Diving into models and views in Laravel
Introduction
---
Brian Coords: You are listening to viewSource, conversations around WordPress and adjacent tech with hosts, Aurooba Ahmed and me, Brian Coords.
What are we building with Laravel?
---
Aurooba Ahmed: All right. Brian, I'm so excited to dig into a little bit more of the meat and potatoes of Laravel with you today. Are you excited to show me around?
Brian Coords: I mean, if there was real meat and potatoes, I'd be more excited, but uh, I will be very excited to talk about this. Should we recap where we left off last episode?
Aurooba Ahmed: Yeah. So, I'm gonna recap it. Let's see if I remember right. So, Basically, we initialized Laravel and it sort of has a lot of stuff that already sets up for you. And, you know, we talked about the ecosystem of Laravel and how much stuff it comes through, comes with, right? That you can just sort of integrate and just starts working.
And, you know, the thing that we're building is kind of cool, but today you're actually going to show us a little bit of the actual thing that we're building, right? Because we're starting to get into that now.
Brian Coords: Yeah.
So we scaffolded a project in Laravel. We talked about it. You know, I think we're going to continue the thread of like comparing things to WordPress as like a good mental model for us to kind of explore around. And so this episode I did push a little bit of code, a little bit of like features closer to what we're trying to build.
And,
Aurooba Ahmed: Mm hmm.
Brian Coords: I'm excited to dig into it. Mm hmm.
Aurooba Ahmed: Yeah. So to recap for anyone who's just jumping in, the thing that we're building is sort of an episode suggestion voting area, and it's going to be live, you know, we'll leave it for people to actually use and try out. And I'm kind of excited because it's the kind of site or it's the kind of like coding thing that you see a lot of. you know, product hunt, Reddit, a lot of these places, feature request areas, roadmaps, they all sort of function with the same sort of functionality.
So it's, it's common. But it's not the to do list, which I really like.
Brian Coords: What are you, are you implying that one of us suggested we do a to do list app? Because I would have, I would have never suggested such an outlandish idea.
Aurooba Ahmed: It's not outlandish at all, it's just that it's always the one you see, so I'm excited that we're doing something slightly different, but still pretty common.
Brian Coords: Yeah.
Well, and it's like, when you do something like this, you want something really simple, something that everybody like understands, but yeah, also isn't, is practical. So this one fits really well into basically the model of the way that Laravel works and that a lot of kind of PHP frameworks and other frameworks work which is the MVC model.
So I guess we should start by just explaining that model and kind of use that to look at Like what it means.
Aurooba Ahmed: Well, why don't you show us what it looks like first? Give us a visual, and we'll talk through the visual a little bit, because I think that will help us talk about that MVC model even better.
Frontend Demo and Laravel Breeze
---
Brian Coords: So I'm going to be sharing my screen. It's going to be my local development environment. There's just a local copy of this tool that we're building on Laravel. What's really nice is when we do deploy it, it's going to be a very seamless process because there's a whole ecosystem for that. So on the screen, what we're looking at here is the front page of this application that we built.
And what the front page is going to show us is there's a header across the top with the view source logo and a login and register link. And I was actually even just talking to somebody about this, you know, the idea that Laravel doesn't come out of the box with user login, you know, UI and chrome, but like it really does.
And so we, we kind of covered that last time, but like the, this like login. And I'm like trying to click on the actual screen share this login and registration page. You know, this actually was just built with
Aurooba Ahmed: Breeze,
Brian Coords: a, with Breeze, Yeah. And it builds all the UI. It gives you tons of this beautiful tailwind.
So all of this is going to be based on some tailwind, which I'm very excited about.
Aurooba Ahmed: And I really like that it sort of gives you, it's, it's not the prettiest UI, but it's also not ugly. Like, it's a really nice, simple, clean UI that if you wanted, you could really run with. And it gives you a really fast runway for setting up a prototype, which is kind of essentially what we're doing right now.
Right?
Brian Coords: Yeah,
And I think that's like what people say. It's like, it just gets you started a lot faster. So on here we have "view source episode suggestions."
MVC - The Model
---
Brian Coords: And as of now we have three suggestions that have been added and then at the bottom a link or a kind of a warning that just says "if you want to add a suggestion or vote for a suggestion, You have to be logged in and create an account." But you can at least view all of the suggestions on the front end without an account. So should I break down kind of like what each one is?
Aurooba Ahmed: Well, what I'm seeing is a list of three suggestions, right? And then the first number in each one, I'm assuming, is the number of votes it got. So for the first one, I'm seeing three votes, and it has the title "Finish That Accordion Block", and you can see who submitted it and at what time and date they submitted it.
Brian Coords: And yeah, and so that's essentially like the model of data. It's, it's a single suggestion, which has: the actual text of the suggestion, the number of votes it's gotten, the user ID of the user that submitted it, and like a date and timestamp for when it was added. And it's like really those four pieces of data together to make a user suggestion.
Aurooba Ahmed: Sweet. So yeah, that totally makes sense. And that sort of leads us straight into talking about that, you know, architecture MVC, which stands for model view and controller. So what you've sort of just described is that model, right? And that model is going to be the basis for a lot of the data that we're going to be looking at when we look at the code.
Brian Coords: Yeah. And so at this point we actually have two models already in our app. We have a user model. So that's like a user is a model like a user has It's a one central kind of piece of data. So it has like the name, last name, you know, email,
address, password, all that stuff. Yeah.
So that's the user model. Now we have this suggestion model.
That's going to have all of that kind of data, the text, the date, everything. That's another model of data. And then as you build out your application, you'll start tying things together, like connecting two different models or, you know, just like we've done here, we've connected the suggestion to a particular user.
But really it's just like, it's just like one model of data, I guess.
Aurooba Ahmed: Yeah. And I mean, you know, I think any kind of application or piece of software has to have models, right? So even in WordPress, we have the user model and we have like the posts model, which is like the basic version of, I mean, a suggestion would be a type of post if you wanted, if you were doing this in WordPress, right?
Brian Coords: Yeah?
Like in WordPress, we'd have a WP_User and we'd have a WP_Post and we would have a custom post type called suggestion. And every, everything other, well, the date, the, the, the author, I guess, you know, would be in that post table. But once you get to like how many votes it has, that'd be all post meta and everything would go into that post meta table, right?
But with Laravel, it's a little bit different because for every model you get to spin up a custom database table and it's.
Aurooba Ahmed: Right.
Brian Coords: Super easy and super flexible. And so you actually get to build all of that from the ground up, but with like, not a lot of code involved.
Aurooba Ahmed: Okay, cool. Right.
MVC - The View
---
Aurooba Ahmed: So, you're always going to have a model, and then the next thing in the, in the framework is, like, the V, which is a view, which I think is safe to say is, you know, how do you take this data and present it, right? That's the, it's the presentation section.
Brian Coords: Yeah.
And I think what's like super important there is like the view really should not be thinking. Like, let's just say, let's say for example, I wanted to change the format of this date right.
here. That kind of stuff, I would actually do that in the model. I would say like, okay, give me another attribute on my model that shows the date formatted nicely.
And I would do all that thinking as part of the model so that anywhere I pulled in this model. I could render that date the way I wanted to. And the view is just going to pull it out. It's not going to like, I'm not going to go into my view and start doing that kind of thinking and logic and data manipulation and stuff.
And like. That's, I think, where maybe it gets a little different from WordPress, where sometimes, you know, you don't really modify what comes out of the WP post. You do that all in your templates, and like, you start getting a little trickier there. But really we're kind of separating that and the view in the MVC kind of framework is really just about showing it on the
Aurooba Ahmed: So when I think about like the WordPress side of it, like you said, you know, it's very mixed. But there are things like, for example, Timber, right, which tries to separate that logic from the presentation and sort of bring more of that MVC paradigm to WordPress. So, and I know a lot of people really like that.
Like, I know in Roots, right, Timber, no, not Timber, like Blade, Blade is in Timber, in Roots and sort of helps you try to do that as well. Right?
Brian Coords: Yeah. So blade is definitely something that comes out of Laravel. So we'll, we'll look at that when we look at the code and we look at the view, you'll get to see blade and you'll see why people want to bring it to WordPress. It's. Basically just like a nicer way to write PHP is all it is. Like it's a lot less opening and closing PHP tags.
That's what it is.
Aurooba Ahmed: Okay, cool.
MVC - Controller
---
Aurooba Ahmed: And then the last part of that framework is really the controller, which is that logic piece, right?
Brian Coords: Yeah. Like a controller is more about I want to manipulate data. I want to handle, you know, for example, when I log in, I'm going to look at a view. I'm going to put in my user information and, but the actual processing of like, okay, is this an authorized user? Let's check. Let's make sure their password is correct.
Let's send them a notification. That's all in the controller and that's what's really doing a lot of the thinking. And so you'll often see When you start building out, you know, each model, you'll generally scaffold it with a controller to kind of, that kind of goes with it. And that works together with it.
That handles the logic for that model when you're like adding a new one, you know, deleting one, that sort of stuff.
Aurooba Ahmed: Right.
And so, when I think about that, I think about the first literal example that comes to mind on the WordPress side of it is when you have AJAX submission. Because, you know, when you have AJAX submissions for like forms and stuff, you do end up doing that kind of separation a little bit because you have your, your...
have your presentation view, like the template that's doing it all. But then AJAX requires you to have that function very separate from your presentation area, where you then run that logic and then return data. So it's a place, even though that's not how WordPress generally works, that's maybe a good comparison that we're all kind of used to.
Brian Coords: Yeah. It's like, you can only go so far with the comparison to.
WordPress because WordPress, you know, at the end of the day is, is. It's such a, it's, it's Not. in the, you know, it's just different.
Aurooba Ahmed: Yeah, for sure.
Brian Coords: I will make it clear that we're not saying that WordPress is like this. We're just pointing out places where it reminds us of WordPress.
Aurooba Ahmed: Yeah. And like, I think that, you know, when you are a person who's spent a lot of time in WordPress and you're exploring other technologies, I mean, this happens with anything, right? Not when you're learning new things, one of the easiest ways to learn something is to make, draw those comparisons with stuff that you already know.
So I think that as we're talking about this, it is important to be drawing those comparisons to WordPress or things in WordPress that we might be familiar with. Because it gives you a more intuitive understanding of those concepts, right?
Brian Coords: Yeah, definitely. All right. Should we dig into some code?
Aurooba Ahmed: Yeah, let's do it.
Artisan Migrations to set up Database tables
---
Brian Coords: Okay. So I'm going to pull up my code and let's take a look at a few of the things that we're going to kind of use and to build out some of the stuff that we looked at. So the way that it kind of works with Laravel is the command line interface is super robust. And what that means is you're, anytime you want to make something, you know.
You're generally going to be running commands in the terminal using their, their artisan, like kind of framework. And you'll spend a lot of time looking up things, but when you want to spin up, say a new model or a new controller or something like that, there's commands to do it. And as long as you pay attention to the way you name things, they will generally work together really well.
So for example, the first thing I needed for this was a database table. To store this information. So I ran a command that said, you know, make me a, my- they call it a migration, which is basically changes to the database. And so when you kind of load up, you'll, you'll basically have a ton of these migrations. And they're super handy because you, they basically let you like
make incremental changes to your database and you can run them and then you can un, you can like revert them and stuff and they kind of keep your database and the rest of your code kind of like in sync. And so in this case, I've created a migration to create a suggestions table to store all of our information.
Aurooba Ahmed: and what's really cool as I'm looking at this is that the name of this file is the date Of when you created the migration and then the actual like thing that you're doing. So, that's really cool. It's like you have sort of a file based history of all the different ways that you've modified that database structure.
I like that. Wow.
Brian Coords: like most of this code. And the file name and everything was created for me. I'm just adding these lines Right.
here, where I'm basically saying like, you know, I want my table to have an ID. I want my table to have like timestamps, like created at updated at timestamps. And then a few different columns that are, you know, kind of important for me to have.
And if I run the migrations backwards, like I want to revert all my code back a few commits, it'll know to just remove that table. And You know, it kind of makes deploying and pulling back things super handy.
Aurooba Ahmed: Right. So if you then create a migration to add a new column, just a new column to this database table, would that reverse function that's called down know to drop just that column?
Brian Coords: Yeah. So like you would basically write, you would write here, like add a new column and you would write the code for that. And then here you would write to remove that column.
Aurooba Ahmed: So that logic you're creating yourself, that rdesign command is not creating that for you.
Brian Coords: No, I'm kind of choosing like what to do in the up and the down. So in this case, I'm just dropping the whole table because I'm adding a table, but say later we add a column. I will, when I write to add the column, I'll also write a line that says remove the column because you never want like an extra column and then you pull back some code and then it's like, this column doesn't have a default value and nothing's, you know, it's like, you know, it keeps all that in track. But this essentially builds out the model and it's like normal
SQL columns, like I have a long text column for the suggestion. I'm using their foreign ID to basically link it to a user ID. And, you know, I won't go too into details, but basically it's going to like, it's going to know that it's a user, like it's, it's very smart because I'm using the same name of like user and user.
It's going to know that user ID actually refers to the users table. Like it's, it's. It's just going to do all that stuff like automatically.
Aurooba Ahmed: Yeah. I think that creating relationships smartly like that by having those similar names is a fairly common paradigm now. For example, I know we did a bunch of work with Supabase earlier this year, and they also use the same, like, relationship, like, smart, intuitive, you know, way to connect things.
So, this whole concept of if you want something to be connected name it the same thing is just really smart and really, like, clean, I think.
And then after that you have the likes column, which is an integer, and then you have your timestamps, right? So those are all the, all the different columns to this table.
Brian Coords: Yeah. And that's kind of everything we need now. And if we want to extend it, we'll just make a new migration to add a new column or I've done like, like I've needed to change the type of a column, that sort of stuff. So super handy.
Aurooba Ahmed: Nice.
Brian Coords: So when I generated this. I actually said, I actually told it in one command.
I basically said like, make me a model of a suggestion, make me the, the migration, like to help me set up my database table for it. And I think I also asked for a controller, but like I, you do it at once and it makes all of them for you. So when it made me this migration, it also made me a like a scaffold kind of framework for the model itself.
You ready to look at that?
Our New Suggestions Model
---
Aurooba Ahmed: Yeah, let's do it. So the model, to remind everyone, is sort of the data and how you're sort of setting that up, and that's going to be very close to essentially, in this particular case more, more especially, very close to the data that we're setting up for tables, right?
Brian Coords: Yeah. exactly. So really by making the model, I don't, it's like, it's just blows my mind. And I'm just like, I don't ever have to say anywhere, like where it's being stored or how to store it. Like, it just knows that the suggestions are like connected to that database table like it, it just blows my mind.
If I want to know who the user that submitted the suggestion, I can use class like functions like this belongs to, and just pass it a generic user model. Like just took the class, but you're just passing it the generic class. And it's going to know, Oh, there's probably a user ID table that connects this ID to that ID, and it's going to like do all that logic.
So my suggestion model basically can now grab the user that it's related to. And like All this stuff just like works. It's, I don't know. It always blows my mind.
Strictness in a Framework
---
Aurooba Ahmed: So, when I look at that, and you know, as we're talking about this, what this says to me is that Laravel is a very strict framework. It's a strictness that, for example, WordPress does not have, right? It, it, and because it's so strict, it's able to make a lot of these assumptions and make your work more efficient, right?
If it wasn't this strict, if it wasn't relying on this very particular way of You know, setting up your file, setting up your code, writing your functions, it wouldn't work. That's why in WordPress, it doesn't work because it's so flexible. Anything can be done in any possible way. But then there are a few things like, for example, when you're creating a new table, like a posts table on your admin side, you, you know, extend that class of tables, post tables which I'm forgetting the name of right now off the top of my
Brian Coords: class list or the
Aurooba Ahmed: Yes, the list, the table list, exactly.
Brian Coords: Yeah,
Aurooba Ahmed: Yeah, and there's like functions in there that you can rewrite and it will intuitively know that this is the version that you should be using. So, we have a little bit of that in WordPress, but that's not very typical. Whereas here, it seems like that is woven into the very fabric of how Laravel operates.
Brian Coords: Yeah.
What it means is that doing things is a lot faster because you don't have to write all that plumbing yourself to connect things and, and to build out that stuff. What it on the other hand means is that you have to know, like you have to learn the rules, like the rules make everything faster and work together, but like it's definitely an, an onboarding, like learning experience to learn the rules and to learn, you know, what is the way to accomplish this.
You know, since I can't just like write a query, you know,
Aurooba Ahmed: That also makes collaboration a lot better though. You know, it means that like people talk about one of the advantages of WordPress being that a lot of people know WordPress and you can like hire and makes hiring really easy. But even though you can hire a person who knows WordPress, there's no guarantee that one person A writes, writes WordPress stuff the same as person B. Whereas in Laravel
some of that is going away because the framework literally requires you to write in a very particular way. So that's, if you sort of jump into a Laravel project, if you're a Laravel developer, there's a lot of stuff that you can just like automatically know how to do and you can rely on. Because without it, it just wouldn't work.
So that's really, that's really neat. I think that's really smart. Yeah.
Brian Coords: really cool. It does not mean that you can't write bad code or insecure code or non performant code. All those things can definitely still happen for sure. But like everybody is generally doing things the same way, like big picture. And, and, you know, like there's just a base level of kind of like ways to do things.
And so he said, Yeah,
people can, yeah, Cause, oh man, like you pull in somebody else's WordPress project and you find out like, Oh, that's how they did that. Okay. All
Aurooba Ahmed: yeah.
Brian Coords: That's a,
Aurooba Ahmed: And you don't know where they might set up, because like, you could take a theme, but how one person structures a theme might be wildly different from how another person structures a theme. But here what I'm seeing is, because you're using that artisan command, and there's a lot of stuff that's built in, you know the models are gonna be in the models folder, and you know the migrations are gonna be in the database and then migrations folder.
You know, those things are very deeply intuitive and very given. That does mean that, you know, you know, the, the barrier to entry is higher, technically, but in a way that makes that effort worth it, I think, like, I can clearly see such a strong advantages of this, you know.
Brian Coords: Yeah. I mean, you definitely understand why it serves like the func- like if you, if you've ever like tried to fight WordPress, then that's maybe a situation where something like this would be better. Whereas, you know, there's, there's advantages to both. That's all I'll say. Yeah,
Aurooba Ahmed: no, no, for sure.
Brian Coords: all right.
Query Builder
---
Brian Coords: Should we look at the view and like how we're basically getting these and looking at them?
Aurooba Ahmed: Yeah, let's do it.
Brian Coords: Okay. We're going to take a very side, quick side trip to the controller. Just, I'm not going to like dig way, there's like, you know, we could talk about how do you route? How do you get to different URLs? How do you, you know, there's a lot of different things. We're going to skip all of that. I think we'll get more into that next time when we talk about controllers and routing and submitting forms.
I'll just do a very quick thing. Here's just an example of how we're querying our suggestions. We're just grabbing the class. We're passing, you can pass like all sorts of like query parameters. In this case, we want to order it from like, you know, the most likes to the least likes,
Aurooba Ahmed: Mm
Brian Coords: and then we're just getting it and we're going to pass it to our view.
Aurooba Ahmed: How Short and tiny and like so clean it is. I just love it. It's so clean, you know building this query. It's literally the suggestion class and then you have two colons because it's a class and then you have the order by and you tell It what you want to order it by and what what? You know if it's ascending or descending and then you say once you've done that just give it To us so that we can do things.
It's so, it's one line of code. Literally this logic. I just, that's beautiful.
Brian Coords: Do you see one server performance issue that we could run into?
Aurooba Ahmed: Well, you haven't limited it.
Brian Coords: Yeah.
So the good thing is once you get to the world of like pagination, and so you need to start paginating these results or adding searches or filtering and all that sort of stuff. So that also, there's ways to do that, to build this query so that it also returns you like print pagination data and returns you like sets and it lets you know, you're on page this of that.
There's all of that stuff like, like it's not in this example, but Oh, it's all there. It's it.
Aurooba Ahmed: It's like WP query, except you can do it on any custom table and you don't have to write it yourself fully. I love
Brian Coords: Yes. Yeah, exactly. Okay. But So.
Aurooba Ahmed: Well, no, go back to that for a second. So when you were looking at this view, what I'm seeing is a function called view, and then you're giving it two parameters. What are those parameters?
Brian Coords: Yeah, this is the, essentially the name of the file from the, like, folder of like, views. So, like, they kind of ignore, like, it's technically welcome. blade. php. They ignore all that. And then, and then you can pass it in an array of, like, just data. So, maybe I want to grab some other information and pass it, but, like, this will be...
It's kind of like a get template part in WordPress. Like here's the name of it, go get it. Here's some arguments to pass with it that you can use.
Aurooba Ahmed: Got it. Okay, cool. So that's the controller. It's controlling the logic of what view we want to show and what data we want to pass it.
Brian Coords: Yeah,
And so let's say like in the future, when I, when we want to like submit a form, we'll pass that to the controller and it'll handle that and render you back to a view saying, yay, you submitted your form, you know? So this file will ends up getting a lot bigger.
Aurooba Ahmed: got it. Okay, so now let's take a look at that view that we are passing this data to.
Views and Blade Templates
---
Brian Coords: So this is that welcome. This is that home screen that we saw. And so, I guess, you know, I guess big picture, what we're dealing with here is Blade. So, Blade is PHP with, like, almost like an extra build process, I think, because what it does is it lets you do things like write components and write these really like streamlined PHP kind of statements.
And then I think it processes it all when you're building it and then, you know, actually makes the real PHP file for you. So what you're writing is a lot quicker and leaner.
Aurooba Ahmed: now I know you have feelings about it, but as soon as I see this, I just immediately think of JSX.
Brian Coords: JSX. Mm
Aurooba Ahmed: Yeah, because it does a lot of that same stuff, you know, it lets you write those Reactified HTML components and does that in a cleaner way. That's a little, it's frankly a lot easier to write than if you were writing those components in like pure JavaScript.
And that's what it reminds me of, you know, because you have HTML, like, looking like things here, or PHP esque looking like things, but they're not actually full PHP or actual HTML, and then it's going to turn it into that after it's processed.
Brian Coords: Yeah.
and like. Yeah. it. is. I mean, it's like, these are basically components. And so in that sense of like a React or a JSX, like you're writing multiple components, components can be inside. Like this is All inside of like a general layout component that has like. The opening HTML tag and like, you know, it's like the header of the thing you can pass little slots and stuff.
Yeah, it is very similar to that, except like you can just really write, like I don't have to write class name. You can just write class. Like it's just regular HTML. And if you absolutely needed to, you can actually do like at PHP and then you can actually just write PHP in here and it'll like deal with it. So you, you
Aurooba Ahmed: is a provision to, like, add logic here, but obviously, ideally, you're not dealing with logic here, because that's what the controller is for.
Brian Coords: Yeah, The only logic you definitely will often use is like, maybe you're checking what URL the person's on, or maybe you're checking if they're logged in, so like here
Aurooba Ahmed: that's the...
Brian Coords: they're logged in
Aurooba Ahmed: That still falls in the realm of, like, presentation logic, which is its own thing, right? Like, that's not data logic.
Brian Coords: So. So in this, in this like view, we have access to that. This is the variable that we passed earlier called the suggestions variable. And it has like the array of all the suggestions that were in the database. We didn't talk about how I put those in the database. That's, that's a whole nother
nice, fun thing that we could talk about. But
Aurooba Ahmed: Yeah, we'll get into it another time, I think, for
Brian Coords: Yeah.
but I, I seeded the database with a couple of fake suggestions just to have it start which is another thing that they, they kind of set up for you. But Yeah.
I mean, it's, it's like, at this point, this should feel very PHP friendly, right? Like I'm looping through my suggestions.
I'm pulling out just those same attributes that we put in our database, right? Like the number of likes. The text of the suggestion, and then because I tied, you know, in the database, we just stored a user ID, but because I tied that user object, like that user model to it, I actually now can pull in like the full model of the user and just use that information.
So
Aurooba Ahmed: From within the suggestion instance?
Brian Coords: yeah. So like the suggestion now has the entire user model of the, the user that made the suggestion. So I can get their name or their email address or anything.
Aurooba Ahmed: That's cool. It's like one less query that you kind of have to do yourself manually because it's handling it for you. Nice. And something I'm noticing here is like, the comparison you made is to get_template_part. But when you pass parameters or like arguments to get_template_part, you still have to assign them to a variable because they just show up in the template part as a variable, as an array variable.
But here, you have to do that because you're just using $suggestions. But you pass it all as an array.
Brian Coords: So there's a few different ways you can get into it. So for example, in this case, this is this, the main view. So it already has access to the, like the variables that we passed in that array. It blade handles. Yeah. Blade handles that. So I don't have to say like args bracket, you
know. I don't have to do that.
Once you get to the place where like, let's say like. In a next episode, I actually wanted to turn this into a separate component because I wanted to be able to use it in different places on the site. Yeah. Like I, I just want to make a separate component. So you have that option. I'll show one here. Here's an example of a component like that, where you preface it with that X that basically says I'm a component and then you can start passing data as attributes here and,
Aurooba Ahmed: It's just like JSX! Yes!
Brian Coords: Yeah.
Aurooba Ahmed: Yeah, it totally is because you have like the X application logo, which is in the name of that element. It's a self closing HTML looking tag. In this case, you've also given it some styles, but then that's where you would give it the parameters too, right? Just like JSX.
Brian Coords: exactly. Yeah, and like, it, I mean, it, it's like
Aurooba Ahmed: Brian's all like, yes, yes, I will kind of admit to it, but I, I don't want to compare this because I don't really like JSX.
Brian Coords: I, JSX. has grown on me tremendously, but I would say this, to me, feels a lot more, like, readable. Like,
Aurooba Ahmed: JSX If you say so.
Brian Coords: Minus, okay, minus this, like, monstrosity of Tailwind classes, but you know.
Aurooba Ahmed: tailwind. Sure, sure. But it's fine for prototyping. So it's okay.
Brian Coords: Yeah, for sure. Like, you, we could abstract this stuff out if you want to. And I love, I do love utility classes, these sorts of, like, flex, justify between,
Aurooba Ahmed: Oh, yeah. 100 percent 100 percent Okay, so that's really cool. I mean, honestly, the more we've talked about Blade and the more I look at this, like, I definitely see a lot of power in it. So Timber is one that I have a little bit of experience with on the WordPress side, which,
Brian Coords: Okay.
Aurooba Ahmed: which uses Twig, but Twig and Tim Blade, like, they're kind of similar ish in some ways, but they also use that whole, like, at sign for the, for the, for the, For like, you know, the if statement or the for each, those kinds of things.
And I think that's something that, you know, you kind of have to start to get used to because my question always when I'm looking at these sort of templating engines is like, okay, but which one do I put the @ on or which one needs to be like in the double brackets? You know, those are things that you kind of have to learn and sort of absorb as you do it.
But I also know that once you know it, it's going to feel so fast and intuitive.
Brian Coords: Yeah, it takes a little time. Like I, that's what I was going to say about some of the components. There's ways like shorthand where like, say you want to pass, like. This we're here, we have to wrap it in brackets. Cause it's like, we want to run the
Aurooba Ahmed: You are
Brian Coords: but you can do things like where like you put a colon in front of it and then you don't need the brackets, but it only works like on certain types of props.
Like there's all sorts of like even faster shortcuts for how you can write this kind of stuff. Depending on whether you're using an HTML element or like an actual blade component. And so a lot of that stuff. Yeah. It's, it's just. If you're somebody who writes with this every day, I bet that it saves you a ton of time.
Aurooba Ahmed: Totally.
Coding Standards in Laravel
---
Aurooba Ahmed: And it also feels like, you know, are there coding standards for Laravel specifically, or are they just like, pair, like PHP pair standards or something?
Brian Coords: like PSR. The one I see a lot is like PSR4, I think.
Aurooba Ahmed: Right. Okay. Which is, you know, really a lot more modern than the standards that we're, we're like required to adhere to in WordPress. If you care about that kind of thing. For example, like, you know, and one of the things that always gets my goat is the fact that I can't use a short array syntax in WordPress.
It, oh my God, the amount of time that I've had to do is to like fix that and always be like, I shouldn't have to fix this. I have many feelings. So it's nice to hear you can do that.
Brian Coords: Yes, I will say I I've grown to really like, like, for example, when you have a function and the opening bracket starts on the next line, instead of starts on the same line that you start the function on. That's kind of a common, did. I,
explain that well, or did I not?
Aurooba Ahmed: No, you did.
Brian Coords: this thing where you start like that?
I'd never liked that. And like, now it's kind of growing on me, you know.
Aurooba Ahmed: I definitely don't like it. There are certain things I do like about how WordPress does them. And maybe it's because like, I was looking from like the world of JavaScript where we also typically all have that opening bracket, you know, curly bracket for a function on the same line. Although you can not do that if you don't want to.
Brian Coords: well, what's nice is like, since like, you know, notice here, like we're using much more modern, I mean, not even that modern, but like modern PHP, like namespaces and things like that. And like typing things. And so very often, like this would be typed to like the view thing for like what it's going to return.
So once you start like typing, like what you're going to return then this bracket down here is nice.
Aurooba Ahmed: Fair.
Brian Coords: But I'll tell you my trick that I learned this week while doing a lot of coding standards cleanup for a project when you're, if you have automatic, like code formatting turned on when you're in WordPress, just use your square brackets when you're writing it.
And then when you save it, we'll let your like prettier, whatever. It'll do it. It'll turn it into the proper syntax. So you can, you don't have to type the word array every time, like do a bracket and then I
hit save and then it turns it into the WordPress like full.
Aurooba Ahmed: You might have to show me that trick because I use phpcbf, like the beautifier, to like correct that, but mine won't correct that for the arrays for me. It'll do lots of stuff, but it doesn't correct the arrays for me.
Brian Coords: Okay. Yeah,
you can, I promise you my setup is terrible cause it breaks all the time, but we can definitely look into it. We should do a whole episode cause I need to figure out, I cannot get code
Aurooba Ahmed: We're going to do a thing on it for sure, at some point.
Brian Coords: So I'm dying, that that's my trick.
Next Steps and Outro
---
Aurooba Ahmed: All right. So, we've looked at the model, we've looked at the view, we've briefly looked at the controller, but we'll probably look at it more next time, right? So what are we going to, what is the next steps after we've set up the model, we've set up kind of this view, what are we doing next in this project?
Brian Coords: So I think the next thing we need to do is be allow people to actually submit a suggestion. So we need to set up a form. Where you can type in an episode suggestion, and then you need to be able to submit that form, and it saves it in the database. And I think we also need a form for like an upvote, where if you click an upvote, it submits a form to like process that.
Aurooba Ahmed: So it's really that data manipulation on the user side, right? That's what we're going to be doing next. And a lot of that is going to be in the controller, because that's where that logic piece comes in.
Brian Coords: Yeah,
so. There, you can do things different ways with Laravel. People. You people, a lot of people do like a React front end, Vue front end. Live wire is very popular. I think we're going to go very vanilla PHP and do like very traditional where we will actually make a route that you submit your form to, like submit a post request to a URL and it processes it by classic PHP.
It works really well. And then it returns you to a view. And so the controller will kind of handle taking that data and saving it.
Aurooba Ahmed: Okay. I mean, I think that sounds really solid and I'm looking forward to digging into that next episode with you.
Brian Coords: All right. Sounds good. I'll see you then.
Aurooba Ahmed: See ya.
Visit viewSource.fm for the show notes and if you're enjoying the show, we would love a review on iTunes or a comment on YouTube.
Creators and Guests
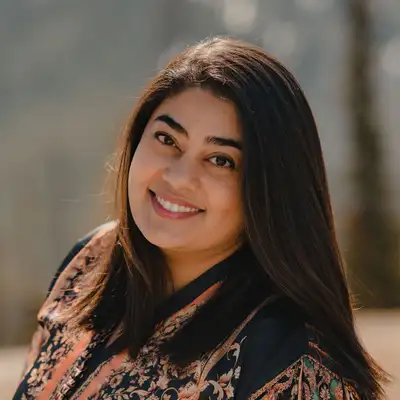
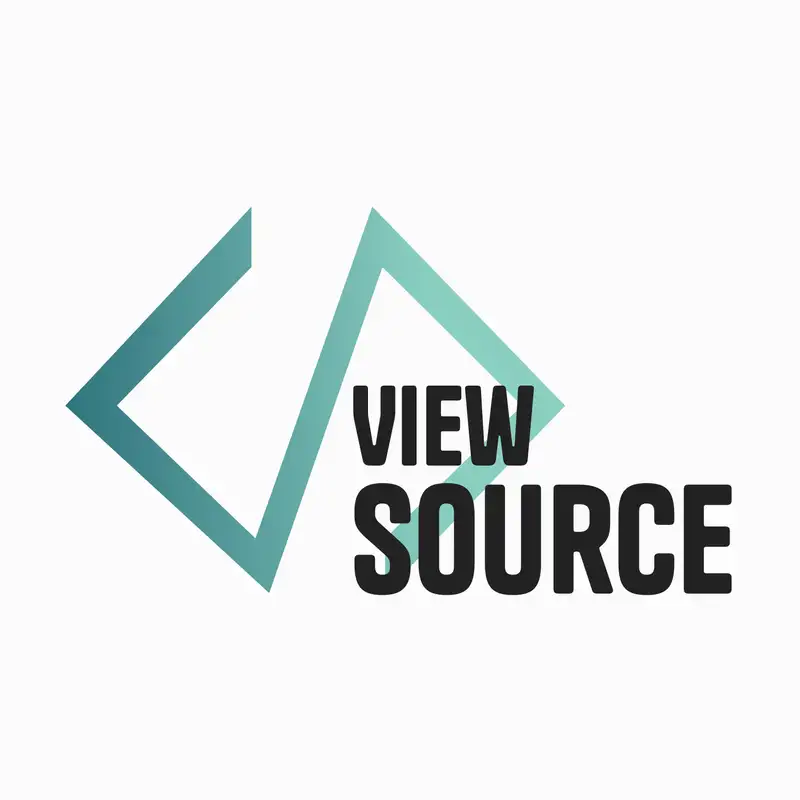