Content relationships in Laravel vs WordPress
## [00:00:00] Introduction
[00:00:00] **Brian Coords:** You are listening to viewSource, conversations around WordPress and adjacent tech with hosts, Aurooba Ahmed and me, Brian Coords.
[00:00:08] **Aurooba Ahmed:** All right, we are back in our Laravel series! Last time we talked about data and, you know, creating tables and, those sorts of things inside Laravel, and that was really neat. But what are we now doing today, Brian?
[00:00:24] **Brian Coords:** So today we're going to actually make our application interactive. We're going to let you fill out a form, a form, click submit. It saves stuff in the database. It's like web 2. 0, like the, you know, the building block of the web, the text input and a submit button that, you know, is the
[00:00:41] **Aurooba Ahmed:** Yes!
[00:00:41] **Brian Coords:** Foundation for every, everything we do online.
[00:00:44] So we're going to do that in Laravel with our application today.
[00:00:48] **Aurooba Ahmed:** And how, when you were doing it, was it like easy? Was it, you know, how did it feel?
[00:00:56] **Brian Coords:** It is super easy. It's um, I feel like I'm finally getting it, but I do remember when I first started, like you do have to kind of just learn the Laravel way to do things.
[00:01:03] But once you do, you're like. Yeah. Five lines of code and I have a form. Like it's great.
[00:01:08] **Aurooba Ahmed:** Let's, uh, take a look at this web 2. 0 version of our suggest an episode app that you've been making.
## [00:01:14] Submitting a form in to create a new suggestion
[00:01:14] **Brian Coords:** Awesome. So I'm sharing the home screen of our application. The difference now is that, um, I'm logged in as a user. We didn't spend very much time setting up user authentication.
[00:01:24] We used a package that Laravel had, so people can sign up for the site, log in and actually, you know, look at these suggestions. And so what we've added here is a form towards the bottom of the screen that says new suggestion. It has one text input and a submit button. Um, and we can just come up with our own episode suggestion, suggestion right now and submit it.
[00:01:46] And now we're interactive web 2. 0.
[00:01:50] **Aurooba Ahmed:** And I can see that the other episode suggestions that you made have, you have the ability to delete them.
[00:01:56] **Brian Coords:** Yeah. So I added a delete button to any suggestion that you personally made. So I can see on the screen. Now there's a bunch of suggestions from me that I input when I seeded the site. Then I added a new suggestion, um, today as a as a demo, and then you actually add a suggestion and on your suggestion, I can't delete it um, yet, and you also notice that I cannot vote for the suggestions yet. And we will talk about that later.
[00:02:23] **Aurooba Ahmed:** Yeah. So this is also using that Breeze UI, essentially, right? That came with that authentication package. You haven't really done anything custom in this yet.
[00:02:33] **Brian Coords:** No, I'm just, um, because breeze adds a couple of like layout, uh, template files into the project. I'm just using those. They, they have kind of components for all that stuff. And it has all the tailwind classes in the component already, which is very nice because I was not going to sit there and like, try to match their style.
[00:02:52] **Aurooba Ahmed:** Yeah. Okay. So should we try adding an episode suggestion? Actually, what
[00:02:57] **Brian Coords:** Yeah. Do you have one?
[00:02:59] **Aurooba Ahmed:** Actually what I'm gonna do on my end is I'm gonna pull up our Twitter slash X Post that we had made right before season two where we asked people for suggestions So what we can do is we can add one of those in here Okay, so there's one here from one of the people on Twitter that says hey, how do you think WordPress is evolving?
[00:03:23] And what, what the changes have been since, you know, it first began as sort of a blogging platform. It's kind of an interesting topic. So I guess the direction of WordPress,
[00:03:36] yes, so you've put it, put the whole question into the text field there,
[00:03:41] **Brian Coords:** Mm hmm.
[00:03:42] **Aurooba Ahmed:** let's do it.
[00:03:42] **Brian Coords:** And I'm submit. And you'll notice here, it's a full page reload. What it actually does is, and we'll see it go, it's like a classic PHP form that goes to a URL, processes it, sends us back to a URL with a success message that says suggestion added, um, at the top. And then I can see my suggestion is now added to the bottom of the screen, uh, with the, my name and date stamp on it.
[00:04:10] **Aurooba Ahmed:** Cool.
[00:04:10] **Brian Coords:** Do you want to see the code? Should we dig in? Yeah.
[00:04:13] **Aurooba Ahmed:** Yes. Let's look at the code.
[00:04:14] That is what I am most excited for.
## [00:04:17] Blade Templating Conditionals
[00:04:17] **Brian Coords:** Okay, so I'm going to share my screen. I think we'll start with that front end, the view. You know, we talked before about how there's that model view controller framework in Laravel. And so one thing we did is build out the view, and then we'll talk about the controller that handles this form. Um, and, and maybe talk a little bit about how you create routes and send data across forms, but I'm going to scroll down past, uh, this first area, which is basically code for the header of the site. Um, it has links. I think we kind of talked about some of these really nice blade conditionals where I can see if the route has some sort of information, I can show something only if somebody's logged out.
[00:04:56] Um, a lot of these conditionals in blade are super handy and just kind of easy to, uh, make templating happen fast.
[00:05:02] **Aurooba Ahmed:** hmm. Yeah. It looks really good. I love that it's like @guest. That's so cool. It's like so easy to understand what's going on and then an @endguest.
[00:05:13] **Brian Coords:** When I'm writing, cause I don't know what it is about, I can not get VS code to write like full PHP open and close tags sometimes, like, especially when you're in like an attribute in WordPress and you're like in the class and you're trying and you're like just auto, like expand this me and it never works.
[00:05:29] I love blade and these little, uh, just like at, and then some PHP, Oh, so nice.
[00:05:35] **Aurooba Ahmed:** It definitely removes some of that cumbersomeness. It just feels a little awkward to have to, like, open and close PHP tags. And especially in WordPress standards, we can't even use a short version of those, you know, in certain situations. So, this is really, it's, it's so easy to just read.
[00:05:54] I really appreciate that about Blade. It's just so easy and it feels so clean.
[00:05:59] **Brian Coords:** so here's the first thing I added, which is this, if session success. So when you load, um, a route, you can pass it these sort of like session, like variables, um, afterwards, and then we're going to check for it. So in this case, I passed a success message and if it's shown, then it's going to, you know, Do this div and it's going to put the success message in there.
[00:06:22] That's, um, one of those really handy things. So we saw that when we submitted the form, that success, uh, message just pops up when the page loads,
[00:06:31] **Aurooba Ahmed:** right. I mean, you know, concept of session is pretty common. PHP has that sort of baked in. WordPress, we use cookies, really, not session data, but similar, similar concept. Yep.
[00:06:44] **Brian Coords:** you know, we use a lot of also like URL parameters. You know, you do see that a lot.
[00:06:50] **Aurooba Ahmed:** Yeah. Yeah.
## [00:06:51] User roles and permission conditions
[00:06:51] **Brian Coords:** I want to start with the form that we looked at earlier, which is the add a new suggestion form.
[00:06:56] So, I'll do a kind of a quick breakdown. The first thing is we're inside that @auth, which is the opposite of @guest. Like only show this if the person is logged in. Um, and, and you can extend that with like separate permissions levels. So you can build out different permissions, like, Oh, they can add suggestions, but they can't delete.
[00:07:16] Or, you know, those sorts of things.
[00:07:18] The roles and permissions is another kind of addition on top. Like it's kind of in the core, but there's also kind of like a package that you use to maintain it. Um,
[00:07:26] **Aurooba Ahmed:** Got it.
[00:07:27] **Brian Coords:** yeah. And so for example, like say I wanted to let you and I have accounts where we could edit them, but not other people.
[00:07:32] So that would be kind of a use case for that.
[00:07:35] **Aurooba Ahmed:** Right. Cool.
[00:07:37] **Brian Coords:** Believe it or not, what we're really doing here is just, we're posting a form to a URL. Like we're, it's, it's kind of funny. Like you can do, you can definitely do single page app, no reload, all that sort of stuff, but in this case, like we're actually just submitting a form to a URL, which sometimes like it just, I don't know why it blows my mind sometimes.
[00:07:58] **Aurooba Ahmed:** Yeah, cause you, we just don't really do that much anymore. Not this, it's basic, but like, not in a bad way, I guess. Yeah.
## [00:08:08] The basics of Laravel Routes
[00:08:08] **Brian Coords:** It's just simple. Like. I've kind of gone both ways and I just sometimes on Laravel, it's just nice, it's just like, and we'll look at how that works for the routes, but essentially you make a route for everything and a route is just a URL. So just like if you were to make a form in PHP and you would have some sort of like form-handle.php file sitting on your server, it's basically the same concept.
[00:08:29] Um, the nice thing with Laravel is that routes. Actually, you can name your routes. And so, um, you can use this route function and it'll handle building out the URL, um, for you. And if you have like a nice auto complete, um, typically, let's see if it'll work. Um, I can, we go. So I can start typing and it knows it actually like scans your code and it knows all the different routes, uh, that you have, so you can, you can kind of get through them pretty quickly.
[00:08:59] **Aurooba Ahmed:** Cool, so you have a suggestions.store route, which is, I'm guessing, the way that it saves and a. k. a. stores the suggestion.
## [00:09:10] Routes and CRUD
[00:09:10] **Brian Coords:** Yeah. So we're going to have a route to store a suggestion. We're going to have a route to delete a suggestion. And eventually you could have a route to add a suggestion. And the kind of like general thing is like, store when you want to make a new one. And it has to be a POST request. Um, Then you'll often have an UPDATE if you want to update.
[00:09:28] And that has to be a PATCH request. And then you would have a DELETE request. And that would be You know, if you want to remove it, which we'll look at.
[00:09:38] **Aurooba Ahmed:** Yeah, so we're, we're looking at that very basic paradigm of any application, the CRUD, right? You know, uh, as it's called. So, um, it's nice how easy it feels like it is to create here.
[00:09:51] **Brian Coords:** Yeah. And so I used, um, Oh, and then you, and Laravel, you always pass it the like security stuff. Um,
[00:09:59] **Aurooba Ahmed:** And it just does it? Like, this, this directive will just take care of it?
[00:10:03] **Brian Coords:** yeah, it just does it like prints out all the, like input, you
[00:10:06] **Aurooba Ahmed:** Frickin magic!
[00:10:07] **Brian Coords:** Yeah, no, like naming your nonce.
[00:10:10] **Aurooba Ahmed:** Yeah! Cool.
[00:10:12] **Brian Coords:** Um, I'm also noticing that when I wrote this, so for the actual input, I'm using this. Text input component, which just came in that breeze package. Um, and I'm actually realizing I don't need to pass it. These classes, like I could delete these just to kind of clear up the screen a little bit. Um,
[00:10:29] **Aurooba Ahmed:** because they're already included in the component?
[00:10:32] **Brian Coords:** yeah, I think I had started with a regular text input and then I was like, Oh yeah, let's just do this.
## [00:10:36] Error Handling in Routes
[00:10:36] **Brian Coords:** Um, So this makes it a little clearer, but, um, yeah, so it's just a component. And what's cool is that when you do submit these forms, if you do have errors and stuff like that, it'll actually pass you back like this errors. Um, array, it'll actually pass you back the value, the person put in the first time.
[00:10:55] So let's say we submit the entire form, but it doesn't validate for some reason, you know, they had, it was more characters than we wanted or something, you know, something like that. And it kicked us back to this page with an error. It would actually. give you the value of what they had put in and all the errors underneath it.
[00:11:10] And it kind of just, all that stuff is kind of built into how form validation works.
[00:11:15] **Aurooba Ahmed:** Got it. So it's super intuitive. You come back. The error's there. The input field still has the suggestion you added, plus the error itself. Okay. That's nice.
[00:11:24] **Brian Coords:** Yeah. And so that's, you know, and then we have a, uh, a submit button and this is just another reusable component that just has some extra classes to it.
[00:11:34] **Aurooba Ahmed:** Awesome. So, overall, it looks like a straight, this is like a super short form. Like, it starts at line 72 and it ends at line 84. It's super tiny. It's a very basic form, but it's doing a bunch of different things and they're all happening through these routes.
## [00:11:51] Passing information to Routes
[00:11:51] **Brian Coords:** I'll give you an even smaller form. This is the form for deleting, um, a suggestion. So, in this case, I'm telling the route which one I want to go to, which is the destroy. I'm also passing it the suggestion object If we look at it in the front end, it'll show the actual URL and it'll have the ID number, like it'll just do all that magically and it'll write the URL the way it's supposed to be written.
[00:12:17] **Aurooba Ahmed:** Cool. Uh, that's, yeah, that's even tinier. This one is, like 9 lines or something.
[00:12:24] **Brian Coords:** Are you including the. Are you including my little conditional here?
[00:12:27] **Aurooba Ahmed:** I might have. It was a very quick count. But, uh, cool. So let's take a look at these routes then, where all this magic is kind of happening.
## [00:12:36] How to create a Laravel Route
[00:12:36] **Brian Coords:** Yeah. So in Laravel, you have this web.php and this is so you can define routes um, so we're looking at the web routes and what the way it basically works is I've added two routes here.
[00:12:52] I've added a post route where we're posting to store that new suggestion. And I've added a delete route where we're deleting that suggestion. Um, and I've wrapped them all inside of this auth middleware, which means if somebody is not logged in, they will not. Like sending data to these routes will not do anything.
[00:13:12] **Aurooba Ahmed:** So, okay, they're both routing to suggestions. Oh, and then it has, like, its own name, and that's where, that's where the store and Destroy come in.
[00:13:24] **Brian Coords:** Yeah. So, so basically here's what the route looks like as a URL, but then when I delete, I need to know which suggestion, right? So I'm passing a little parameter in there. I'm saying, I'm just saying suggestion. Like, I don't have to deal with like suggestion ID. Like I can just say. There's going to be a suggestion object here. Back in that form, when I create the route, I pass it that suggestion object. And then, you know, all the URL stuff is going to be sort of solved for me because those things kind of talk to each other. Then I'm saying, what happens when I get to that route? Like what should, like what PHP needs to run? And that's where the controller comes in.
[00:14:00] And so. You know, similar to WordPress, like you pass it, the object that you're, that has the controller. And then you pass it, what function name in the object to run.
[00:14:08] And then finally you can name your route. And that's like, gives you that nice, like. You know, ability to tell what a route does and, and reference it later.
[00:14:17] It, it kind of brings you closer to like basics. Like if you've been in WordPress and like WordPress handles routing for you, and it's not often, you know, you only hit, you only get into those like redirect rules when you hit a certain level of development so that is a big context shift of like, Oh, I got to think about my routes and what they're going to be.
[00:14:35] **Aurooba Ahmed:** Yeah. If anyone ever does any complex work with BuddyPress or with, um,
[00:14:40] **Brian Coords:** Hmm.
[00:14:40] **Aurooba Ahmed:** bbPress, yeah. The The wildness of dealing with permalinks and routing in there is, you learn a lot and you also tear out a lot of your hair.
[00:14:52] **Brian Coords:** Yeah. Oh my gosh.
[00:14:55] **Aurooba Ahmed:** Okay, cool.
[00:14:55] **Brian Coords:** You're not wrong. So the routing is basically, these are our two forms. We can send data to the post form. We can send data to the delete form. The point is we have this controller and that has the functions that are going to actually run.
## [00:15:09] Looking at the Suggestion Controller
[00:15:09] **Brian Coords:** So now let's talk about the controller. And I think we, to briefly summarize. The controller is basically like the actual PHP that's going to run when you hit that URL and it's always going to return like the view, it's going to return You know, the template that you want to see on the front end.
[00:15:25] Yeah. Now here's our two functions that we just talked about, which is the store, which is saving a new suggestion and the destroy, which is deleting a suggestion. So we'll start with the store.
[00:15:40] It's basically when you make a request to a URL, the first parameter of that function is always the request object itself. And that has like, you know, a lot of data like, um, you know, the user, any like parameters, query parameters, you sent all your input fields and stuff like that, they're all in that request object.
[00:15:58] And so. Laravel does a lot of things on that default object, like it includes a little validate function where you can say, Oh, I want rules for all the data. And if this fails, it's going to send us back with those error messages we looked at earlier.
[00:16:12] And you can do a lot of stuff with it. You can extend the object and you make them custom for everything. Um, and that's how you get that data from the form that was submitted.
[00:16:22] **Aurooba Ahmed:** Cool. When I look at this, my first, like, in my head, I'm comparing this to extending the REST API in WordPress. That's what it kind of makes me reminds me of because that's very similar to what you would do there You know, you got a request object You can validate it or not validate it if you didn't want to Then you do something with the data that was passed and there you go
[00:16:44] **Brian Coords:** Yeah. It's so nice. Not like literally typing $_REQUEST, like all of that, you know, like again, the request object, it's going to handle that security stuff we talked about too, right? Like it's all of that's in there. And if you need extras, you can extend the object, make your own version of the request and add those extra kind of checks and balances and stuff.
[00:17:06] But at the end of the day, yeah, it's like, um, give me, take data in and process it for me. And that's kind of what the REST API, like you said, kind of does in its WP request object class. You know which one I'm talking about.
[00:17:22] **Aurooba Ahmed:** Yeah, yeah for sure, okay, so when someone hits the store Uh, endpoint, essentially, route. Uh, you're creating the suggestion and attaching it to the user ID.
[00:17:35] **Brian Coords:** Yep. So we're just taking the suggestion model and we're running the create function, which will just create a new one. And we're just passing the actual suggestion that they made from the request object, um, and then we're passing the user ID of whoever sent that request. So that way we know who it is.
[00:17:53] **Aurooba Ahmed:** Yeah. So then after you've created the suggestion, then you redirect back.
[00:17:59] **Brian Coords:** Yep. And so at that point I redirect back and again, it's a named route. So I know which, you know, say we were, say each suggestion had its own URL. I could route to the URL for that suggestion, or, you know, I could route. Somewhere else, but yep, I just say, Hey, redirect back to this place. And, and that suggestions.index is just going to take us back to that homepage with all of the, the redirects in it.
[00:18:24] Um, and then with the route, there's this with function where I can actually pass some extra data back to it. And that's where we can pass a success message or an error or something like that on top of it.
## [00:18:36] Comparing Laravel Scaffolding to WordPress Features
[00:18:36] **Aurooba Ahmed:** I think that's like my reaction to everything in Laravel right now. I love how clean it is. I love how so much of it is taken care of you and you can just think about the high level logic that you care about because the base stuff that everyone cares about is already so nicely taken care of.
[00:18:50] And you can say the same thing about WordPress on a content level, you know, but this is like base application level and I really appreciate that.
[00:18:58] **Brian Coords:** Yeah. I mean, you like, if you're using custom post types in WordPress, it's very similar, you know, I'm going to use WP insert post. I'm going to, you know, you can do a lot of that stuff pretty easily and you're right. It's, it's just in a different context, you know, in that application context, you get that same sort of scaffolding.
[00:19:18] It's so nice.
[00:19:19] **Aurooba Ahmed:** Yeah, definitely, okay.
## [00:19:21] Creating the Destroy function
[00:19:21] **Brian Coords:** Then, separately, we have our destroy function, and that's the one that's going to run if we go to hit that delete form, um, and I don't actually do any validation on the request at all, which is, you know, like, there's probably a few things I would do differently, I just wanted to keep it very simple, um, but the nice thing is, if you remember in our route, we had passed the suggestion as like a parameter in the URL, so the URL probably looked like, Suggestion slash an ID number and we were hitting it with a delete request.
[00:19:53] Um, it's going to pass us that. And it's like, I don't have to say like, get the ID number, look up the post, you know, like, I don't have to like find the model and everything it's going to know. Like it's. It's a URL with a number in it, and it's gonna give me the entire object now. Like, it's gonna give me the entire suggestion, like, you know, model
[00:20:14] **Aurooba Ahmed:** it handles it for you
[00:20:14] **Brian Coords:** that ID number.
[00:20:16] **Aurooba Ahmed:** Mm hmm.
[00:20:16] **Brian Coords:** and I can just
[00:20:17] **Aurooba Ahmed:** All the data is there.
[00:20:18] **Brian Coords:** just run a delete function on it.
[00:20:20] **Aurooba Ahmed:** That's cool. And then you do the same redirect thing, it's going, it's basically the same thing, but the success message is different now. Cool. I mean, I love how nice that is.
[00:20:32] **Brian Coords:** Yeah, it's like this. I mean, it took us a long time to talk through it, but once you've done this a number of times and you're building out an app and you like, all right, I need to add a custom field to my object. You start using these same things and it just gets so much simpler to scaffold out a lot of different data, a lot of different forms.
[00:20:52] Um, you know, the fact that we connected the request to the user right here, the, where we added the user ID, that's not just storing it. Like you can actually do things like now on my user, I can say, get me all the suggestions for that user. And you don't have to write that query. It's just going to know because it knows how to combine, uh, things in the tables pretty effectively and stuff.
[00:21:15] So there's a lot of things that aren't even shown here that are kind of like. you're gonna have ready for you when you, when you're ready to add that next piece of functionality.
## [00:21:25] Comparing Routes in WordPress
[00:21:25] **Aurooba Ahmed:** Yeah. You know, when I think about this, I think about how It gives you a lot of appreciation for the kind of things that WordPress does handle for you as well. Because, you know, if you really are just making a page based website or something, doing it in Laravel, that's like almost annoying. It's overkill.
[00:21:42] Like, it's, it's like you have to do so much more manual work, whereas WordPress, it's so easy. You know, you create the pages, handling all of this for you. But sometimes I think that we can fall into this, like, way of thinking where, Oh yeah, because there's some sort of pages and stuff, I just want to use WordPress because I don't want to do a lot of this manual stuff.
[00:22:01] But when you are doing something so drastically different from creating just like a basic content filled website, doing this is actually helpful because it gives you that finer control, right? That you don't actually have in WordPress. You can, but the effort of getting to that finer control is much higher than it is in this case.
[00:22:21] **Brian Coords:** Yeah. And like, let's say this was a very large application and we wanted to start adding things like, you know, better indexes on our tables or something like that, like the fact that we're not putting everything into two tables, post and post meta, but we're actually like separating all of our different types of data into different tables and relationships, um, that helps a lot.
[00:22:39] You can. You, you know, we use for it like sets up foreign keys automatically. So if you want to delete something, uh, it might not let you, it might say like, Hey, you know, uh, you can't delete that because it's connected to this data over here. Like, so there's a lot of kind of nice database things that happen under the hood, but you does require a little more knowledge of that.
[00:22:58] Um, which I will say is my perfect segue into the next steps and what I did not do on this, which I thought I was going to do.
[00:23:05] **Aurooba Ahmed:** Yeah, okay, tell me.
## [00:23:07] When the Data Model needs to change
[00:23:07] **Brian Coords:** Okay, so to explain my mistake, I will switch over to the front end to kind of talk about, talk this through with you, um, and see if you kind of catch on to my mistake of what I did. So when I set up the suggestion model, we did like, um, we did the text of the suggestion as one piece of data, you know, we connected it to a user ID and we had date stamps as other piece of data.
[00:23:33] And then I added the number of likes, or upvotes, that the suggestion received as a piece of data. And so, can you see where I'm going with this?
[00:23:44] **Aurooba Ahmed:** Yeah, because you need to know, you need to connect the vote to a user,
[00:23:48] **Brian Coords:** Yes.
[00:23:49] **Aurooba Ahmed:** you, so that they can't, like, re vote and keep voting for the same thing again and again, because we don't, we don't want that to happen in this, in our case.
[00:23:58] **Brian Coords:** Yeah, we don't, we don't need just the number of votes. We need votes per... We need the connection between a user... And a suggestion we need to know we need that vote is, is a separate piece of data that needs to have those two pieces of information into
[00:24:14] **Aurooba Ahmed:** Yeah, it's a relation. It's a relationship. It's a related piece of information. It's not just directly tied just to the suggestion. Yeah.
[00:24:22] **Brian Coords:** Yeah. So in fact, I would not put the likes as a column in the table at all. The number of likes, what I would have instead do is set up a new table. That's just for likes. And it would have two, I like, it would have, you know, a user ID and a suggestion ID. And it would, so then I could combine those two things and then I could say, okay, pull me the number of likes that have this suggestion ID. I could make sure I don't add two likes to the same person. I could let somebody remove their like if they wanted to. Um, all sorts of things. So
[00:24:54] **Aurooba Ahmed:** Yeah,
[00:24:55] **Brian Coords:** that was a, a good example of messing up, but with Laravel, it's very easy to make changes to your database and to roll out to a migration that deletes a column and blah, blah, blah.
[00:25:06] So it will not be that hard to do.
## [00:25:09] Content Relationships in WordPress
[00:25:09] **Aurooba Ahmed:** that's good. You know, this brings up like a really good point because I think when you start building websites Bespoke, you know client related websites One of the things that we've all had to deal with is relationships between different types of content inside WordPress And there were so many I would say workaround y ways that we do that, you know Um, there's no like clear and actual proper way that you can do it on this like level the way you're talking about here in Laravel, right?
[00:25:38] Like, wasn't there was like that, that really popular plugin post to post or something like that that people used to use. Yeah. And then, or there's like what we, we, we do this a lot with like advanced custom fields, for example, right. To create these relationships. So I look at this and I think, oh, yeah,
[00:25:55] **Brian Coords:** I was gonna say, ACF has tried to push that further because they need to do like the two way relationship fields and stuff. So it's like, you know, the need is there. But, but you're, you're totally right.
[00:26:05] **Aurooba Ahmed:** It doesn't really exist. And it's. Maybe a little bit wild that it doesn't exist at a core level. And yes, there's taxonomies that we create relationships through, kind of, but that's not the same thing as making a relationship between two pieces of content that are the same type of content, maybe.
[00:26:21] Or... You know, it's just, it's interesting. And I like how in Laravel, that's something that you can do at a base level. That is an optimization. It means that you're not like doing some sort of meta queries, which, for example, in WordPress are expensive, you know, to do, um, you're able to do this in a very performant way here.
[00:26:41] Whereas in WordPress, it's a little more tricky.
[00:26:44] **Brian Coords:** I was thinking about if this was WordPress and this was a post and I needed to store that, and then I would have like post meta, right. Of an array of all the user IDs, whoever liked it. And then if I wanted to get all of the ones that I liked, I'd have to somehow do a reverse query of all the meta that has my ID in it, or I could
[00:27:04] **Aurooba Ahmed:** Or you could
[00:27:05] **Brian Coords:** usermeta,
[00:27:06] **Aurooba Ahmed:** Yeah, exactly.
[00:27:07] **Brian Coords:** Then the suggestion, when it needs to count it, it needs to go through all the user, like, or,
[00:27:12] **Aurooba Ahmed:** Yeah, or you do both. I mean, yeah.
[00:27:15] **Brian Coords:** And you're trying to keep it in sync. There's an, and then maybe you have another spot just to store the total count, like
[00:27:21] **Aurooba Ahmed:** Yes.
[00:27:22] **Brian Coords:** a lot of
[00:27:22] **Aurooba Ahmed:** a whole thing.
[00:27:23] **Brian Coords:** yeah. But then when you have, when you have much more control and access to the database and you don't also have to write all of the like CRUD operations and the, the form handlers and stuff from scratch, uh, it's so much easier to do it this way.
[00:27:38] **Aurooba Ahmed:** Yeah I think this is something that before this I hadn't really paid a thought a lot about. I mean, it's a pain point We've all experienced but you like it's you're resigned to it almost, you know And so now I'm thinking like do we have to be resigned to it? I mean, maybe we should do something about this.
[00:27:57] I don't know.
[00:27:58] **Brian Coords:** Well, I was thinking about, there is a movement for it, and I think it's, I want to say Berlin DB? Does that sound right? I think it's Berlin
[00:28:09] **Aurooba Ahmed:** Yeah
[00:28:10] **Brian Coords:** I think that's the one, and I think they're used, they use it in a lot of, um, sandhill. I feel like Sandhill, like Pippin, like, I feel like it comes out of there.
[00:28:20] And that team. And. And it's, it's trying to do like a nice like ORM abstraction for WordPress and stuff. So I want to throw that out there because I guarantee somebody will comment and say like there is this possibility, but yeah, it's not core. It's completely different, but it is, if you like that idea in WordPress, I've heard Berlin DB, but I've never tested it.
## [00:28:40] What are the next steps of the application?
[00:28:40] **Aurooba Ahmed:** Now that we have this. I mean, I think that we'll do the voting part behind the scenes a little bit, but that code will be available. But what are the next steps with this application?
[00:28:51] **Brian Coords:** So. It's sort of next steps. This technically is already deployed on the server because we've been messing around with it and stuff. But I think it's worth talking about how you, uh, how you deploy code in the Laravel world and kind of their recommended approach, um, which is Laravel Forge and, um, I think, I think it'll be kind of fun to dig into that whole world of, uh, modern
[00:29:16] **Aurooba Ahmed:** tooling
[00:29:17] **Brian Coords:** modern deployment tooling. Yes, definitely.
[00:29:22] **Aurooba Ahmed:** okay. That sounds really exciting because I know the ecosystem around Laravel and its, like, tooling in general is just very, very well done. And just, even without using it, just from the stuff I've seen online, I have always been impressed, so I'm excited to dig into it.
[00:29:37] **Brian Coords:** Yeah. And it's influencing WordPress for sure. So, uh, there's a lot to learn from it.
## [00:29:42] Conclusion
[00:29:42] **Aurooba Ahmed:** Yeah. All right. Well, I guess we'll talk about that in the next episode.
[00:29:45] Visit viewSource.fm for the show notes and if you're enjoying the show, we would love a review on iTunes or a comment on YouTube.
Creators and Guests
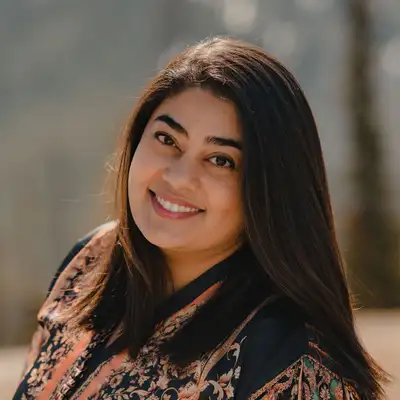
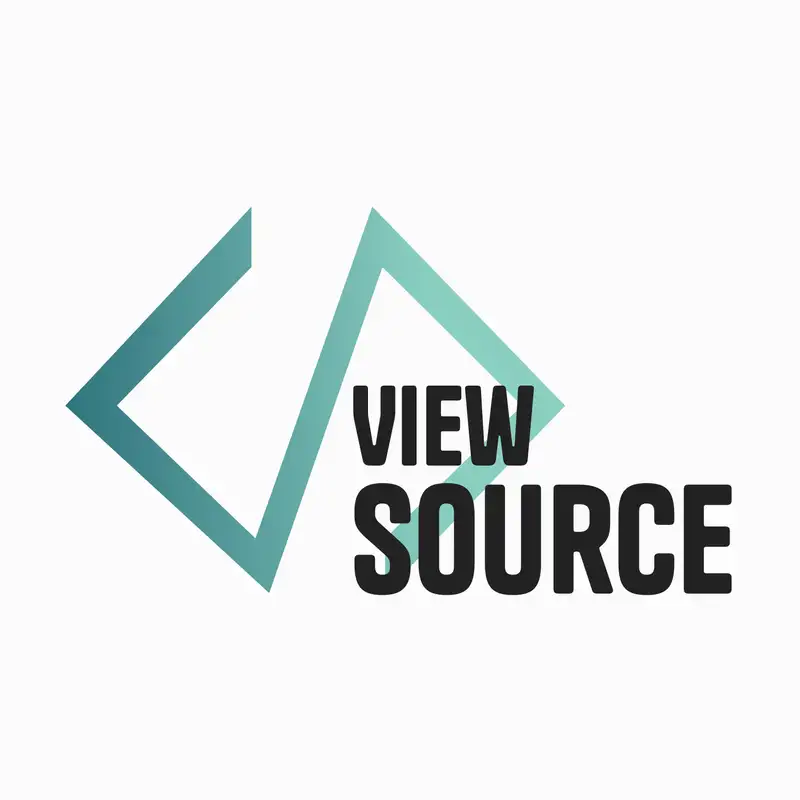