Building a Next.js Application - Part 2
**Brian Coords:** You are listening to viewSource, conversations around WordPress and adjacent tech with hosts, Aurooba Ahmed and me, Brian Coords.
## Intro Rant
**Brian Coords:** So we're back on our next JS trip journey that you're teaching us. And I'm going to recap what we've been through so far in building this application, but last episode we talked about CSS and then you posted on Twitter that I went on a rant about it. And I just want to clarify. I don't think I really went on a rant about it.
I think I've gone on some rants, but like, am I ranting about things? Is that what this podcast is now? It's just me ranting about web development issues.
**Aurooba Ahmed:** So, I think I think of the word rant a little bit differently than a lot of other people. This has come up before. I think rants are awesome. I think the rants are like, I have an opinion, I feel strongly about it, and I'm gonna tell you what it is, you know, and you do that a lot, and I enjoy it thoroughly, and that's kind of what we do with this podcast.
We teach, and we educate, but we also have strong opinions that we share with the world.
**Brian Coords:** Am I ranting and raving? Am I a ranting and raving lunatic? Like, when I think of rant, I think, like, a rant sounds like something that went on too long. I don't even remember what I was mad about. Was it dark mode or documentation or something? I don't know.
**Aurooba Ahmed:** Okay, well, I'm sorry. I didn't mean to say it like that. It was just, I think rants are great. Like, I rant all the time. I have opinions all the time.
**Brian Coords:** I got em.
**Aurooba Ahmed:** Yes, yes, it's excellent. But yeah, we talked about CSS and we talked about the decisions that we have to make whenever you're starting a Next. js application.
## Decisions and Planning
**Aurooba Ahmed:** You know, you started this episode off saying, This is where we are in the journey of building it. In fact, we have not built anything yet.
**Brian Coords:** That is true.
**Aurooba Ahmed:** And I think that's something to, that's worth observing, right? It's worth pointing out because you have so many decisions to make in any application, just regardless of whatever framework you're working with, there are certain decisions you have to make.
And if you don't think about them, just at least a little bit, you might end up. Needing to do them again or end up in like a really weird path or, you know, just having struggles that you might not have had. If you just spent a little bit of time planning, I think it's really great and fun to jump right into the code, but it's important to think about things a little bit.
And I think that's what we did last episode.
**Brian Coords:** Yeah, this morning I was working on a project where I had that realization of, I've spent a few hours on this, and I've probably written about 15 lines of code, because all I'm doing is sitting here thinking about, The database, where this should live, what's going to be the most performant, where do I want to access this data?
How do I want to save it? All this, I've spent so much time. Sort of untangling someone's code, thinking about all this stuff. And then I was like, I put in a lot of time for the tiniest commit you've ever seen, but that's what it, that's like what the work is. Sometimes, especially when you're using a framework, like.
You actually don't have to write a lot of code, but you do have to still do that high level thinking, planning, strategizing, picking your tools, all that stuff. And that's where we've been picking tools, picking the methods, picking, you know, what CSS, CSS and JS frameworks, everything. And so what is our next thing that we need to pick and what are we working on today?
**Aurooba Ahmed:** Yeah, I just want to say one more thing on that topic. We're developers and we really like code. But our job is not just to write code. Our job is to write well architected code, for the most part. And that means that sometimes your output, like you just said, you know, it may just be 15 lines of code, but that planning that you did is going to make sure the rest of the code you write, all the other lines you write, are going to be better.
So, your code output is not equal to your actual output. You know? And I think that as developers, we really need to remember that.
**Brian Coords:** Yeah. And the reason it was 15 lines of code is because the first draft of it was like 200 lines of code because there was no real thinking. Right. So then if you have a lot of codes, sometimes that's a sign that like you need to stop and you need to think.
**Aurooba Ahmed:** Totally. I agree with that.
## Today's Topic - Authentication
**Aurooba Ahmed:** But going back to what are we doing today? Let's talk about authentication. I think authentication is one of those things that when you think about how you want to do it, if you're not a hundred percent sure how to start, or if you're used to working with frameworks that just give it to you out of the box, it's like, well, why isn't this out of the box?
And, you know, it can be something that feels a little weird and nebulous. And you're like, Oh my God, this is going to be so hard. And. With WordPress, we have it out of the box. We never really have to think about it. And that's really nice. We saw with Laravel that there's a helper tool package package, I guess, that sort of gives it for you, but in Next.
js you could do it in 7 million ways and there's no built in way. So there are helpers, right? But. We talked about last time using SupaBase as our database solution and SupaBase has a built in authentication solution that works really well with Next. js. So that's what we're going to explore today because I happen to really like it and it gives you a lot of flexibility.
It comes with some helping packages that you can use or you can choose not to use. But Overall, it doesn't feel as complex as some of the other solutions you could go with, where you kind of have to create a little too much from scratch.
**Brian Coords:** So when I think of user authentication. From a high level, I'm thinking someone needs to come to your app. They need to be able to create an account or log into an existing account, which is like two different things that might happen on the front end. Then we need some sort of database that's storing like usernames and hash secrets for passwords and email addresses and all that stuff.
Then we need a sort of a state. Like throughout the case of the app, that's basically able to say like, yes, this person is logged in and or not. And maybe depending on the use case, you might also need, I'm just thinking, I'm like throwing all the things out there. You might also need roles and permissions, like different levels of users.
I don't think we will, but that's a thing that. And then finally, you need a way to communicate with the user because they need to reset their password or they need to confirm their email address, that sort of thing. So all of those pieces, when we say like user authentication, I don't know that we'll hit all of those today, but we're saying like, those are all the things that yeah, like WordPress does for you, that a package does for you.
So I'm curious to see how much of that SupaBase handles for you. How much of that, you know, we're going to have to think about as we build out the application.
**Aurooba Ahmed:** Totally. So one of the great things is authentication is a big part of SupaBase on top of just database stuff. So there's an entire section in whenever you whip up a project in SupaBase that you basically can turn on and customize a lot of stuff in. So let's take a look at that. And then we'll take a look at how you actually go about implementing it in a framework like next.
## The Supabase Admin UI
**Aurooba Ahmed:** All right, so I've spun up a project inside SupaBase, it's completely free, has a great free level, and the first thing you're going to see is that it puts the documentation front and center. It tells you how you can get started. You can go look at it, you can go Straight into the table editor and you can see all the other applications and functions that they offer like authentication and storage and edge functions and real time applications features and one of the really great things is That I'm not gonna scroll all the way down here, but it actually gives you documentation that has your own applications information filled in.
And that means that it's really much easier to understand the documentation and literally copy paste stuff into your code and have it just work because it has your credentials, anything custom from your tables, your columns, your API keys, whatever it is, it's already in there. And that's a level of personalization.
That's really cool documentation.
**Brian Coords:** I do love when documentation does that. And it like pulls from your actual applications like that. I will say, I do not like when it puts your API keys in there because you, it's, I think sets up a bad habit of people pasting, copying and pasting their API keys in, like if there's a line of example code and it's like connecting the API key, your API key should never be in that line of code.
It should be in a secret in your environment variables or something like that. Right. So that's my one complaint about that. Like they should use that. You know, approach to show like, Hey, we're putting your real key here, but we're putting it as a secret. So that way you get used to the idea that you should never have your key in your code.
**Aurooba Ahmed:** That's a very good point. And I know that in some places, they will show it to you because you might have public keys that are easy to like, they're not dangerous for people to see. And so they do expose them. But then in a lot of other places, you'll see in the documentation, they do use like environment variables, but you're right.
Like, there are places where they don't do that too, which would be better. Okay, so. One thing I don't like about this interface is the fact that there's no labels on these icons in the menu and you have to hover on them in order to be able to see them. But, you know, this is a, this is an ongoing trend.
It's everywhere. So you kind of get used to it.
**Brian Coords:** Yeah. That to me in the WordPress repo, yelling at everybody, freaking icons, no more icons, I don't know what they mean.
**Aurooba Ahmed:** I know, I know, I, I, I feel you and when you're first starting with a new UI in any kind of application, it can be a little frustrating and thankfully there's not too many things that you have to focus on at one point. So maybe that can help. But overall, like I wish there was like a text mode that you could turn on.
**Brian Coords:** If we had like the world's longest podcast, I would play a game where you, we try to guess what each of these icons goes to and see if we're right. But I don't think we have time for that.
## Authentication UI in Supabase
**Aurooba Ahmed:** so we're not going to worry about the actual storage and creating things today. We're going to look just at authentication. For authentication, it's this lock icon. And when I go into it, you'll see that this is where you would manage your users.
And so as you were creating users, you'd be able to say their display name, their email, and there's some default fields here that they just provide you with, like phone and provider and created and all that. And you can create users from here. But then, of course, you also have the ability to have them created inside your application.
And then there's a bunch of configuration settings here. So one is policies. That's something we touched on last time, where that's how you make it. So someone can only see their own data and not everyone else's data. And we'll look at that next episode. Then there's providers. Look at how many providers you can log in through. Mm hmm.
**Brian Coords:** there's a couple there. I don't even know what they are. I.
**Aurooba Ahmed:** Yeah, like cacao.
**Brian Coords:** Yeah, I mean, I'm, I know that there's, it's probably more popular than some of the other ones just based on like geography and stuff. Work OS, it's funny. Yeah, yeah, that's, so these are all like the OS, the OAuth stuff is set up if you want to do like social logins, third party logins.
Okay. Email
**Aurooba Ahmed:** Yeah. You can even set up like a custom one, you know which I actually didn't realize. So last episode I had said that if you needed to, you set up your own custom SSO, you can't use it, but turns out you kind of can.
**Brian Coords:** Okay.
**Aurooba Ahmed:** So the one we have enabled is just email. Yeah. And when you open up the email, you can see, like, how do you want this to work?
Do you want to enable it? Do you want people to have to confirm their email address when they sign up? Do you want them to have to do it again if they ever want to change it? Do you want them to be able to log in first and then change their password? They're all security measures, and you can turn them on and Then you can also even customize.
Oh, you know, what's the minimum password length? And how long until what the confirmation link in the email in your email expires. So right off the bat, really deep settings right there.
**Brian Coords:** who sends the confirmation email? Like, how does that get routed?
**Aurooba Ahmed:** So SupaBase has its own sort of free little small version, but then you can connect it to a transactional API as well.
**Brian Coords:** Okay, cool. So, if you're using like Mailgun or whatever, connect to Mailgun. It'll all go through that. Go through your, your branding and stuff if you set it up on the email templates and everything. Okay.
**Aurooba Ahmed:** Yeah, and so we'll look at that in a second too. So this is where the rate limit section is, so you can set up your own SMS or email. All of that stuff you can set up here. And then there's these email templates. So even if you connect a transactional API, you actually can set up the, the contents of those emails right here to be context aware for your application.
So right now we're looking at the confirm signup. email. And there's a heading, confirm your signup. And then you have all these variables and then you can set up your actual email right here.
**Brian Coords:** Okay. Okay.
**Aurooba Ahmed:** So I've got this already customized based on the way I set up our application that we'll take a look at, but I've set it up.
So you do have to confirm your email. So once you try to sign up, you get an email, that email was sent to you to this particular URL in the application. And then you confirm there in order to actually have your users credentials set up and you can even preview it here, which is very, very basic, but it lets you sort of, you know, see kind of how something might show up.
Just nice.
**Brian Coords:** Could you put in, do you think, like, if you use like actual full HTML, like, I don't know if you ever had to do like HTML coding of emails or use like MJML or anything like that, like, I guess it's a little code editor, so you could like put in your tables and your dumb styles, the way that HTML emails work.
Okay.
**Aurooba Ahmed:** I mean, that's why you might want to use a transactional API there because you could have a template there that's set up and this is just like the very basic little content that you want to send, you know, that's customized based on whatever the action is being taken. So there's a bunch of different email templates.
There's an invite user one, a magic link one to log yourself in with just One link click to change your email address, the confirmation for that, for the reset password. So everything you can think of in terms of authentication that would generate an email notification, you can customize that here.
**Brian Coords:** Okay. What about two factor authentication? Do they, do you know if they have support for that? Yeah.
**Aurooba Ahmed:** we're not going to be able to cover that today, but they do have, they do have support for two factor authentication, or multi
**Brian Coords:** for a project, sorry. Yes. Multi factor.
**Aurooba Ahmed:** I'm so used to saying two factor, but like, now it's like the thing is to say MFA, you know, multi factor because you can do it with more than one.
It's, there's so much stuff here.
**Brian Coords:** Yeah. I mean, it's a good, like, I'm glad that that's a more widely used thing and stuff. But so just to know, like, if you were thinking about this for a project, you could, and that was a requirement. That's the option. I. I could pretend like I understand passkeys enough to ask a question about it, but I'm not gonna lie, I don't really know what the deal is with passkeys, I understand they're pretty cool but I'm sure support will come at some point too.
**Aurooba Ahmed:** Yeah, I don't know too much about setting up 2FA either. I've never really had to do it too much. I've used, always use like helper providers or plugins that do it for you, but. It's nice. And then you have the other really important thing, which is the site URL. So here you can see the site URL that we've set up is just my local host, because this is just a local development environment that we're setting this up for, but this is how that site URL gets customized in your email templates.
**Brian Coords:** Okay.
## UI versus Config Files or CLIs
**Brian Coords:** So I've, one thing that I've kind of noticed, and I think when we get into like the database stuff too, we'll talk about this, but like SupaBase is pretty UI driven where, you know, like you're not going into, like, I mean, I'm, we will go into some config files and like set some things up, but also SupaBase just has like a nice UI for like looking at tables, setting up your tables, looking at your user stuff.
Like we don't have
**Aurooba Ahmed:** Mm hmm.
**Brian Coords:** like, just think about like WordPress when you want to set up like a table and you have to like programmatically do like a whole activation, plug in activation hook and all this stuff or whatever, like, there's just something nice about SupaBase where you're like, I can just log in and do things here and like, get that stuff done.
It's, it's super well presented. Yeah. Mm
**Aurooba Ahmed:** agree with that. And You can do things with a CLI and they have a robust CLI, but like you, sometimes you just want to click in and log in and click through a few things and just handle it, you know? But I'm just going through this documentation here and you can see there's a lot more detail and it shows you how you can, you know, verify and customize your templates even further depending on the situation you might need.
Really great documentation for the most part, especially for things that are very well established. For newer things, obviously, sometimes it takes a little while, but a lot of the main things you want to use for authentication, or just database in general, are really well explained in the docs for SupaBase, which I really, really appreciate.
**Brian Coords:** And I'm just confused because you're looking at them, and I see they have a dark mode toggle, but I see that you're looking at them in light mode. I'm just, is everything okay? I mean,
**Aurooba Ahmed:** Honestly, I dislike the dark mode settings for Supabase. I find them really hard on my eyes. So I don't like the light gray and the green combination they have in dark mode. So I prefer to always look at Supabase in light mode, but we are about to switch to my code editor, which we have in dark mode.
## Frontend Preview - What are we building?
Alright, so before we actually dive into the code, let's turn the application on and look at what we're gonna be building. So I'm going to go over to my code editor and you can already kind of see some stuff here, but I'm just going to pop open my terminal and run NPM run devs.
**Aurooba Ahmed:** And that's going to kick up my next JS application and it's telling me the URL, which is localhost 3000. So we'll just grab that, go back to our you, to our browser and go there.
**Brian Coords:** How do you remember going from project to project, whether it's going to be NPM run dev, NPM run watch, or NPM run start, because I feel like those three get used very interchangeably and I know I have a project right now that I'm working on using each one of those. Can we just like, is this a rant?
Sorry. Can we just like pick one and go with it?
**Aurooba Ahmed:** I would love if we would all just pick one and go with it. And personally, I think dev makes the most sense because you're developed, you're in development mode, right? Like you have environment variables, for example, in node that are like development or production. So let's just all stick with dev and say, dev is when things are getting watched and things are being dynamically compiled and all of that goodness and forget.
Watch, especially watch.
**Brian Coords:** Yeah, I was going to say, I prefer start, but actually, once you said that, actually, I agree. I think dev is the perfect one. I'm about to dev it up. So npm run dev. All right. What are we looking
**Aurooba Ahmed:** Yeah, all right, so we're looking at a page here that is very basic. So it all it says is this area is styled by vanilla extract, which is if we get there. Then it says get started and then there is a link to log in or to sign up. So what should we do?
**Brian Coords:** Well, I've, I've never signed up, so I need to sign up.
**Aurooba Ahmed:** Alright, I'm gonna hit sign up. And you're going to see basically the world's most simplest form. This is literally just a form that I created so that we have the most basic implementation to look at in the code. And all I'm going to do is I'm going to put in an email address. So I'm going to put my own in put in a password.
Ideally, you would have a verify password set up here as well, but for example's sakes, I don't have that. And then I'm going to hit sign up. And when I do that, it's going to redirect me to the login link and it's going to say check your email for a confirmation link. And when I go into my email, I can show you in a second what that email actually looks like.
**Brian Coords:** Yeah. And I'm curious to like password security, password strength, things like that. Like, is that going to be handled? That's probably something you need to handle in the form on your end. When you think about those sorts of things.
**Aurooba Ahmed:** Yeah, so you can confirm it and You can set up stuff in SupaBase, so you're verifying that a certain password is a certain strength and all of that, but then you all still have to come into your UI and then connect it to that API from SupaBase in order to be able to verify all of that.
## AuthUI
**Aurooba Ahmed:** There's a lot of different ways, again, that you could do that, but, and there is a UI. Package called off UI. That's who base offers that has a lot of this built in so you can actually use that to deal with it and then just style it a little bit more. The problem is, the reason we're not looking at that right now is it's not as smooth with the app router, which is the latest version of routing in next JS and you see a flash of unstyled text on the screen.
Almost always with the way it's set up right now. And I hate that. I hate unflashed. I hate unstyled stuff. I hate that flash. And so I refuse to use that even for the example.
And so this is the unstyled email. This is what SupaBase sends you. So you can see the subject is what we had set up.
Confirm your signup. And it's just coming from the SupaBase email address. It says confirm your signup. There's a link. And then it says powered by SupaBase. All of this is customizable, especially if you're connected to a transactional API. But. It gives you this really simple sort of email and you can use that to test especially.
So I'm just going to copy that link and I'll bring it back in here. And when I refresh, now you can see I'm logged in and now there is a log out button instead of a log in button. And that's it. Now you are logged in.
**Brian Coords:** And now that's persistent. That's
**Aurooba Ahmed:** Yes. If I go home. It's yep. Now I've gone back to home and it's still persistent. And in SupaBase is all cookie base. So it's setting up a local first party cookie in your browser that helps your session persist from page to page.
**Brian Coords:** Okay. And so in SupaBase, we would now see your user on that table of like users that we, we added.
**Aurooba Ahmed:** That's right. So now if I go to SupaBase and I go to the users table here, you can see I have an email address. I signed up using an email. This is when I last, like this is when I set it up. This is when I last signed in. And then there's a UID as well.
**Brian Coords:** Nice.
**Aurooba Ahmed:** I can delete it. I can send a pass password recovery link.
There's a lot of stuff that you can do right from SupaBase as well. And it's just very simple if you think about it.
**Brian Coords:** yeah. I mean, cause I also, you think about this, like in WordPress, you can go into like the admin interface and you can do all those things, but like, you're not going to have that in your application unless you build it. So the fact that SupaBase is going to give you that sort of stuff of like, Oh, this person needs a password reset.
This person needs this. It's like, you know, you don't have to build the UI to deal with all of that. That's pretty nice.
**Aurooba Ahmed:** Yeah. It means that you can get up and running really fast because remember, I'm using the hosted version right now, but this is included when you self host SupaBase as well. So this
**Brian Coords:** Oh yeah.
**Aurooba Ahmed:** whole thing, it's all there.
**Brian Coords:** Yeah. I was kind of curious, like how. All of that works with the self hosted like, so self hosted, you have access to all the same features. It's not like the hosted version has like just some extra stuff that's just, you know, only possible
**Aurooba Ahmed:** things the hosted version has, like edge functions are a hosted feature. And if you want to do real time with self hosted, I think there's a little extra set up there, but authentication and tables and the other stuff is all like part of the open project as well.
**Brian Coords:** Okay. So, I'm really curious what it looks like, like how much work this was to set up.
## Our package.json and Cookies
**Aurooba Ahmed:** Yeah, let's take a look at that. In Next. js, let's take a look at our package. json file first, because I know you were really curious about that. and you're going to see a few things extra here that we don't need because I tried off UI, but really what we're using is SupaBase SSR, SupaBase, the JavaScript client, and we need the cookies dot dash next package as well so that we can access cookies either on the server side or on the client side to be able to authenticate and check if someone is logged in.
Those are the three things that we're dealing with today.
**Brian Coords:** Yeah, another topic that I could go off on these cookies, like I was just importing, like they were just like import, like whatever the basic JS like cookies packages, because you don't want to write that same functions over and over. Like, you know, it's cookies are so funny because they've been around for so long and you.
There's not like just a nice basic UI or like a face, but then yeah, in the next application, because you have that server and client side, everything gets a little crazier with things like that. I remember that being a kind of struggling point for me to grasp like, Oh wait, I don't have cookies the same way.
You got to pass them through the headers of the requests. Like there's all this sort of stuff. So it's nice to know that they do have a package to kind of abstract some of that stuff for you.
**Aurooba Ahmed:** Yeah, it helps because in Next. js, you have your client side, you have your server side, and then you have your middleware. And the way cookies get handled in all those three places is slightly different, because of the fact that middleware is kind of on the server, but it, is interacting with the client.
So it's kind of in the middle where area and it happens a little differently than the server. You have to fully pass it because it has no access whatsoever to your browser and the client. It's totally different because of the fact that it has all the access so you don't have to send it anything. It just has all of that available.
It's something that took me a little while to wrap my head around too. I think it ever is the case for everyone, but I feel like by this point I have a pretty good handle on it. Thankfully. So those are the three things that we added to our package. json. In order to integrate with SupaBase, first of all, we need to set up our environment variables.
And we really just need two, which is the SupaBase URL and then the anonymous key that they give you. You're looking at my sample file but of course I have a env. local file where I actually have these API credentials filled out.
## Folder Structure
**Aurooba Ahmed:** And then we're going to take a look at, first of all, the setup. So in Next.
js, because we're using the latest version, there's an app router and everything's a little bit different app router because now you can create components that are server side or they are client side. And the way we have it set up right now, we're actually doing something, I want to make it a little closer to Laravel.
So we're using mostly server side components, which is why you saw that the page would refresh when we hit a button instead of things just dynamically changing.
**Brian Coords:** So under the app folder, you have auth, error, log in and sign up. Are those the base of like a route potentially? Like
**Aurooba Ahmed:** no, each of these is a route.
**Brian Coords:** Yeah. So like sign up is a route and then inside of there is going to be everything that. Okay.
**Aurooba Ahmed:** Yeah, so inside SignUp, if you want to have a page, then you can add a page. You could also add a route, which is API that you want someone to hit. So, you know, if you had an API route called, in this case we have an API route called Auth, and then inside it we have a confirm and an error.
And the confirm actually just has a route. ts file in it, because it's just doing a GET request, and there's no page to show there. But with every single route that you can create, there's a different types of files you can create in there that do different things. If you want it to show a page, you have to have a page.
tsx if you're using TypeScript file in there, or else it won't show anything. So there's some very specific file conventions just like Laravel has when you use the app router. And that's the reason I like it because it gives you a very specific structure that has to be the same no matter what project you're in.
And that makes getting started and jumping into new projects easier and better.
**Brian Coords:** So, when somebody logs in, are you, and you submit the form, data, you could submit it to a route in, like you would submit it to a route in your app or something like that, and that route Is going to handle all of that kind of like in Laravel, like you can build a route, that's a get request and displays data, but you can also have, you can post to it so that you can like submit a form and come back to that page and that sort of thing.
So it's kind of, I like, I, I think there was like a world where everything was. So like the Ajax era of like submitting little like things and stuff like that or whatever, and then you kind of come back to like the basics of like, no, a URL that you can route, like, and submit forms, that sort of stuff, even if it is running on the server or anything like all that aside, it's kind of nice to make sure like, Hey, this is a, we're going to have a form and we're going to submit it and this is where it's going to go.
You know?
## Setting up a Supabase Client
**Aurooba Ahmed:** Yeah, I agree. So, in order to start doing that with SupaBase here, we first need to set up a SupaBase client in our code. And I've set that up in a library folder. And There's a few different things here because we talked about how there's the middleware, there's the client side, and the server side. We need different kinds of clients that interact with SupaBase depending on what use case or what context we are using SupaBase in.
Okay, so from our SSL library, we're grabbing the create server client component and then we're passing it our environment variables and also telling it how we want to handle any cookies for user authentication.
**Aurooba Ahmed:** And then we're able to use this, this single instance throughout our application whenever we need to interact with base on the server side. Does that make sense?
**Brian Coords:** Mm hmm. Yeah. Okay.
**Aurooba Ahmed:** and then there's one for the client side as well, in which you don't have to do anything about cookies, because everyone knows how to deal with cookies in a browser, and so does SupaBase.
And, but there, the client is called Create Browser Client. And then you have sort of an actions area, which is sort of middleware. It's kind of server side. But a little smaller and it mostly looks the same as server side, but sometimes there's extra settings that you can pass in middleware that you could also put it in here for simplicity sake.
I have that file set up, but right now we will use it differently later, but right now it's exactly the same as a server. client.
**Brian Coords:** Okay. And so in your application, you're going to use these throughout the, anytime we need to like authenticate the user, make sure the user's logged in, determine
**Aurooba Ahmed:** We're going to use this any single time we want to deal with SupaBase at all. So not just for authentication, but later as well when we are creating, you know, user information, showing all of that stuff. Everything has to go through the SupaBase client. And instead of making a new client every single time you need it, you just make one.
globally and you set it up inside a function because then there's just one instance of it. Otherwise you get multiple clients getting made every single time there's a render and that gets used everywhere until you shut down your application or you go away.
**Brian Coords:** Okay. So what does this look like? Like in context of a component or a page?
**Aurooba Ahmed:** Yeah, let's take a look. So let's take a look at the signup page. So the signup page looks like this and here we Remember, we're in a server side client component, server side component right now, so this is not client side. So, we're using our cookies from the headers area, and then we just import our create client that we, the component we did created for SupaBased client, and then we pass it the cookies that we have, so that in case someone already has a persisting session, we're able to use that information, and then we Use that client, call it SupaBase, and then call auth.
getUser. And that's how you can check if someone is signed in or whatever user information you have for them right now.
**Brian Coords:** And we're using like, like async functions to make sure that like, we kind of, we need like, like, we kind of need to get that information. Right. Like we,
**Aurooba Ahmed:** Yes.
**Brian Coords:** and that, that's another. Place where I remember really struggling with the idea of wanting to use asynchronous functions to make sure you have the correct data and make sure that you're waiting for it and all that sort of stuff.
But also I think there was issues with like, if you're on the client side, that sort of stuff. But really you want all this to happen at that server level first, right? Before you're really inside of your application.
**Aurooba Ahmed:** Exactly. And so instead of having that thing where the page loads and then things change because now it's detecting after the load that someone has signed in, let's just handle it on the server and then say, Oh, this person is signed in. So send the correct information. That's something that in Next.
js 12, for example, was not a sort of a regular pattern. And it was a harder thing to do there. But in Next. js 14, which is what we're in right now, that separation we're Makes this a lot more familiar to people coming from, say, Laravel and other applications like that, but also just makes for a better user experience.
You know, like, what's the point of all of this if we don't create a good user experience in the browser? You know what I mean?
**Brian Coords:** Yeah. And I was thinking like, there's such a fundamental difference between a logged out and logged in visitor using your website that like. That really is something that should be determined well before, you know, you get into like the, the, the user's browser, like running client side JavaScript and stuff.
So,
**Aurooba Ahmed:** Yeah, I agree. I think one of the things that as we keep going forward in the world of web development, Is really getting really important is personalization, which is, by the way, one of the core components of good user experience that was written in this old book that I can't remember the name of right now, a long, long time ago.
Personalization is really important. And so instead of loading something and then personalizing it, we should just send something personalized, right? And that means in this case, The logged in view, if someone is logged in. So here you can see, I just have a basic form and all I'm doing is I'm checking from that authentication.
If I have a user. So if I, if I do have a user and that user is, you know, correlated with my user database in SupaBase, then. I will just tell them they're logged in. Otherwise, I'm going to give them a form that let's ask for their email address and their password. And when they hit that signup button, it's going to have a form action, which is special, that is passed a signup function, which is actually going to do that magic.
**Brian Coords:** where did you get signup from? And
**Aurooba Ahmed:** which is part of this route. And that's what we're going to take a look at next. So if I look, go go into that actions. ts file, I've set up a signup function.
## Submitting Forms to Supabase
**Aurooba Ahmed:** And the really important thing is all actions in this sort of structure. only interact with forms. So if you want a server action to happen, it must be through form data that gets passed to it.
So it only works with forms. And what I'm doing here is, again, I'm creating that SupaBase client here, but it's the same client. So I'm just referring to that same instance that we've already created. I'm grabbing the form information we have, so the email and the password, and then I'm sending it to SupaBase with their signup command.
And If it worked, then I redirect them to the login page, and if it didn't work, then I redirect them to the error page.
**Brian Coords:** so the process of setting the cookie and all that will happen in that SupaBase. Client that you've created, it's going to handle all that process. So like, you don't need to, like, once you've called that, it's, it's going to take care of everything else. And you can just send them straight to the login and
**Aurooba Ahmed:** so,
**Brian Coords:** is this idea of like you have a page component and you have these actions and they're not just functions at the top of your page component.
Like it's a lot of times you just think, okay, I have my component and I have all this space between, you know, the opening of my component and my return statement and I'm going to cram everything in there. But like the convention of actions. ts as a separate file, is it just something you did or is that something that's going to.
That next JS is going to recognize this file in a specific way. Does that make sense? Does my question make sense? Like, are you following a specific convention that adds functionality by calling it actions at TS?
**Aurooba Ahmed:** Correct, correct, exactly. So if you want a server action in a different file, you have to have that in actions. ts. You can create inline actions, but A lot of times, you might have more than one action. And so the same thing that you just said, it's nice to put that in a separate file and keep your actual page uncluttered and just easier to read to see what is actually happening in terms of the page content.
**Brian Coords:** So you are using browser validation, right? Like I see you have required and type equals email and stuff. So like there shouldn't be, but like, if you did want to add like some sort of validation stuff to this, like you could do that in this setup, right? Like,
**Aurooba Ahmed:** That could also go into your actions file as sort of a validation function and use that and gets passed in here. In however way you want to validate it, validate it here against the information inside SupaBase and the functions they provide you with. Yeah, so it's not exactly co location, but it's still co location because it's all in the same folder.
You know, it's in that
**Brian Coords:** Mm hmm. Yeah.
**Aurooba Ahmed:** it's actions and page. And I, I just really like that that is now a specified Next. js convention that you can always rely on no matter what project you're in.
**Brian Coords:** I love a folder with files that are all named a specific way, small files with specific, meaningful names. Whether you're building blocks in WordPress or you know, an application in next, or even like Angular is a lot like that too. So I just, it's a nice little, like, I just, I love consistency.
**Aurooba Ahmed:** I agree with that.
## Session Data and Server-side Console
**Aurooba Ahmed:** So, let me show you what that session data actually looks like. So, if I go to our home page and I console that. Out, it's going to show up in our terminal. And if I refresh this, you can see that it's giving me this user and it's giving me my ID, if I'm authenticated or not, what my email address is, all, a lot of that information that you saw in SupaBase in the database there, you can see it here in your code as well.
**Brian Coords:** Okay, so, and this is like one of those things that trick me up every time, but like paying real close attention to where you're console logging, like server versus client, right? If you're going to console on the, in like the actual terminal of where you're running, like, cause you know, in your terminal, you're running like a fake little server, right?
Like a little local server. For your app. So when you do things in the server, they come out here so you have access to all this information when the, so like you could very easily use that user object to say like you know, up in the corner, like how do you put the person's name right. And, and do that kind of stuff.
**Aurooba Ahmed:** Yes. And for context for everyone listening, it took me four tries because I kept forgetting where this would get console logged out. Would it be in the browser or would it be at the terminal? What page did I need it to do it to? So it's even though I'm so familiar with this application that I wrote, I still forget.
So it's okay if you forget. It is very normal. It happens all the time.
**Brian Coords:** Yeah. The things people don't see when you're videoing code tutorials. Yeah. The
**Aurooba Ahmed:** all the things that you set up on. Yep. Yep. 100%. 100%. So that is honestly the crux of how we're logging in. But when I hit that confirm, there is that extra confirmation that we need to set up. And that's where that auth confirm file is. So when I got that email and I hit that confirm link, There's this route which has no, you know, visible UI that it is a get request that we're running in order to actually confirm this user and then officially let them have access to our application.
And it's not that crazy, but it's basically taking a hash. Cookie hash and it's checking it from your application in SupaBase. So that database checking that it is actually correct and then saying, yep, we got that. We're cool. This person has actually clicked it, clicked on a link with this special sort of, you know, hashed password thing, and now they are confirmed.
And then it changes their status in SupaBase itself. And that's what this file does.
## Scaffolds and Boilerplates
**Brian Coords:** And, did you, did you have to write this? And, was it easy to find a good example of this? When you, if you wrote it?
**Aurooba Ahmed:** I didn't write this. I edited it just a little bit to decide where someone should get redirected here on line 16 once they've been confirmed. But the rest of this, I just copy pasted it from the SupaBase documentation. And it works like magic.
**Brian Coords:** Yeah, that's an interesting thing, I think, too, about a lot of, like, JavaScript API integrations when you do these sorts of things. Like, it's not quite the same as off the shelf where a plugin or even a package in Laravel, which will copy a bunch of files into your thing. Like, there's You know, anybody who's ever set up like a Stripe connection or something like that, it is a lot more time going into the docs, copy and pasting, figuring out why it didn't work, getting it, you know, up and running and stuff.
So it's, it's definitely a comfort level with that approach to integrations that you have to have when you're in like the Java world, I feel like.
## Flexibility vs Effort
**Aurooba Ahmed:** Yeah, do you want to do your integrations in code or do you want to do your integrations in a UI? You know, I think. WordPress is there's lots of code in it, but ultimately, it has this administration panel that's trying to make it a little more no code, you know, before the term no code even existed, but the JavaScript world is very much about, yeah, pop into your code editor and do a thing, you know, and It's still easy, a lot of the times, but it's a different kind of easy than using a visual UI.
And maybe that's why I really like SupaBase, because I really like that, you know, it does have some kind of UI that I can do certain things in, instead of having to do everything in UI, or in code only. You know?
**Brian Coords:** Yeah. I mean, it's also like. The flexibility of like, give me, give me enough UI. That's basically like a table, like, you know, the idea that every website is just a spreadsheet. Like we, I really just want to see the spreadsheet and just, you know, whether it's the users, whether it's the database and stuff, show it to me.
Looks like a spreadsheet. Let me do a few things in there and that's perfect. Whereas, yeah, sometimes with WordPress, like it is great that you get all that stuff done, but once you do want to personalize or customize it and you're looking in something, you're like hoping that the person wrote their plug in the right way and all this sort of stuff.
That's the tradeoff that you get. So it depends on like the situation and how happy you are with something off the shelf that's gonna like get you 90 percent there versus in this situation where you're probably working on a project where somebody's budget is higher, their expectations are higher.
The end result is much more, I mean, we keep saying personalized, but I do think that's like the driving force between. Behind word, like headless WordPress or JavaScript in general, like, or JavaScript, like apps in general, it's like that level of deep personalization and seeing your own kind of view of data and stuff in front of you.
That's like the, the kind of point of this. So that's why there's the extra work is because of that extra flexibility.
**Aurooba Ahmed:** Yeah. And I think you brought up headless, you know, that's something I've been thinking about kind of deeply and why people might want to do that. And, and I think one of the reasons is that in the world of JavaScript, for example. You have to make every decision yourself and sometimes that's not a bad thing.
That can be empowering because you're able to reduce bloat and only have the things that you want. But the really great thing about WordPress is that you can get a lot of the way there and then just add a little customization. The trade off is you might have things in there that you don't want. And then you have to fight them sometimes or, you know, ignore them or do something about them.
**Brian Coords:** that's why we've had projects where. You look at it afterwards and say, we built like a crazy, complicated application. And for that client, honestly, setting up a WordPress with some gravity forms to get them to an MVP, to get them to paying customers, to get them to a place where they have the budget to then go and build the giant complicated thing, because now you have the budget, you have the customers, you have like
**Aurooba Ahmed:** The validation,
**Brian Coords:** The validation, yeah. Versus trying to do that first and put in all of your crazy ideas and everything and never even get to finish it because you just can't, you know, you don't have the budget for it or the time or, you know, whatever. So it's definitely
**Aurooba Ahmed:** or you waited because it turns out your premise, your idea was not good, you know, so now you wait all this custom stuff and you have nothing to show for it.
**Brian Coords:** Yeah. And you could have honestly found that out with some gravity forms, typically, like, like if everything is a spreadsheet, everything is just a form on the internet. And like, at the end of the day, you know, that's, that's the place to start. And then when you get to the really complicated stuff where you want to show dynamic data based on the person's location, based on what they've looked at before, based on their browsing history, based on who they're connected with in the app, all that sort of stuff.
Then you use this and then you get like. This great experience of, of personalization, but with a little work behind it, just a little bit of work. Yeah.
**Aurooba Ahmed:** bit. But yeah, you know, that is how you do authentication using SupaBase inside Next. js. Is it a bunch of code? Yes, I would say it was like a few hundred lines of code in total to set up the login page, to sign a page, and then to set up that confirmation route.
But a lot of that is given to you in the documentation, so it's not like you're writing it all from scratch yourself. And the other parts just make, make it so that you Have a lot of empowerment, and you can set it up exactly how you want, or you can use UIs that people have already built. There's more than one UI, you know, that you can take for a login, set up with authentication and validation and the reset password and all of those things are in there.
Plug it in, slot SupaBase into it, and it will work. I've tried like two or three when I was testing this out, and it does work very quickly. And then, those few hundred lines go down to like ten lines of code. But, I wanted to show the very
**Brian Coords:** let's say the expense of the thousands of lines of somebody else's code that you brought in that you might actually not need. So, you know, do you want to save yourself some time and get a UI, but now they put in like 50 different widgets that you never really needed or something?
Yeah.
**Aurooba Ahmed:** Exactly. There's always a trade off, right? You either have something like WordPress where there's lots of stuff that you just can't get rid of, or in JS you can bring in other people's packages, but same thing, there's lots of stuff that you can't get rid of. I just think that it's a lot easier in JavaScript though, to say No, I'm not going to use any package and I'm just going to use these raw methods that someone has given me and just build my own thing.
That's a lot harder to do in say a CMS like WordPress.
**Brian Coords:** So what are we doing next for Next. js episode 3. 0, not 2. 5.
## Next episode
**Aurooba Ahmed:** Yeah, so next episode, we are going to take a look at using this user authentication and actually setting up a account area for someone to set up their own WP audit checklist using SupaBase and its rows. So we're going to take everything we learned today and all the decisions that we talked about making in the last episode and put it together into an actual application that allows someone to log in and then customize and save their own checklists using SupaBase.
Our backend database.
**Brian Coords:** All right. I'm excited. I'm excited to see what this looks like. I'm actually excited for people to use it too. Once it's like out there, because I think, I think it's just going to be a handy, a handy tool.
**Aurooba Ahmed:** Yeah, so I'll see you next episode.
**Brian Coords:** Alright, see you then.
**Aurooba Ahmed:** Visit viewSource.fm for the show notes and if you're enjoying the show, we would love a review on iTunes or a comment on YouTube.
Creators and Guests
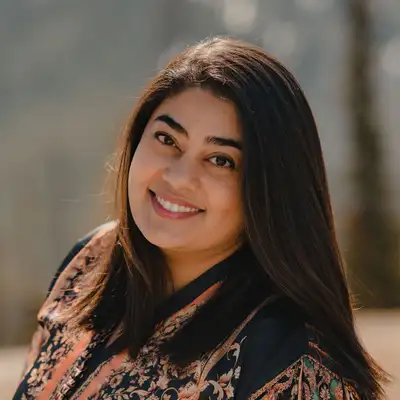
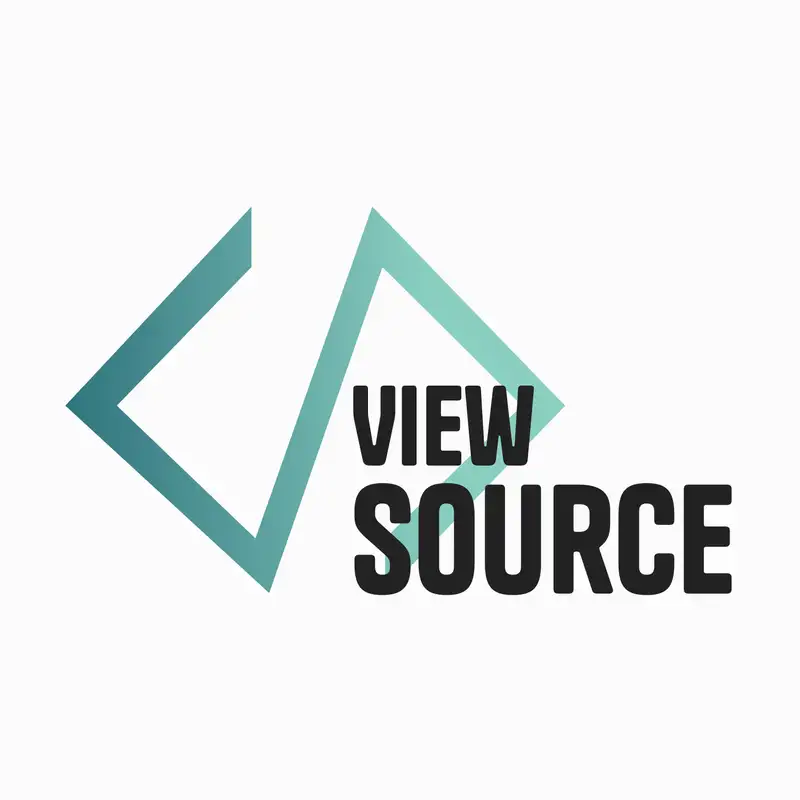