keep my state,
**Aurooba Ahmed:** yep.
**Brian Coords:** let's see.
And
**Aurooba Ahmed:** And when I clicked Hello, it showed World. And for this very, very simple thing, it seems like a lot of JavaScript, but as soon as we start adding all the other things, it's gonna be like, oh, you know, this is so much shorter to do in React than if you were to do it in jQuery or like just vanilla, JavaScript.
**Brian Coords:** Yeah, it's, you know, obviously if all you were doing is doing this, like yeah, it maybe wouldn't be worth loading React or something like that. But like we said, like you're putting it into a block, so like you kinda have React anyway and you're putting it, um, You're putting, say you're gonna start stacking 'em up and doing multiple accordions and you close one, it should open the other or whatever.
That sort of stuff. Um, that definitely makes a lot of sense. Maybe if there's things inside of our accordions, . Once the complexity adds up, um, then all those things pay off really.
**Aurooba Ahmed:** Exactly. Which is, you know how it works with like general development always. Even in WordPress, there's just a lot of stuff that, this is why people use theme scaffolds, right? It does all this work for you so that you can just get to the actual meat of meat and potatoes of what you wanna do.
**Brian Coords:** Mm-hmm. . And so what do you think is the next step? Like, give us a preview. What do you think is the next step that we should take for this, um, this React app? What would you wanna do with it next?
**Aurooba Ahmed:** I think that we should make an actual accordion panel without even worrying about the block part first in our next episode, and make it nice and accessible, you know. Make it a really simple, easy to use panel before we even turn it into a block. We need to know what it looks like in React and how we would use it before we get into the WordPress Block API itself.
**Brian Coords:** So, uh, like, so you're saying multiple collapsible elements in one.
**Aurooba Ahmed:** Yes, so we can have more than one that you could add. And when you open one, the other one closes. But also, right now what I've created is not accessible as an
**Brian Coords:** yeah,
**Aurooba Ahmed:** you know? So we need to turn it into something that is accessible as well. and how you do that in React if you were to do it we'll, we'll cover that as well.
**Brian Coords:** Yeah, we need to make it accessible. We need to make it extend. We need to make it a block. And you know, one thing that I always like that I'm already thinking like, okay, well if I'm gonna have multiple ones, each one, like I need a wrapper block. And maybe each of them should be an individual block or something.
So how is that gonna affect the fact that they're connected? You know, there's, I think there's like a lot of little things that are like slowly gonna dig into how you would render it, how you would use in your blocks, um, how you're gonna keep it accessible. Um, so I'm excited to see where it goes next,
**Aurooba Ahmed:** yeah. All right, well, we'll cover all of that, or at least part of it in our next episode.
**Brian Coords:** All right, see you then.
**Aurooba Ahmed:** See ya.
**Brian Coords:** Visit viewsource.fm for the latest updates and links to the show notes. Review and subscribe to viewSource in iTunes, YouTube, or wherever you get your podcasts.
Creators and Guests
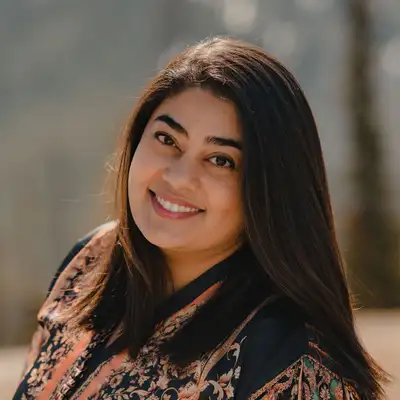
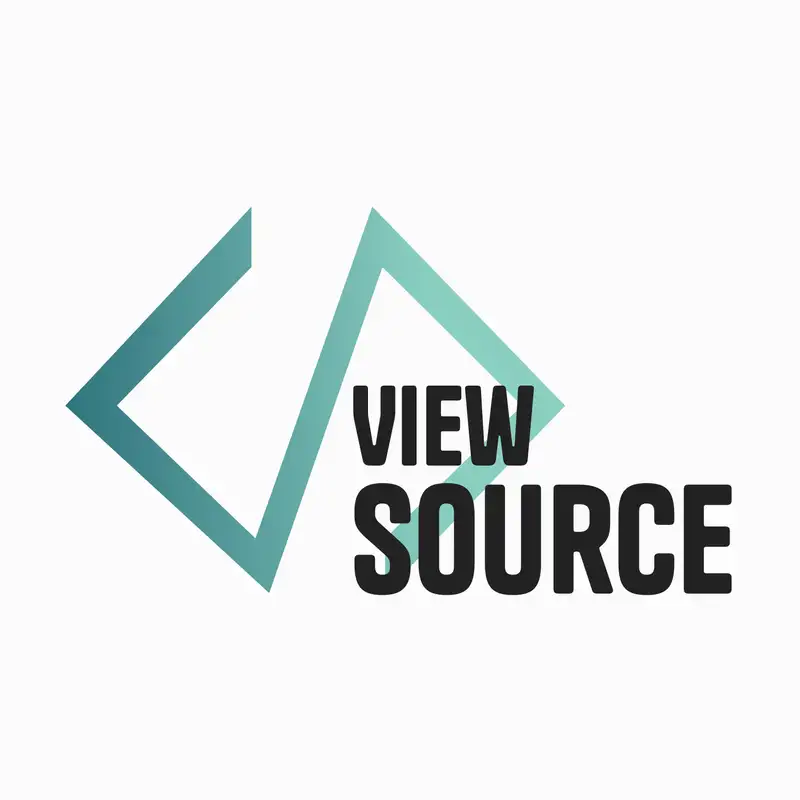