Managing State in React
## [00:00:00] Introduction
[00:00:00] **Aurooba Ahmed:** You are listening to viewSource a conversation around tech, web development, and WordPress with hosts Aurooba Ahmed That's me and Brian Coords
[00:00:10] Hello. How are you doing today, Brian?
[00:00:13] **Brian Coords:** I'm doing fantastic, as I always say when you ask me that opening question every time.
[00:00:20] **Aurooba Ahmed:** Every time. It's always the same. Well, I'm doing pretty okay myself.
## [00:00:25] Recap of where we are in the React series
[00:00:25] **Aurooba Ahmed:** Today we are gonna dive right back into our React series and it's part five, I guess. Yeah, it's part five. And you know, we've been on this journey where we started with a bit of React on our WordPress website and then we started building an accordion and eventually we're gonna turn that into an accordion block in the block editor.
[00:00:46] Um, but we're not quite there yet. The last time we were here, Brian had finished turning our little accordion component into a series of mini components. That, do different parts of the accordion and we walk through a part of that, right?
[00:01:04] **Brian Coords:** Yeah, we, we looked at, you know, you have an accordion, you have the panel, you have the header, you have all these little separate pieces. How can I break them apart? How can I share data between them? You know, we, we stopped right at, right at the like, edge of like, how do I share interactivity and events between them?
[00:01:23] But, but, uh, that's what we're, we're here to, to get into today.
[00:01:27] **Aurooba Ahmed:** Yeah, so, you know, how does React's interactivity actually work? What is the core piece that you sort of find yourself working with when you, uh, want something to react to something else that's you're doing as a user? That's what we're gonna dig into today. So let me go ahead and share my screen.
[00:01:46] **Brian Coords:** I'm super excited to see what you've done, I feel like you've gotta have changed my code a little bit. Um, you know, it's kind of like a, it's a real pair programming cuz we each take turns kind of like chipping away at the code. And, uh, so I'm excited to see what's happened since the last episode.
## [00:02:03] Rerenders in React
[00:02:03] **Aurooba Ahmed:** Yeah, so I'm sharing my screen here and right now we're seeing that com, that sort of proper accordion that Brian built, which is more than just one panel. So there's a first Hello world, and I can open that up and see the Lorem Ipsum, and then there's another one, and I can open that up. If I were to open another one without closing the first one, the current one that was open will close when you open the new one, right? So they sort of have a relationship there. They toggle, on and off based on the interaction that you have with other, other pieces. So that's the part that Brian built and what we wanna talk about now is what happens that makes that interactivity happen without the page refreshing.
[00:02:52] So if you were looking at this, Brian, what would be your guess about how this works?
[00:02:58] **Brian Coords:** So, you know, I have a good high level understanding of React, and I have experience in it, but I would nev, like if I had to actually look at inside of React itself, it would definitely be like way over my head. Like, what is it doing? You know what I mean? Like, I just know how to make it, do the things I want, you know, versus say WordPress, where you're like more, I understand from like line one, you know what's happening.
[00:03:23] So from my understanding of React is like essentially every time you make a change, it's sort of like, removing and repainting really everything in your component. Like, so your component
[00:03:35] **Aurooba Ahmed:** yes
[00:03:35] **Brian Coords:** kind of like almost instantly like disappears and is repainted fresh. It's like a, you know, it's, it's rendered fresh every time there's a change, which is why a change over here can impact over there, because it's just gonna say like, I'm watching all the changes and I'm just gonna paint the whole thing fresh every time you do a little change.
[00:03:51] Do I have that right?
[00:03:52] **Aurooba Ahmed:** That's right. Yeah, I mean there are situations where it may not be painting the entire thing from like, from fresh, from get-go, but yes, essentially every single time you interact with a piece of, uh, React, a part of a React application, it's, we've talked about the virtual DOM in our very, very first episode of this series.
[00:04:12] You know, that's where it's gonna compare the virtual DOM based and the current actual what's on the what's in the browser and then based on that it will swap out and make those changes to make it look interactive and react. So I actually made a little bit of change to the code, like you guessed, uh, to just sort of show and sort of demonstrate, you know, when you do react or change something in here, the app is in fact rerendering. So I'm, I've opened up the console and the very first thing you're gonna see in this console, aside from this jQuery migrate. Uh,
[00:04:49] **Brian Coords:** classic
[00:04:49] **Aurooba Ahmed:** thing that's always there, yeah. Is it says rerendered accordion component zero times.
[00:04:55] So I had just finished refreshing the page. It has just been zero really means one, like it's just been rererendered once. But now if I open
[00:05:04] **Brian Coords:** It's been rerendered zero times cuz it's been rendered once.
[00:05:08] **Aurooba Ahmed:** Yes,
[00:05:09] **Brian Coords:** so you, you were not wrong.
[00:05:12] **Aurooba Ahmed:** that's true. That's true. Now if I click on the very first accordion component to accordion header to make it open, it opened. And now we see a new message in the console and it says rerendered accordion component one time. Or it well says one times, but you know, and if I close it now, you can see it's saying it's rerendered it a second time.
[00:05:36] So every single time I am interacting with this app, with this little react application and something is changing in the DOM, it's rerendering every single thing. So it's not just hiding and showing this one component, everything, all the functions, all of the stuff involved in this app is being run again.
[00:05:56] **Brian Coords:** Yeah, Yeah and I think we've talked before about the idea that, you know, right now we are showing we're not just showing and hiding things with the accordion. We're like actually removing it. Like when, when it renders it right here. It's literally not rendering anything in between those buttons. It's only rendering the little Lorem Ipsum panels, um, when actually visible.
[00:06:18] So, um, that's
[00:06:19] **Aurooba Ahmed:** Exactly, so if I were to go.
[00:06:22] **Brian Coords:** Yeah,
[00:06:23] **Aurooba Ahmed:** If I go and look in the code, literally right now, all you see is these three H2, uh, sort of wrapper buttons. And then if I were to open one, you can see automagically underneath that first H2, we have that actual panel that showed up.
## [00:06:39] What is state in React?
[00:06:39] **Brian Coords:** Nice. So, so we've seen it re-render. We've seen it do this. What, I mean, what's the, like, how do, how does it know? Like what, what's the process for it to say like, How he, like, what is it, what tells React, okay, something change, repaint. Like, what is that called? Like what? What do you, what do you call?
[00:07:01] **Aurooba Ahmed:** so this is where the concept of state really comes in, right? We're managing the status, the state of some part of this application. There's something that we are keeping track of and then updating when, uh, an interaction happens and really state management is that core. Mm. Part of React that makes React what React is. It's why Facebook invented it to be able to manage those reactions. Like, you know, you talked about it in our very first episode too, that, you know, when you click like somewhere or have a notification that shows up here, it shows up in another part of the application as well because you're managing the, that that is like sort of a global variable that all the different parts of the application are aware of.
[00:07:50] So in in React, we actually. a hook called useState and you, you have used useState to create, um, an activeID that we talked about last time for managing what accordion component is open. So do you wanna walk us through what activeID does and how you change it?
[00:08:16] **Brian Coords:** Yeah, sure. So, and like I also think of state like, as like short-term memory in the sense that it's memory. It exists when you load your page and it's kind of remembering things in the moment. Um, it's not like long-term memory, which would maybe be a database and some APIs and stuff, and it's sometimes you change data and that's like your short term memory being updated and stuff.
[00:08:40] And then, you know, further down the line, we'll probably get into the process of taking that and pushing it deep into your long-term memory so that it's, you know, you're remembering things for the future, but state is just like, what, what's happening right now. So,
[00:08:54] **Aurooba Ahmed:** Right.
[00:08:56] **Brian Coords:** so when we, um, When we like use state, we call the useState function and we basically grab two things out of it.
[00:09:02] We, so on line 32 you can see like I'm defining two constants. One is the actual value that I wanna store. We're calling it activeID. Cause we're saying like which ID of our accordion is active. Is it the one with the zero? Is it the first one? Is it number two? Like what is the ID number of the one that's currently active and open.
[00:09:22] So that's the first variable we're getting. And then we're getting a second variable, which is really a function called setActiveId. And that's our way to update it. Like we don't just change activeID, we don't just change the value of the variable. That's we just, uh, no, let's like, we'd be in big trouble if we did that.
[00:09:39] We tell our state with that function. Please update this for me. Uh, and State does a good job of like not screwing that up for us. So that's what line 32 is. It's like what am I, what's the value and gimme a little function I can use when I want to change that value.
[00:09:56] **Aurooba Ahmed:** Right, and because this is all set up as a constant, these are set up as constant variables, essentially functions. That's why you can't really go ahead and change activeID. It's meant to be affected by something else and not changed directly on its own. So that is the state you have set up here. And then, uh, you have this function called expand.
[00:10:19] And it's, that's the part that is actually changing, what is put into activeID. And here is where we have the thing that I really like and the thing that you really don't like, which is a ternary function. you wanna walk us through why or at least what this is doing first?
## [00:10:40] The expand() function
[00:10:40] **Brian Coords:** Yeah, let's talk about, so expand is basically, it's a function that's like when I click on a button, call this It's like, it's our classic like onClick event for clicking the buttons. Um, it's something you would do in any sort of JavaScripts, like even if you were building your jQuery accordion or something, you'd have, you know, when I hit click, run this function.
[00:10:59] That's what the expand function is, right? And what the function needs to do is basically say, Whatever button I clicked, that's the accordion that I want expanded. And I also want you to go ahead and close all the other accordions, right? Like, make sure the other ones are not still open.
[00:11:15] **Aurooba Ahmed:** Right.
[00:11:15] **Brian Coords:** So when I wrote it, I wrote it three different ways because I thought there would be, uh, kind of fun to see three different examples.
[00:11:23] So example one is like just set the activeID to the accordion that I, that I.
[00:11:30] **Aurooba Ahmed:** Clicked on
[00:11:31] **Brian Coords:** That I clicked on. So is this, can you save it and run it or no?
[00:11:35] **Aurooba Ahmed:** Yes, I can. So I saved it, and when I click on the first one, it opens.
[00:11:44] **Brian Coords:** And you click on the second one and the second opens. Cuz I'm setting the activeID two. But what happens if I click on the same one twice?
[00:11:52] **Aurooba Ahmed:** Nothing.
[00:11:52] **Brian Coords:** I can't close it because all I did was I set it to just set the activeID, but I didn't say, unset the activeID, if I click on the button again, like
[00:12:02] **Aurooba Ahmed:** You didn't toggle it.
[00:12:03] **Brian Coords:** it's not a toggle. Yeah, you can, it clicks to expand, but it never says, oh, I clicked on the same one, unexpand.
[00:12:10] **Aurooba Ahmed:** Yes, exactly.
[00:12:12] **Brian Coords:** So, so go back to the code. So I wrote it that way first, and then I very quickly saw that it was not a good way to write it. Um, so example one, then we have example two, which is the one we've had active. But let's skip over it first, and let's go to example three, because example three is the same thing.
[00:12:31] It's just the, the very verbose version of it. It's, um, we, we break down all the logic into separate lines so we can make sure very clear what's happening here.
[00:12:40] **Aurooba Ahmed:** It's a proper if statement WordPress style..
[00:12:44] **Brian Coords:** Yeah. If, um, I think we probably talked about this before, but I know I'm very, like, I'm coming around, but I'm very partial to long-winded code that's like easier to read. Um, you know, you, you do, you do grow into the, the ternary functions, but I really, uh, I love this. It's pretty easy to read.
[00:13:04] **Aurooba Ahmed:** And we've looked at, yeah, and we've looked at how when ternary functions actually don't make sense. Like in our debugging, uh, debugging episode, we had a ternary expression, and it just made no sense to use it because we were looking at more than two things, right? So it's really only appropriate in certain situations.
[00:13:21] **Brian Coords:** Yeah, it's really great for like binary choices. Like either I want this or I want that. Once you get more like different things and, and the logic is more complicated, uh, it can get too hard. Like if you see, if you start seeing multiple ternaries like. Changing something and then something else changes that, and then something cha like, then you're, you're probably on the wrong path.
[00:13:41] **Aurooba Ahmed:** Yeah, okay.
[00:13:43] **Brian Coords:** So what we're doing here, you clicked expand button. What we're doing is we're checking what is the current activeID that's already existing, the, the variable, and is it, is it the one that's, that we clicked on right now? And if it is then just null out the activeID so that there's no active and that's gonna basically collapse all the accordions give us that, that collapse and then if not at make the one we clicked on active.
[00:14:09] **Aurooba Ahmed:** right? So now if I go back to my actual window here and I refresh, I can click on the very first accordion item and then I can click on the second one and that closes the first one and open the second one. And if I click on the second one again, it will in fact close that one because by doing that, we actually nulled out that activeID.
## [00:14:30] How a ternary expression works
[00:14:30] **Brian Coords:** Yeah. So. And also, you know, we had to use null because we couldn't just say like zero. Cuz obviously our first accordion is indexed to zero, just, uh, is kinda a standard thing.
[00:14:43] **Aurooba Ahmed:** Mm-hmm. Yep. We have that set up in our accordion array at the top here, which I'm showing on screen right now.
[00:14:49] **Brian Coords:** So we can look at example two now, which is the, the kind of like very, like it's all of that in one line of code, which is. We're actually doing all like cuz setActiveId is our function that basically we have to pass setActiveId either one of the ID numbers or a null. Yeah. We gotta pass some value, and here we have an entire like if statement all in one line in there. And it's that if statement's gonna just return basically the correct value, it's gonna return null or it's gonna return the accordion ID.
[00:15:24] **Aurooba Ahmed:** Right. So we have the first part of the expression, or sorry, we have the first part of the statement. That is an expression. That's what you would've put inside the brackets if you had an if there. So here it says activeID. And is equal to with my ligature three equal signs right there and is equal to accordion.id.
[00:15:42] Then we have a question mark, so anything before the question mark that's the, that's the expression that we're evaluating. Then the first value after that question mark is what would happen if that expression we were checking is true? If it's true in this case. So if activeID is the same as the current accordion ID that we've clicked on, we want to set this value for setActiveId to null, then we have a colon and then whatever we wanna happen, if that expression was false. So if the current activeID is not the same as the one we've clicked on, then we wanna set it to be that current one we clicked on and opened that one up. So it's all happening in one.
[00:16:23] And you know, we've seen that this happens. This is far more common in JavaScript than PHP.
[00:16:30] **Brian Coords:** Yeah. Why do you have I have a theory. Do you have a theory? Why? Because even the WordPress coding standards, they're pretty strict, in the php, like they really don't like, you know, they really want your code to be so readable for beginners and stuff. And I think we've all experienced the fact that in the JavaScript side of WordPress, a lot of that expectation of the commenting the code and making code really verbose, like really is not quite as strict as it was in like the PHP side.
## [00:16:55] Why are ternary expressions more common in JavaScript?
[00:16:55] **Brian Coords:** Um, and it's just a, it's just a different way of thinking. Why do you think these, sort of like ternary expressions are so prevalent in JavaScript?
[00:17:04] **Aurooba Ahmed:** So I can't speak to why the codes, why the standards are like more relaxed. My theory there would be that it's because it all started to get really fast when Gutenberg development was happening, and having to, being able to move that fast was not gonna work as well with having to be super, super verbose about every single thing, if it's breaking and changing constantly.
[00:17:28] But for the ternary statements, you know, when you write something like a variable, like a var, you know, variable equals to two plus two. The stuff be, that is in, in entirety, it's a statement. It's a, it's a statement, whether you're in PHP or in JavaScript. That would be considered a statement. But the stuff that is on the right side of that equal sign, which is what we're setting the variable to that value, that's called an expression.
[00:17:59] So an expression, in React, especially in JSX, you know, you can't have a statement sort of like an IF statement everywhere, but you can have an expression in a lot more places. So for example, if I wanted to ha, if I wanted to return something conditionally in JSX, I can't write a statement there because it's all supposed to be rendered into HTML and there is no HTML for an IF statement.
[00:18:28] You can pass an expression and say, Hey, um, if a certain value is true, if a certain value is real, then put this HTML on the page. That's why like ternaries are more common because as an expression, it's legal and valid to include when you're working with HTML or something that needs to get passed to the DOM.
[00:18:52] But then if statement, that's pure programming concept of a pure logic that can't, that can't, uh, live in the area that renders HTML, which is why ternaries became so common. Does that make sense?
[00:19:06] **Brian Coords:** And I, I think, well that's one thing is that's one reason I think a lot of people really like Vue and that more like declarative style where you can have like your v-if your v-else and you know, I understand. It's like if you're just starting the JavaScript framework path, Like something like Vue feels a lot like friendlier cuz you can do a lot of that sort of stuff.
[00:19:26] And if you've came from the WordPress world of like template tags and building out everything in your PHP, then like Vue feels a lot closer to that. Um, I think there's another piece where, like the PHP world, like the way PHP runs is it is very linear. Like it's like, What goes on this line? Then what happens on this line?
[00:19:44] And then what happens on this line And everything is very, like, if I put something here and then I put something here, I know what's gonna happen. But JavaScript a lot of times doesn't really work that way. And like a lot of times you really want to be as quick as possible through everything. So that stuff can kind of like execute as effectively, um, as possible.
[00:20:03] **Aurooba Ahmed:** Yeah, totally. I think that whenever we think of asynchronous anything, we automatically first think of JavaScript, right? And when you have things that are happening asynchronously, You don't know when what's gonna evaluate. So if you need something to work you, it's, it's safer to put it all in one line than to have it over multiple lines or in multiple places because you don't know which part is gonna end up happening first.
[00:20:29] Right? Yeah.
## [00:20:33] Where it makes sense to use a ternary experession
[00:20:33] **Brian Coords:** So one example of what you were talking about where you can and can't be as conditional is in the accordion item. So this is the accordion item component, but like if you actually go into that component, we don't want to show the content unless the, unless it's actually set to be expanded. And so that's like one example of where you put that kind of like, You're, you're sort of tr you're trying to use a conditional, but really it's an expression and like that, that right there like took a minute for me to wrap my head around.
[00:21:07] **Aurooba Ahmed:** Yeah, and you know, you wouldn't have, you couldn't, you don't necessarily have to write it this way, right? You could in fact have done an entire if statement and then had a return that either returns the accordion header and the panel, or it just returns the accordion header, right? So that would've been the if statement version of doing that.
[00:21:26] But the downside of that is now you have two return statements to pay attention to and look at what is happening. Whereas in this case, you know that there's just one return. You look down and you into this function, see what's going on, and then see which part you need to pick out and like sort of read and evaluate or debug or whatever.
[00:21:46] You know, you have one source of truth to deal with. Instead of saying, oh, if this is true, then I gotta look at that return statement. But if this is true, then I gotta look at that return statement. And maybe you made a mistake in one and didn't make it in another, and they're very similar and it's hard to spot.
[00:22:01] I mean, that happens all the time.
[00:22:03] **Brian Coords:** Yeah, is it's definitely like you want that DRY code, don't repeat yourself. You want everything broken down. Um, one other question. Our accordion button that we're clicking is all the way in that header component, right? Like that's where the onClick is. But the logic of the function that I run when I click it is way up in the app.js right?
[00:22:28] So how does it work that my accordion header component, which is like two components down this little tree can actually trigger. Because I think that's a lot of, you know, a lot of times the, the thing about state is you kind of want state at the highest point, you know, and you want it to like trickle down.
[00:22:44] So how am I clicking something way down here and sending that, that event all the way up here?
[00:22:51] **Aurooba Ahmed:** First of all, whenever, because like you said, you wanna have stayed at the highest possible level that you would want access to it. So in this case, you know, we want activeID in this very highest level because we wanna be able to control all the different accordion items from here if needed. And which we do.
[00:23:11] But then here in the your return statement, you actually pass not only the state, the the, the activeID state, but you actually also pass the function that hel, that controls that, um, that changes the actual activeID as well. Right. Based on the evaluation. So because you've passed that down as props, the app, the React knows to pay attention to that part of the app as well in order to deal with that state.
[00:23:39] If you hadn't passed it down, then whatever changes you made there would be localized and wouldn't cause a rerender of the whole app. So there are also situations. That you could have a React application where making a change of some sort of state doesn't actually render the entire application again.
[00:23:57] For example, in the block editor, each little block is kind of like a little react application of its own sitting inside this larger React application, right? So you can affect and re-render your in your block without causing it to reinder everything else around it. You can. Yeah. So it, it knows to pay attention to state wherever you passed it that state.
[00:24:20] Does that make sense?
[00:24:21] **Brian Coords:** Yeah, so in my accordion header, there's a, you know, there's literally the onClick event of my button, right? Like that's, there's just a standard button and it's hitting expand. And if we follow that back up, that expand variable is just a prop that we passed, but it's a prop that we passed that that's essentially sending that function down.
[00:24:43] We're saying like, this is just a function. You can now use this function that you defined here. You can call it anywhere else that you've passed it down. Uh, your props don't have to just be val values. They can be functions as well.
[00:24:57] **Aurooba Ahmed:** exactly. You can pass down anything, literally anything in there that could be stored in some sort of variable or given a name in, uh, you could even technically pass it an anonymous function as well, but once you pass it down, it'll actually be associated with the name because you have to give it a name in order to pass it as a prop, as a property of that item.
[00:25:20] Yeah, so. There are other ways that you can also pass down or, or share, share knowledge of state or any kind of information between different parts of, a React application. One of them is this thing, this funky thing called context, but I don't think we're quite there yet where we can explore that. What do you think?
[00:25:43] **Brian Coords:** Yeah, I, you know that. I think that's a good question of where we should take this conversation next because there's so many, you know, I think that we both, we wanna end up with an accordion block, I think has gotta be the final thing. But like, it makes so much more sense to like, learn all of these pieces before you get into like WordPress React and Block Editor React, which is very different.
## [00:26:09] What's next?
[00:26:09] **Brian Coords:** So it, it makes me wonder, what, what could we see as, Give us a preview. What do you think? What are you thinking should be the next thing that needs to happen with this accordion?
[00:26:21] **Aurooba Ahmed:** I honestly think that it is time for us to go into the block editor. No?
[00:26:27] **Brian Coords:** Yeah. No, I, I think you're probably right. Like it functions, it's accessible. It does all the stuff we need it to do. So now I should be able to actually like populate the content in the backend of the WordPress.
[00:26:41] **Aurooba Ahmed:** Yeah. And I think that when we do that, we have to start it out that same way, where we just do a single accordion item first. What does that look like? And then what are our options for creating a proper accordion? So, which is, you know, a set of accordion items, not just one. What does that experience look like and what kind of react does it take?
[00:27:02] When those things have to be dynamically populated and can't just be a little array of values and IDs that we hardcoded into the application.
[00:27:13] **Brian Coords:** And as anybody who's done any block editor based sites has already done this, like you've
[00:27:19] **Aurooba Ahmed:** Yeah.
[00:27:19] **Brian Coords:** you know, you've built an accordion and you're just thinking, all right there, there's a lot of decisions that need to be made to get to get to that point.
## [00:27:28] Taking the editing experience into account when making a block
[00:27:28] **Aurooba Ahmed:** And like eight different ways that you could achieve it, depending on how you want it to, or what kind of experience editing experience you wanted to create. Right? Because once you get into the block editor, you're not just thinking about, oh, what is the code required to accomplish this task? It's also what is the code required to make this an intuitive or user-friendly task? Right?
[00:27:53] **Brian Coords:** Yeah. I mean, I think that's what a lot of people get tripped up on. You know, it's not just the final markup of the actual accordion. And it's not just the functionality of the actual expanding thing, it's the user experience of adding that content. And how do I add another accordion to my, you know, how do I add another panel?
[00:28:13] How do I put content in here? How do I edit it? How do I, you know, all of that stuff. Like you, you do have to kind of build that out and there's tools to do it, but, uh, it definitely requires, um, some, some thought and planning.
[00:28:27] **Aurooba Ahmed:** Yes. Yeah. And like, how can I work with the block editor's existing language and way of expressing itself to the, the user instead of just, you know, oh yeah, I thought I should do it this way, and then I did it, and now five different people have made five different blocks in five different ways, and you have sort of a jarring experience that is a lot like the notification chaos of the current WordPress dashboard.
[00:28:52] **Brian Coords:** Yeah, I mean. I do think compared to the difference between using two different page builders, we are getting to a place where that backend user interface is a little bit more consistent. But yes, you people, I think would be surprised to see how much actually freedom you have when designing the editing interface of your block.
[00:29:13] And, um, you really do want to give something that feels consistent and cohesive and still easy to understand. So there'll be a lot to unpack there.
[00:29:23] **Aurooba Ahmed:** Definitely. So yeah, I think that in our next episode of this series, when we get there is to look at what a initial accordion item looks like as an editable experience in the block editor.
[00:29:38] **Brian Coords:** All right. I definitely, I mean, I have some ideas cuz
[00:29:42] **Aurooba Ahmed:** So do I.
[00:29:43] **Brian Coords:** been been doing this a few times, so I'm looking forward to this.
[00:29:47] **Aurooba Ahmed:** me too. So I'll see you in the next episode then.
[00:29:50] **Brian Coords:** All right, see you then.
[00:29:51] Visit viewsource.fm for the latest updates and links to the show notes. Review and subscribe to viewSource in iTunes, YouTube, or wherever you get your podcasts.
Creators and Guests
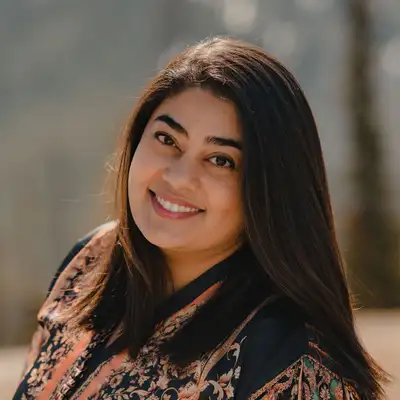
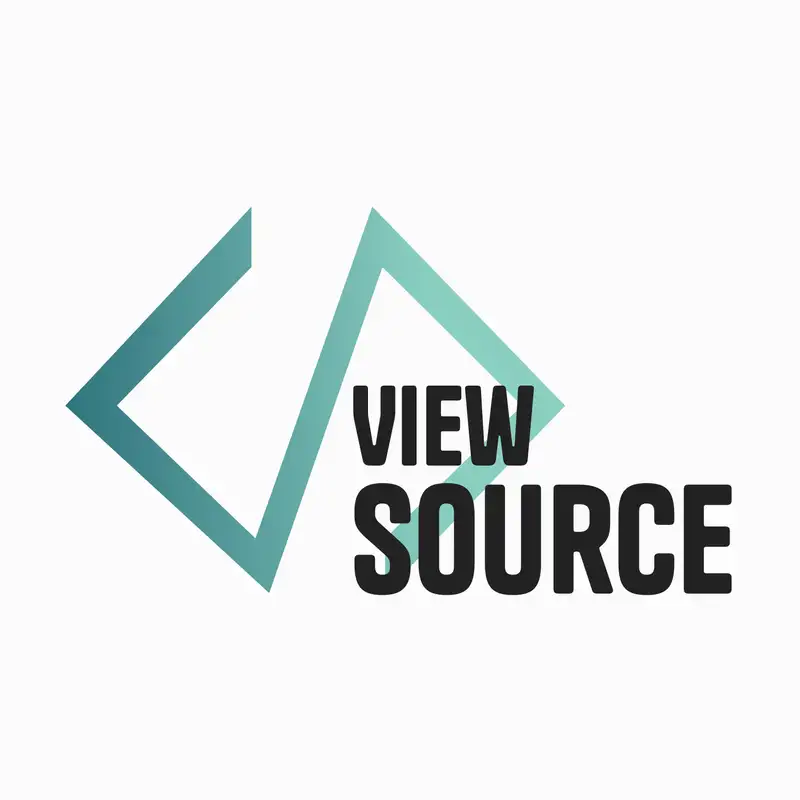