Reusable Components in React
**Aurooba Ahmed:** You are listening to viewSource, a conversation around tech, web development, and WordPress with hosts Aurooba Ahmed, that's me and Brian Coords.
**Brian Coords:** Aurooba, do you ever dream about code or web
**Aurooba Ahmed:** development?
Yes, yes. I dreamed about it. Yes. Oh yeah, definitely anxiety, but also sometimes when I'm really excited about an idea or I just learned something new, I will end up having weird dreams about it. You?
**Brian Coords:** Like, yeah, but like there's never a computer involved, if that makes sense. It's like I know that I'm dealing with , Coding concepts, but they're so abstracted that there's no like keyboard and screen. Does that make sense?
**Aurooba Ahmed:** Yes. This is maybe gonna sound really, really weird, but usually I'm like Tony, Tony in Ironman. Doing it with my hands and just manipulating the dom just like this or doing stuff. That's how it is in my head.
**Brian Coords:** Okay. I think mine is like, A more Willy Wonka version where I'm maybe like at a giant espresso machine and there's like knobs and levers and like steam coming out or something, and I'm trying to make like a smoothie, but I'm doing it with components or something. Like, it's just, it doesn't make sense.
I don't...
point is-
**Aurooba Ahmed:** Dreams are weird.
**Brian Coords:** dream.
Yeah, dreams are definitely weird and. I think the point is that sometimes there's foundational structures of web development that like get into your brain at like an abstract level, and one of those is components, which is our topic of today, and the idea of making components.
**Aurooba Ahmed:** Yeah, I think, you know, we're gonna be talking about React, but honestly, the concept of components is so old. I think it's as old, almost as old as like the web. You know, like even HTML is very componenty. I mean, you don't create a brand new element for every single thing you wanna create. You're using the same like diviv and paragraph, and they all have, they're certain settings that they apply to your content that you've wrapped them in.
Um, it's, everything is very component-ified and certain frameworks on top of the web often lend themselves to being more easily component-able than others.
**Brian Coords:** It's funny that you said that because like you said, we don't make our own like HTML components, but sometimes in a react that feels like exactly what you are doing. Cuz like a React component feels like a little like HTML guy, you know, because he is got his little brackets and stuff? And so it almost is like: I'm making my own HTML thing and it's gonna do all the things I want it to do and it's gonna be like my own version of it.
Like that's what it feels like sometimes when you start breaking things and reacting to like your own components.
**Aurooba Ahmed:** Yeah, and I mean, to be fair, web components. They do exist even though they're not as mainstream yet, which is a way for us to create actual HTML custom components ourselves. So that is coming there. There is gonna be a time when we're gonna doing, be doing a lot more of that than we are able to really do so easily right now.
**Brian Coords:** Which is a good reason to get into this kind of like React component mindset because this, this really will be like, you know, a potential forward way of the web is, is thinking in terms of like reusable components, web components, things like that. So,
**Aurooba Ahmed:** Yeah, definitely.
**Brian Coords:** With that, do you wanna give us the recap of where, where we've been and then where we're going?
**Aurooba Ahmed:** Yeah, so although you can listen to this episode on its own and get a lot out of it, this is part of an ongoing series we're doing where we start from scratch, uh, and work with how React is added inside WordPress. And then we're slowly building our way up into building an accordion block eventually within the WordPress block editor.
What we've done so far is create a accordion first, inaccessible, then accessible, inside just pure React. And working on it in the front end of a little WordPress website. And today we're gonna take it a little step further and bring in that concept of reusable components that we were just talking about.
**Brian Coords:** Okay, so I'm sharing my screen and do you notice anything a little bit different here from the last episode?
**Aurooba Ahmed:** Yes. Now I see a proper accordion and not just one accordion section as we talked about last time. So I see three "hello world" sections with the little triangle to indicate that you can open these three, three things. And on the side I can see you have the dev tools open.
**Brian Coords:** Yep. So I have the Chrome extension for React installed and in my dev tools it adds like a couple new tabs. Um, and the one we're gonna look at today is the components tab, which is just an amazing- it's like the list view in Gutenberg, like it's just the breakdown of all the components and how they're nested and everything.
I love it.
**Aurooba Ahmed:** I love it too. And actually if you ever get curious and wanna open that, that up in the block editor, it's wild. It's wild how much stuff is going on in there.
**Brian Coords:** Yeah, you, the, you would never be able to like navigate to anything because there's so many components in the, the block editor itself. But there is, um, what I love is like when you highlight over a component, it highlights it. Here, it's very similar to like the HTML inspector where you can like pick something and then like focus on it in here to see, um, to see all about it.
**Aurooba Ahmed:** totally.
**Brian Coords:** This was our app in the previous episode. It had everything in it, but now we have three separate components, um, one for each accordion item. And what's great here is, um, we see our props, which is something we're gonna get into today, which is like, what are all the things that are being stored kind of as part of this component.
Um, so there's a little object that has like the accordion, kind of like markup content in it. Um, some additional val like variables that we'll kind of go over, functions, things like that. And, If I click on one of these accordion headers and it expands, ooh, look at
**Aurooba Ahmed:** Then all of a sudden, a new accordion panel shows up underneath the accordion header. Cool.
**Brian Coords:** So there's our new component.
It's got, um, some additional props that we've passed down, like the content of what's inside of it, an ID and stuff like that. So as I do it, click it, it expands you. Did you see that?
**Aurooba Ahmed:** this. Yeah, that's that's really cool. Yeah, I, I really like that. So basically when you open one accordion section, the other, any other ones that was open, they als they close. So you have a relationship going on.
**Brian Coords:** Yeah. So when we dig into the code, um, We're gonna look at sort of why, how we broke this part into different pieces, what the reasoning for, for it is. Um, and then we're gonna look at how are all of these props being defined? How are we passing them down from, you know, the App, which has, um, actually no props.
And then all the way down to the individual accordion items.
**Aurooba Ahmed:** But if you go and click on App again, something that's really interesting to see is that it does tell you what hooks are being used, and you can see that it has one state hook. So the fact that we see state here leads me to believe that you're managing the relationship between the different accordion sections in your global App.
Am I right?
**Brian Coords:** Yeah, I mean, think about how you would've built this in like the jQuery days where you click on one accordion and then you like jQuery up the parents to find it and then like close all the other ones. Like every, we've all done it. We've all done that kind of like jQuery, parent, child, sibling selector, like tree.
Um, So, no, the, everything kind of starts in App and then we send things kind of down the tree, uh, to the places that need it. But it's, it's up in the App where the functionality happens.
**Aurooba Ahmed:** Which is a fairly core piece of React knowledge to have. Where React is, you know, everything is one way. You start at the top and you can pass things down. There are exceptions where you can pass things up, but that is basically called an escape hatch, and it's something that you are supposed to only very rarely do, so it makes sense that you have it right at the top there.
**Brian Coords:** Are you ready to look at the code for these, uh, these components and see how they work?
**Aurooba Ahmed:** Yeah, let's do.
**Brian Coords:** Okay, so I've got my code editor open and we're looking at our App.Js file. And as you can see, we've got a few, uh, new fancy things going on here. Should we go through them line by line?
**Aurooba Ahmed:** Yes, let's.
**Brian Coords:** Okay, so the one thing that changed here is that we're importing a new component called AccordionItem. So you'll see I have tabs open for accordion item, accordion panel, accordion header. That's, you know, literally what we were just looking at on the front end. Those different components, the item, and then inside of the item, the panel and the header.
Um, so we're just importing it here and we'll kind of get down to it.
**Aurooba Ahmed:** Okay. Next, I'm seeing you have an object that where you have all of your accordion data, so these are, because this is not a block yet, you need some way to actually add information to the accordion panels, right?
**Brian Coords:** Yeah, and so I like to think in terms of like in the future. When we turn this into, say, an editable block or something, or even if it was like, if you were kind of going old school WordPress in like ACF fields, if that like mental model fits for you better, um, this is like what is the structure of the data that I'm gonna get?
Um, whether it's through, you know, some sort of, uh, data layer, fancy function or it's just like some localized data or something. But like, essentially like these, these are the accordions as I'm gonna hopefully get them, uh, when we actually hook this up to like a nice user interface on the back.
**Aurooba Ahmed:** Right. That makes sense. And so you have ID, heading, and content for each of the objects. And I should correct myself because I initially said this is a object, but it's actually an array of objects.
**Brian Coords:** Yeah, I wasn't gonna call you out on it. I was gonna let it go, but
**Aurooba Ahmed:** Well, I caught myself. Thank you very much.
**Brian Coords:** of objects.
**Aurooba Ahmed:** Yeah, cool.
**Brian Coords:** Hey. And down here we have, , a new state. So this is actually a bit of a difference from last time where it's pretty similar in that we're pulling in state. , but we're actually the only thing we're tracking in state this time is like, which accordion is open, , rather than whether the individual accordion is open.
, but we're going to save all of this stuff for the next video and, and deal with that later.
**Aurooba Ahmed:** Yeah, the state and the change logic, it deserves its own little episode cuz there's, on the surface it doesn't seem like a lot because it's like 1, 2, 3, 4 lines of code really. But those four lines of code do a lot of interesting things behind the scenes. Yeah, I think that makes sense.
**Brian Coords:** and I'm not, yeah, I, I have a lot to discuss about it, let's just say that. Hmm. Okay.
**Aurooba Ahmed:** Definitely.
**Brian Coords:** And finally, , our actual component's return function. So all of our components are gonna return basically some HTML, , to spit out on the front end of our site. So, , in this case, we're actually just kind of doing like an array map through the accordions and returning out an AccordionItem component for each of our accordions to kind of spit each one out on the, the content of the.
**Aurooba Ahmed:** Right. Totally. And so, yeah, that makes sense.
**Brian Coords:** And then inside of each accordion we're passing it. That object that we saw up above the accordion. Kind of like this guy right here, the id, the heading the content. So we're passing that as a prop. We're passing what the active ID is, so like which accordion is active? , we're passing this little conditional statement right here.
Uh, can you take a gander, like what do you think is happening in this statement right here?
**Aurooba Ahmed:** Okay, so it says the property is called isExpanded, which is something that we had before too. So it's gonna be probably tracking whether this current accordion should be open or not. If we are going with the same pattern we had before. And here we have a little conditional that says activeId is equal to accordion id.
So. Only expand this if the current accordion is selected to be open. Like if that the current accordion that is open is equal to the ID of this current item that we're looping over.
**Brian Coords:** Mm-hmm. Yep. And so this will return false for most of the accordions except for one. And you know, we'll kind of when we get into state, we'll deal with this. But essentially, anytime we change what the active accordion is, all of this gets re rendered. All of it gets like refreshed. And so this kind of happens automatically.
**Aurooba Ahmed:** Yeah, which is pretty cool if you think about it.
**Brian Coords:** Yeah, and the method for that is this function that we're passing right here called Expand, and we will save that as a juicy teaser for the next episode.
**Aurooba Ahmed:** Cool. I think though that's a nice clean little AccordionItem you've got there.
**Brian Coords:** Thank you. I'm, I'm pretty proud of it. All right. Should we actually open this AccordionItem and see what it looks like?
**Aurooba Ahmed:** Yeah. Let's see what you're doing with all those props that you're passing.
**Brian Coords:** Yeah, so we're going down the tree. This is the AccordionItem that we imported from that file and that we are using right here. So this is the file for that AccordionItem. And we can see here that we're importing some components here. So as we get down, we're kind of bringing in more components and we'll get to those as we get further down.
But, um, why don't you break? Like walk us through this piece right here. The accordion item is really a function and it's being passed an object with some variables in it. Can you kinda, how do you like to explain this?
**Aurooba Ahmed:** Okay. So. You actually just pass one, I guess in a way you could call it one, variable or one object to the component. But one of the great things about that you can do in React is the concept of destructuring. So here, this is kind of an implicit destructure where we're saying, Hey, we know what props are getting passed down, and these are the ones that we already know we want to pull out.
So instead, Adding one, uh, variable there beside in the function line saying props, we actually open up that object and say, here are the things that I want to create individual variables for. So in this one, in fact, you know, you might have had lots of other props also getting passed, but you don't necessarily wanna break all of them out.
So you could have just picked a couple and restructured those in here, and then actually, uh, just pulled those out. But in this case, you're actually. No, that's true. You are not adding, you're not doing all of them here. So you have three.
**Brian Coords:** I was wondering if you would catch that.
**Aurooba Ahmed:** Yeah. So you actually hit four, right? Go back to app.
**Brian Coords:** Yeah. Active ID right here.
**Aurooba Ahmed:** Hmm. Yes. So you are passing accordion. Active ID, isExpanded, and expand. But in accordion item, your only Destructuring accordion, isExpanded, and expand.
**Brian Coords:** Yeah. Do you have a theory of why I did that?
**Aurooba Ahmed:** Uh, no.
**Brian Coords:** Yeah, there's, uh, there's no reason I, you know, I probably like, had a different logic for when I started. I mean, you know. This is like code in progress and I like to be, uh, you know, open here. But like, I, I think I had a different method and then I was like, oh, wait, why don't I just do this in the prop? And like, you know, so originally I was probably gonna do that logic, uh, inside of my component when I realized, oh, I can do that in the prop in, in one line and save
**Aurooba Ahmed:** Ah, so you didn't have that conditional before.
**Brian Coords:** Yeah, so I could actually get rid of this if I wanted to, and yeah.
**Aurooba Ahmed:** And it would still all be fine. Yeah.
**Brian Coords:** mm-hmm. And like on a side note, I was at Word Camp Phoenix and I watched, um, a really great presentation on PHP, like seven and eight, um, where he was like breaking down, um, If you haven't really dug into PHP since like the like five, early seven era, like what are all the cool things?
And like even PHP does a lot of this cool stuff with, um, like constructors and objects where you can start like writing in the names of the things that you want. They could pass it and you just don't have to care about like what order everything is in. And like, you know, when you write a function and you're like, what's the first thing I pass?
What's the second thing? And like, dealing with. I just like love this. Yeah. It's like that modern way of like, I just, I know the name. That's all I care about. I don't care what order I put my variables in, if I use the same name, you know?
**Aurooba Ahmed:** totally. Totally. I, I, I love that part, you know, and when I remember when I first started with React, I was just like, this is awesome. I can pass it whatever I need, and then just use the parts that I need. And just like you, you know, I would often err on the side of like, passing more props than less, and then seeing how, what I end up using just to like not be a, a, like a blocker for myself and then later go back and clean it up a little bit.
And so like I didn't actually end up using that. I didn't actually need that, or I could do this a little better, you know? So
**Brian Coords:** Yeah. I get your hint. I should probably clean this up. Let's do that. Boom.
And then we destructure a little bit more. I just, I knew when I looked at this, I was like, something is not right here.
**Aurooba Ahmed:** Yes. I'm happy that you fixed the spelling on Destructure right there.
**Brian Coords:** That's
**Aurooba Ahmed:** bothering me.
**Brian Coords:** I was looking at it for a while and I was like, man, that's such a weird word. Like, but it didn't possibly occur to me that like, man, maybe I spelled it wrong.
**Aurooba Ahmed:** I mean, it is still a really weird word when you really look at, but you know.
**Brian Coords:** Yeah. And it's a weird concept that like, you know, this accordion is the same thing that I had here. You know, and you know, what this really means is everywhere that I'm using these, like what I could write is accordion, right? I could write accordion dot heading to get that value and I could write according.id and it's just
**Aurooba Ahmed:** is very vanilla, JavaScript.
**Brian Coords:** yeah.
And. I don't know. In some ways a bit more readable in the sense that like I know where it came from, but like in when you break things into like little tiny components like this, it's so nice to just like make little variables that are just easy to write.
**Aurooba Ahmed:** Yeah, I mean, this is a 30-line file. You know, that's not a lot and it's really easy to absorb in a very quick pass. So in, in situations like this, it doesn't really matter. But, uh, I will agree with you that sometimes I've seen very, very long files, really, really big, complex, complicated components. And when they destructure too early, it does make it harder to follow.
So there is something to be said. When you destructure something that matters, depending on how complicated or long your file is, you know, in terms of readability.
**Brian Coords:** and I think a, another thing, like part of the reason that this file is only 30 lines is because I broke the header and the panel into separate components as well, right? So, These, this, you know, in the earlier version of this, like all of this was kind of all HTML in one big spot, but I've kind of separated the header and the panel into separate components to make this file smaller.
And I'm honestly, I'm like, I'm on the fence about whether I needed to do that or not. You know what I mean?
**Aurooba Ahmed:** Well, how long are the other ones?
**Brian Coords:** let's start with the panel cuz the panel is very simple. Like all the logic is really happening here. All we're passing it is the content and the id so we can make a few names on it. Um, yeah, so this is the whole accordion panel right here,
**Aurooba Ahmed:** It's 15 lines. Mm-hmm.
**Brian Coords:** but like, I mean, I could just put this in, in right here, you know,
**Aurooba Ahmed:** Yeah, you could, but you. I think that, you know, we've talked about this before, but you and I love small files. We love having our code and little itty bitty things because it's not that hard to click around. And like when we have really nice code editors, like Visual Studio Code, or even when you're looking honestly on GitHub, you could have a, you can have a split view where you might have multiple files open and so you can like read them together if needed.
And I just think that as apps and websites get more and more complicated, the value of these tiny little files gets even, like it's, it's even more valuable to have that. So I, I think it makes total sense that you did it and I would've done the same thing, so,
**Brian Coords:** Yeah, I was curious cuz I think like the whole, one of the best parts about components is like the like DRY mentality, the like don't repeat yourself. Right? So,
**Aurooba Ahmed:** Yeah.
**Brian Coords:** You know, theoretically if I'm writing this component, it's because I'm probably going to use it in multiple places. Like a button is a very like reusable, modular, dry component.
Um, you know, even like the user avatar with their name next to it, like that becomes a component that you can use in all sorts of different places. , I'm not a hundred percent sure I would like reuse this as another component, like reuse this component in any other context. So,
**Aurooba Ahmed:** I I think, I think if you were going to be thinking that way, you probably wouldn't have called it AccordionPanel. You probably would've called it, you know, like ContentPanel or something like that. That just happens to have a few extra, you know, accessibility features in it and. Then you would be able to use it maybe in other places as well, just to be able to spit out some content.
And I think the same thing goes for the header, like having a button that could have a relationship with some content. I mean, that could be an accordion, that could be a tabbed area. You know, that could be a navigation, it could be a dropdown. There's a lot of ways that you could actually do it. So if you were using that con idea in a larger, uh, project scope or context, You would probably, maybe not call it an accordion. But having those little pieces would make it more configurable, right?
Maybe you started with a accordion, realize it's basically the same code as you're trying to write for this tab thing and decide to refactor it so that you could use it in both cases, but that would be much harder to do if you hadn't broken it out into more files, right?
**Brian Coords:** Yeah, like tabs, modals, a lot of these sort of like dynamically showing and hiding content that need a lot of the same accessibility. They need a lot of the same like showing and hiding functionality and stuff. Um, yeah, I could see that.
**Aurooba Ahmed:** Totally.
**Brian Coords:** since you said accordion header, I opened the accordion header so we could take a look at it real quick.
**Aurooba Ahmed:** ,
Yeah.
**Brian Coords:** Essentially it's, it's really a lot of the same stuff, um, where we're passing some, some of the props, we're pulling them out. Um, there's really no logic. The only thing I would say that's a little bit fancy here is that we do have an onClick function for our button. Um, and, and that kind of is gonna get passed back up.
You know, when we call this function, it's kind of gonna go back up the tree all the way up to that App.Js, which we'll look at next, next.
**Aurooba Ahmed:** Yes. Yeah, and I mean, we have always had an onClick right from the beginning of this series, is just that the way we're, what the behavior is that we're doing is changing now that we have a relationship between multiple accordion items.
**Brian Coords:** And if I were to look at some of this, there's, I definitely, you know, I went through a few different versions of it where I, you know... It's kind of like, part of it's like, what do I want to be able to show in, like in this conversation, what do I want to be able to keep as simple? Like there's some things where, you know, a lot of these, um, Attributes and stuff could actually be, be defined a lot higher up and we could just parse out those attributes.
These like one idea, like there's a lot of other ways to make it more DRY and like reusable. Um, and put a lot of this logic back up into the accordion item. Um, which is really like the kind of core component here.
I.
**Aurooba Ahmed:** Yeah. Yeah. I mean, right now the AccordionItem itself is in charge of showing and hiding the panel, and aside from that, just passing some props down to other components. So I could definitely see a value there for maybe keeping a lot more of the logic there than passing it down. Because the other thing with the React is you know, you never wanna do any super deep prop drilling, which is something that we'll get into at some point, probably.
Um, but, and it's really easy to, and you're find yourself in a situation where you're doing that.
**Brian Coords:** Yeah, and I think these are a lot of the places where when you're new to React, you sort of like. You get in your head a little bit or you get bogged down because there's so many ways to kind of do this where you're like, well, I could define it here. I could define it there. I could pass it now.
I could pass it later. And you know, a lot of those things end up just being, you know, 1) based on the future of how scalable you want this to be. , and then also there's a component that's just like personal preference in your style. You know, everyone has their style. But scalability, what you're gonna do with it, where you're planning to grow with it.
, that's probably, I would say, maybe the most important like thing to think about when you start planning out where props go, where things happen, where logic is. What do you think?
**Aurooba Ahmed:** Yeah. Yeah, I think so. I also think that there is no harm in doing prop drilling or anything else that is not quite scalable when you're first sort of prototyping a component or prototyping a piece of functionality. Sometimes you're not- no matter how much you might think about it, really hard, sometimes you won't know exactly where you wanna, where it makes sense to piece, put a piece of logic or where to set up like a context or something like that until you've played around with it, tried it all out.
And then you can do a little bit of cleanup, which goes for like all the code in the world, all projects, anything that you might wanna do, right? So yeah, there's no harm in it as long as you are cognizant that you might wanna come back and clean it up a little bit? I think always the first goal should be, let's just make it work first.
You know, let's not get too in our heads about what is the perfect thing to do from the get-go, because you're not, you don't know until you've done it.
**Brian Coords:** Yeah, and so to kind of like bring it full circle, coming back to, you know, this piece right here of , the data that's gonna come from the front end is our starting point. Cuz we know what an accordion needs to be. We know it needs to be a few rows of heading and content. And this was like the first piece of our
you know, data. And so it was really cool to see like it kind of trickle down through each component and how you can just pass things and, and set it up. do you wanna give us, cuz you know, we definitely glossed over state and our expand function and stuff, so do you wanna wrap us up with like a preview of, of, of what we should think about for our next
**Aurooba Ahmed:** episode?
I think what we have here, the parts that we wanna cover in the next episode of this series will be the way React actually works under the hood in, in terms of how it re-renders and when it renders things on the front end. Like we're taking a advantage of that quirk of React here to make things a lot simpler for ourselves.
And of course we're, you know, we've got a complicated little ternary statement here that we'll walk through, on how we change what a accordion item isOpen and being able to close the other ones. So I think that we're gonna have a lot to dig into and really talk a little more higher concept about React in our next episode and look at, you know, maybe a few other ways that we could also approach this depending on what our ultimate goals were for this component.
**Brian Coords:** Yeah, cuz I think the way that you click a button down here and it travels and every other piece has to "react" to it, is like the core of React. So, uh, I'm looking forward to it because I think there's, there's, like you said before, there's so many ways to do things. So it's always interesting to figure out and learn like slowly, like which ways pay off for which situations.
**Aurooba Ahmed:** Yeah. I'm excited for that conversation. I think it'll be a good one.
**Brian Coords:** Okay, then I will see you.
**Aurooba Ahmed:** See you next time.
**Brian Coords:** Visit viewsource.fm for the latest updates and links to the show notes. Review and subscribe to viewSource in iTunes, YouTube, or wherever you get your podcasts.
Creators and Guests
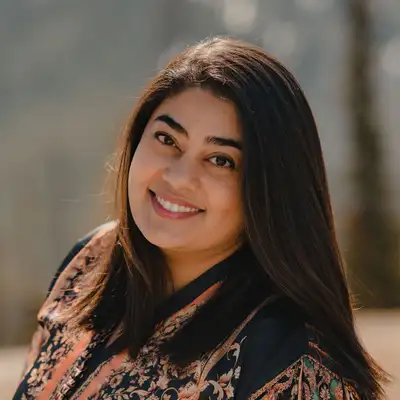
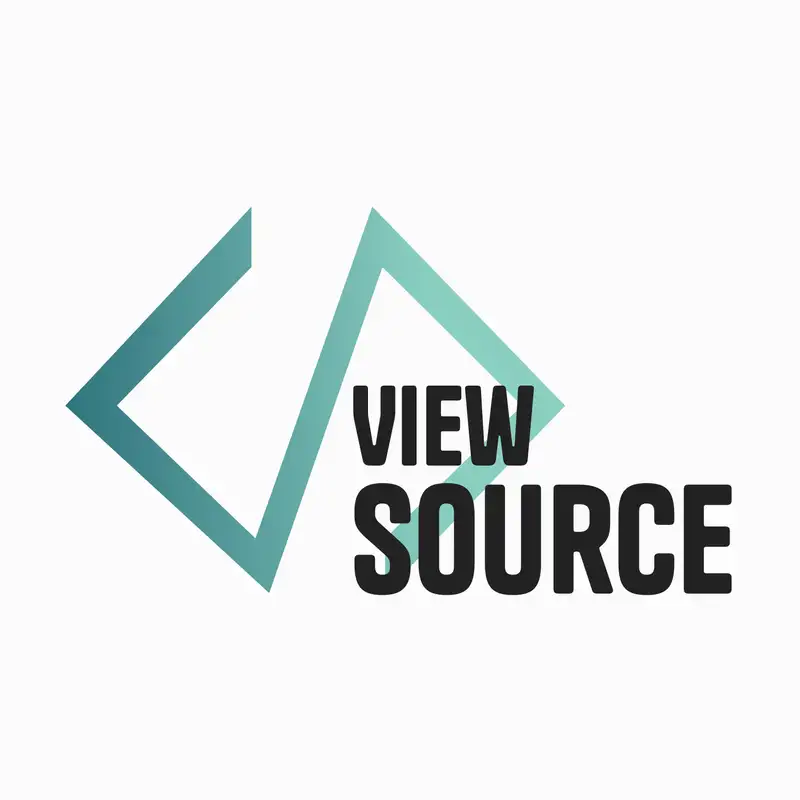